Data visualization plays a crucial role in transforming raw data into meaningful insights. It helps simplify complex datasets by presenting them visually, making patterns, trends, and relationships more accessible to the human eye. Data Visualization in Python Using Matplotlib stands out as one of the most widely used and versatile tools.
In this article, we’ll explore the essentials of Matplotlib, demonstrate how to create and customize plots, and introduce how it integrates seamlessly with Pandas for simplified visualization workflows.
Introduction to Matplotlib
Matplotlib is a Python library designed for creating static, interactive, and animated visualizations. It serves as the backbone of Python’s data visualization ecosystem, supporting diverse use cases, from quick exploratory analysis to building polished and publication-ready charts.
Installing Matplotlib is straightforward. You can install it via pip:
pip install matplotlib
Once installed, import the library as follows:
import matplotlib.pyplot as plt
Plotting Using Matplotlib
The core functionality of Matplotlib revolves around its ability to create a diverse range of plots, enabling users to visualize data in meaningful ways. Whether analyzing trends, comparing categories, or exploring relationships, Matplotlib provides the tools to craft compelling visual representations. Each type of plot serves a specific purpose and offers unique insights.
1. Line Plot With Matplotlib
This plot is ideal for showcasing trends or changes over time, such as stock prices or temperature fluctuations. With just a few lines of code, you can create a visually appealing and informative chart. Here’s how you can create one:
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [10, 20, 15, 25, 30]
# Creating the line plot
plt.plot(x, y, marker='o', color='blue')
plt.title("Trend Over Time")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.grid(True)
plt.show()
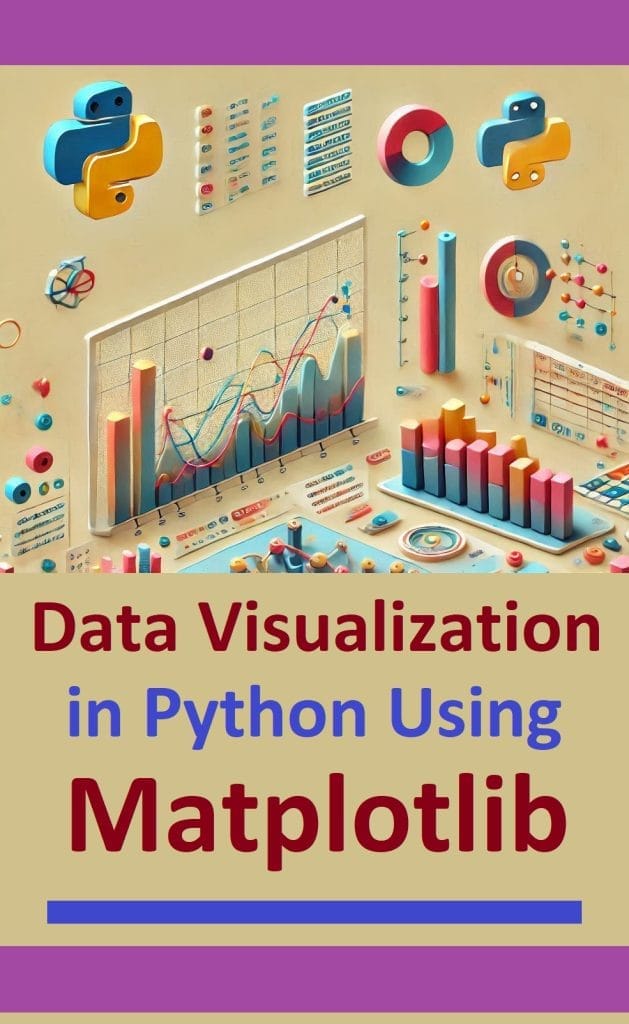
Download PDF: Data Visualization in Python Using Matplotlib
2. Bar Plot With Matplotlib
Bar plots are perfect for comparing categorical data, such as sales by region or product popularity. By representing categories on one axis and their corresponding values on the other, they make differences visually distinct. Matplotlib also lets you customize bar colors and orientations, making the plot more visually appealing and informative.
categories = ['A', 'B', 'C', 'D']
values = [5, 7, 8, 6]
plt.bar(categories, values, color='purple')
plt.title("Category Comparison")
plt.xlabel("Categories")
plt.ylabel("Values")
plt.show()
3. Scatter Plot With Matplotlib
Scatter plots reveal relationships between two variables by displaying data points in a Cartesian plane. They are often used in exploratory data analysis to uncover correlations, clusters, or outliers. With Matplotlib, you can adjust point size and color to reflect additional dimensions of data, enhancing the plot’s depth.
categories = ['A', 'B', 'C', 'D']
values = [5, 7, 8, 6]
plt.bar(categories, values, color='purple')
plt.title("Category Comparison")
plt.xlabel("Categories")
plt.ylabel("Values")
plt.show()
4. Pie Chart
Pie charts are best suited for representing proportions of a whole, such as market share or budget allocations. They divide data into slices, with each slice’s size proportional to its value. Matplotlib makes it easy to create visually compelling pie charts by adding colors, labels, and percentage annotations.
labels = ['Category A', 'Category B', 'Category C']
sizes = [40, 35, 25]
colors = ['gold', 'lightblue', 'lightgreen']
plt.pie(sizes, labels=labels, colors=colors, autopct='%1.1f%%')
plt.title("Pie Chart Representation")
plt.show()
Customization of Plots using Matplotlib
Customizing plots in Matplotlib allows you to create visuals tailored to your specific needs, enhancing their clarity, aesthetics, and impact. Whether it’s adding labels, changing styles, or annotating key points, customizations transform generic plots into insightful visualizations that convey information effectively.
1. Adding Titles and Labels
Titles and axis labels provide context to your plots, making them more comprehensible. A meaningful title captures the essence of the plot, while well-labeled axes clarify what the data represents. Adding titles and labels is straightforward, as demonstrated below:
x = [1, 2, 3, 4]
y = [10, 20, 15, 25]
plt.plot(x, y, marker='o')
plt.title("Customized Plot Example")
plt.xlabel("X-axis Label")
plt.ylabel("Y-axis Label")
plt.show()
2. Changing Styles
Matplotlib offers a variety of pre-defined styles to improve the aesthetic appeal of your plots. These styles simplify the process of creating professional-looking visuals without extensive customization:
plt.style.use('seaborn-darkgrid')
x = [1, 2, 3, 4]
y = [1, 4, 9, 16]
plt.plot(x, y, marker='o', linestyle='--', color='teal')
plt.title("Styled Plot Example")
plt.show()
3. Adding Legends
Legends are crucial when dealing with multiple datasets in the same plot. They help differentiate between datasets, ensuring the audience can interpret the data easily:
x = [1, 2, 3, 4]
y1 = [10, 20, 15, 25]
y2 = [5, 10, 5, 15]
plt.plot(x, y1, label="Dataset 1", color='blue')
plt.plot(x, y2, label="Dataset 2", color='orange')
plt.legend()
plt.title("Plot with Legend")
plt.show()
4. Annotations
Annotations emphasize specific data points or provide additional context to your visualizations. Using arrows or text annotations, you can draw attention to key insights:
x = [0, 1, 2, 3]
y = [10, 20, 25, 30]
plt.plot(x, y)
plt.annotate('Highest Point', xy=(3, 30), xytext=(2, 35),
arrowprops=dict(facecolor='black', shrink=0.05))
plt.show()
Customizing plots ensures your visualizations are not only accurate but also engaging and meaningful to your audience.
The Pandas Plot Function (Pandas Visualization)
Pandas, a powerful library for data manipulation, includes built-in visualization capabilities that leverage Matplotlib. This feature simplifies the process of generating plots by providing a straightforward interface for visualizing data directly from a DataFrame. With Pandas’ plot function, users can create a variety of charts like line plots, bar charts, histograms, and more without explicitly configuring Matplotlib, making it highly efficient for quick exploratory analysis.
1. Line Plot with Pandas
Line plots in Pandas are an excellent way to display data trends over time or across sequential categories. Using the plot method, you can specify the type of plot, axes, and stylistic elements in a single line of code. For instance, plotting monthly revenue is effortless:
import pandas as pd
# Creating a DataFrame
data = {'Month': ['Jan', 'Feb', 'Mar', 'Apr'],
'Revenue': [100, 150, 200, 250]}
df = pd.DataFrame(data)
# Line plot
df.plot(x='Month', y='Revenue', kind='line', marker='o', color='green')
plt.title("Monthly Revenue")
plt.ylabel("Revenue ($)")
plt.show()
This creates a visually appealing and informative chart with minimal effort, perfect for identifying revenue trends.
2. Bar Plot with Pandas
Bar plots are effective for comparing categorical data. They are easy to generate using Pandas’ plot method and can quickly highlight differences or patterns within categories. Here’s an example:
# Bar plot
df.plot(x='Month', y='Revenue', kind='bar', color='orange', legend=False)
plt.title("Monthly Revenue Comparison")
plt.ylabel("Revenue ($)")
plt.show()
The bar plot displays each month’s revenue in a visually distinct manner, aiding in quick comparisons between categories.
3. Histogram with Pandas
Histograms are useful for understanding the distribution of numerical data. In Pandas, histograms can be created directly from a DataFrame column:
# Generating sample data
df['Sales'] = [50, 60, 70, 80]
# Histogram
df['Sales'].plot(kind='hist', bins=5, color='blue')
plt.title("Sales Distribution")
plt.xlabel("Sales")
plt.show()
This histogram visualizes how sales values are distributed, helping identify patterns such as clusters or outliers within the data.
Conclusion
Matplotlib is a fundamental tool for data visualization in Python, offering a wide range of capabilities for creating and customizing plots. Its integration with Pandas simplifies the process further, making it an indispensable library for data analysts, scientists, and engineers. By understanding how to create and customize visualizations with Matplotlib and Pandas, you can transform raw data into actionable insights, enabling better decision-making and communication.