Data analysis is a powerful skill that opens doors to countless opportunities in a data-driven world. Python, one of the most versatile programming languages, has become a go-to tool for data scientists and analysts due to its simplicity and wide range of libraries. Its rich ecosystem of libraries and tools makes it ideal for manipulating, visualizing, and deriving insights from data. This comprehensive guide covers everything from Python data analysis to handling big data and performing advanced tasks like text data analysis, Statistical Analysis with Python, and time series forecasting.
Python Libraries for Data Analysis
Python owes much of its popularity to its vast array of libraries designed specifically for data analysis. These libraries simplify the handling of datasets, statistical computations, and visualization tasks. Python libraries like NumPy, Pandas, and Matplotlib form the backbone of data analysis workflows. They cater to various needs, such as numerical computation, data manipulation, and visualization.
NumPy for Numerical Data
NumPy (Numerical Python) is a foundational library for handling numerical data efficiently. It is widely used for performing mathematical operations, handling large datasets, and working with multi-dimensional arrays. Its powerful capabilities make it an essential tool for numerical computations and data analysis in Python.
Creating Arrays with NumPy
NumPy arrays are faster and more memory-efficient than traditional Python lists, making them ideal for processing large amounts of data. These arrays support various operations, such as element-wise arithmetic and broadcasting. Here’s how to create and manipulate arrays:
import numpy as np
# Creating a 1D array
array_1d = np.array([1, 2, 3, 4])
# Creating a 2D array
array_2d = np.array([[1, 2], [3, 4]])
# Basic operations
print(array_1d * 2) # Multiply each element by 2
NumPy also provides robust mathematical functions, such as trigonometric operations, matrix manipulation, and statistical calculations, making it a go-to library for numerical computations.
Pandas for Data Manipulation
Pandas simplifies working with structured data like tables, providing tools for efficient data analysis and manipulation. Its intuitive interface makes it easy to handle datasets of any size.
DataFrames and Series
- Series: A one-dimensional labeled array capable of holding data of any type, such as integers, strings, or floats.
- DataFrame: A two-dimensional labeled data structure, akin to a spreadsheet or SQL table, allowing multi-dimensional data management.
Creating a DataFrame
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]}
df = pd.DataFrame(data)
print(df)
This example creates a DataFrame from a dictionary, representing structured tabular data in rows and columns.
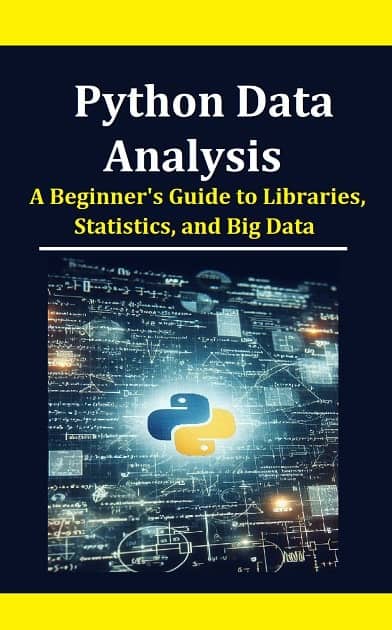
Statistical Analysis with Python
Statistics is vital for making data-driven decisions. It allows us to derive meaningful insights, test assumptions, and validate models, which is crucial for real-world applications in fields like finance, healthcare, and marketing.
Hypothesis Testing
Hypothesis testing is a statistical method used to determine if an observed effect or difference in data is statistically significant or due to random chance. It helps in validating assumptions in experiments.
from scipy.stats import ttest_ind
t_stat, p_value = ttest_ind([10, 20, 30], [15, 25, 35])
print(f"P-value: {p_value}")
Regression Analysis
Regression analysis explores relationships between dependent and independent variables. It predicts outcomes and identifies trends, making it a cornerstone of predictive analytics.
from sklearn.linear_model import LinearRegression
model = LinearRegression().fit([[1], [2], [3]], [4, 6, 8])
print(model.coef_)
Machine Learning Basics
Python simplifies machine learning with libraries like Scikit-learn, making it easy to implement machine learning models without having to delve deep into the complexities of algorithmic theory. Scikit-learn provides tools for preprocessing, model training, validation, and evaluation. It supports a variety of algorithms, such as classification, regression, clustering, and dimensionality reduction, making it versatile for different types of machine learning tasks.
Supervised vs. Unsupervised Learning
- Supervised Learning: Models are trained on labeled data, meaning the algorithm learns from the input-output pairs, such as using past sales data to predict future sales.
- Unsupervised Learning: Involves finding patterns in unlabeled data, where the algorithm seeks to discover inherent structures, such as grouping customers by behavior without predefined categories.
Basic Algorithms
- Linear Regression: A simple approach for predicting a target variable by fitting a linear relationship between independent and dependent variables.
- K-Nearest Neighbors (KNN): A classification algorithm that assigns a label to a data point based on the majority class of its nearest neighbors.
- K-Means Clustering: An unsupervised learning algorithm used to divide data into clusters based on similarity.
Handling Big Data with Python
Big data analysis often involves datasets too large for traditional tools, requiring more robust solutions for processing and analyzing massive amounts of information. Python provides the necessary libraries to handle such tasks, ensuring you can work efficiently with datasets that go beyond the capability of standard data manipulation tools.
Working with Large Datasets
Using PySpark, you can read and analyze large CSV files from distributed storage systems like Hadoop’s HDFS. The code below demonstrates how to load a large dataset in PySpark for further processing:
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName("BigData").getOrCreate()
df = spark.read.csv("large_data.csv", header=True)
df.show()
This allows you to handle enormous datasets efficiently without running into memory limitations.
Python for Time Series Analysis
Time series analysis focuses on data indexed in time order, such as stock prices, weather data, or any other data collected sequentially over time. Python provides specialized libraries to help analyze and forecast time-dependent data, making it a valuable tool in domains like finance and weather prediction.
Introduction to Time Series
Time series analysis is used for various applications like stock market analysis, predicting weather patterns, and sales forecasting. Understanding how data behaves over time helps in making informed decisions and predictions.
Time Series Decomposition
Time series decomposition is the process of breaking down time series data into three components: trend, seasonality, and residuals. This helps in understanding underlying patterns and predicting future values more accurately.
from statsmodels.tsa.seasonal import seasonal_decompose
decompose_result = seasonal_decompose(time_series, model="additive")
decompose_result.plot()
plt.show()
This method helps identify trends and seasonality, providing insights for forecasting.
Forecasting Methods
- Moving averages: Used to smooth out short-term fluctuations and highlight longer-term trends in time series data.
- ARIMA models: A more advanced method for forecasting, which combines autoregressive, integrated, and moving average techniques to predict future values based on past data.
Python for Text Data Analysis
Python is also ideal for analyzing text data, such as reviews, tweets, and articles. It helps extract valuable insights from unstructured data, which can be applied in sentiment analysis, topic modeling, and text classification.
Introduction to Text Data
Text data includes unstructured formats like emails, tweets, customer reviews, and articles. Analyzing this type of data involves transforming raw text into meaningful insights using techniques like tokenization and text preprocessing.
Text Preprocessing
- Tokenization: The process of breaking text into individual words or tokens, which is essential for understanding and processing the content.
- Removing stopwords: Removing common words such as “the” or “is,” which do not add significant meaning to the text.
- Stemming and Lemmatization: Reducing words to their base or root form to ensure consistency across similar terms.
Sentiment Analysis
Sentiment analysis is used to determine the sentiment expressed in text, such as whether a customer review is positive, negative, or neutral. This can provide insights into customer satisfaction, social media trends, and more.
from textblob import TextBlob
text = "Python is amazing!"
analysis = TextBlob(text)
print(analysis.sentiment)
The output will show polarity and subjectivity, helping gauge sentiment based on text content.
Conclusion
By understanding Python statistics techniques and leveraging libraries such as Pandas, NumPy, SciPy, and Matplotlib, you can transform raw data into actionable insights. Moreover, with tools like Dask and PySpark, Python scales to meet the demands of big data, enabling real-time processing and analysis of massive datasets.
Whether you are working on small datasets or large-scale big data projects, adopting best practices such as code optimization, modular programming, and efficient memory management will significantly enhance your analytical capabilities.
1 Comment
Abdirahman Muhudin · January 16, 2025 at 8:55 pm
I want to learn it
Comments are closed.