Python is a versatile and powerful programming language widely used in data analysis, scientific computing, and machine learning. With its robust libraries and easy-to-learn syntax, Python has become the go-to language for both beginners and professionals working with data. In this article, we will explore the essentials of Python programming for data analysis, delve into its popular libraries, and demonstrate practical examples to help you get started. We will also discuss tips for optimizing your data analysis workflows to save time and increase productivity.
Data Types and Data Structures in Python
Data analysis begins with understanding the foundational data types and structures available in Python. These elements define how data is stored and manipulated during analysis.
Key Data Types in Python
- Numeric: Includes integers (int) and floating-point numbers (float) for handling numeric computations. Integers represent whole numbers, while floats handle decimal or fractional values.
- String: Text data represented as sequences of characters, enclosed in single (‘) or double (“) quotes. Strings are useful for handling names, descriptions, or any textual data.
- Boolean: Represents binary values, True or False, crucial in logical operations and conditional statements for filtering or decision-making processes.
- NoneType: Represents the absence of a value or a null variable. It is often used as a placeholder or to signify optional data.
Common Data Structures
Python provides versatile data structures for handling complex datasets:
Lists
Lists are ordered, mutable collections of items that can store heterogeneous data, making them useful for a wide range of applications where the order of elements matters or changes.
Example: my_list = [1, ‘apple’, 3.14]
Tuples in Python
Tuples are immutable, ordered collections, ideal for data that should not change, offering more efficiency and safety for data that needs to remain constant throughout the program.
Example: my_tuple = (1, ‘banana’, 2.71)
Dictionaries
Dictionaries store data as key-value pairs, offering quick lookups, and are particularly useful for representing associative relationships, such as mapping names to ages.
Example: my_dict = {‘name’: ‘Alice’, ‘age’: 30}
Sets
Sets are unordered collections of unique elements, often used for deduplication and performing set operations like union, intersection, and difference.
Example: my_set = {1, 2, 3, 3}
DataFrames in Python
Available through the Pandas library, DataFrames are tabular data structures with labeled axes, ideal for data analysis, and can handle large datasets with complex relationships between rows and columns.
Example:
import pandas as pd
df = pd.DataFrame({'Name': ['Alice', 'Bob'], 'Age': [25, 30]})
Essential Python Libraries for Data Analysis
When working with Python for data analysis, the following libraries are indispensable:
1. Pandas
Pandas is the cornerstone of Python-based data analysis. It provides powerful tools for data manipulation, cleaning, and wrangling.
Key Features:
- DataFrame and Series objects for tabular data.
- Functions for filtering, grouping, and aggregating data.
- Handling missing data with ease, ensuring clean datasets for analysis.
- Built-in methods for merging and reshaping data efficiently.
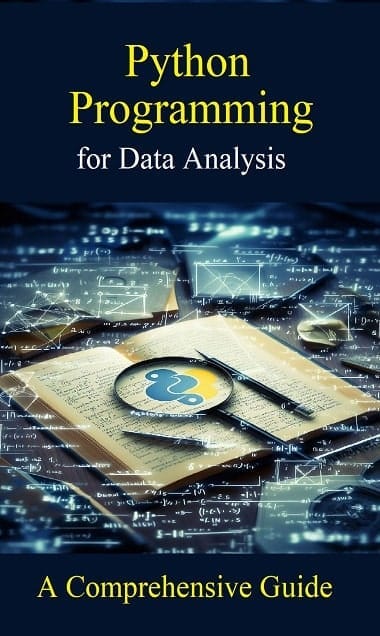
2. NumPy
NumPy is essential for numerical computations. It provides multi-dimensional array objects and mathematical functions to perform complex calculations.
- Key Features:
- Efficient storage and manipulation of numerical data.
- Operations on entire arrays without explicit loops, ensuring faster performance.
- Support for linear algebra, Fourier transforms, and random number generation, making it the backbone for numerical tasks in Python.
3. Matplotlib and Seaborn
Visualization is critical for data analysis, and these libraries make it easy to create insightful charts and graphs.
- Matplotlib: A comprehensive library for creating static, animated, and interactive visualizations, offering control over every aspect of a plot.
- Seaborn: Built on Matplotlib, it simplifies the creation of aesthetically pleasing plots with enhanced themes, color palettes, and statistical capabilities.
4. Scikit-learn
Scikit-learn is a machine learning library that provides tools for predictive data analysis. It supports tasks such as classification, regression, and clustering.
- Key Features:
- Preprocessing data for machine learning through scaling, normalization, and encoding.
- A wide range of algorithms for training models, including support for ensemble methods.
- Tools for model evaluation, such as cross-validation and metrics like accuracy and precision, to ensure reliable predictions.
Step-by-Step Guide to Data Analysis Using Python
Step 1: Data Collection
Data collection is the first step in any data analysis project. Python makes it easy to retrieve data from various sources like CSV files, databases, and APIs. Using Python’s built-in libraries ensures a seamless data gathering experience.
Example:
import pandas as pd
# Load data from a CSV file
data = pd.read_csv("sales_data.csv")
Step 2: Data Cleaning
Raw data often contains missing values, duplicates, or errors. Cleaning the data ensures that your analysis is accurate and meaningful. Common techniques include handling missing data, identifying outliers, and removing irrelevant information for consistency.
Key Functions in Pandas:
- dropna(): Remove missing values
- fillna(): Replace missing values
- duplicated(): Identify duplicates
Example:
# Fill missing values
data['column_name'].fillna(value=0, inplace=True)
Step 3: Exploratory Data Analysis (EDA)
EDA involves summarizing and visualizing the data to identify patterns and anomalies. It’s a crucial step in gaining insights before diving into detailed analysis. EDA helps guide the direction for further data transformations or model building.
Example:
# Generate summary statistics
print(data.describe())
# Create a histogram
data['column_name'].hist()
Step 4: Data Transformation
Transformation involves reshaping the data for better analysis. Techniques include normalizing data, encoding categorical variables, and scaling features. This step ensures your data is in a suitable format for statistical modeling and machine learning.
Example:
from sklearn.preprocessing import StandardScaler
# Normalize a dataset
scaler = StandardScaler()
normalized_data = scaler.fit_transform(data)
Step 5: Visualization
Visualization helps convey insights effectively. Python offers tools like line charts, scatter plots, and heatmaps. Proper visual representation allows you to better understand trends, relationships, and outliers in the dataset.
Example:
# Create a heatmap
sns.heatmap(data.corr(), annot=True)
plt.show()
Step 6: Statistical Analysis
Perform descriptive or inferential statistics to derive insights. Statistical analysis helps quantify patterns and relationships, providing a clearer picture of the underlying data. You can use mean, median, variance, and correlations to summarize key features.
Example:
mean_value = df['column'].mean()
median_value = df['column'].median()
correlation = df.corr()
Step 7: Advanced Analysis
For predictive modeling or clustering, Scikit-learn comes in handy. This step focuses on applying machine learning algorithms to make predictions or find patterns that aren’t obvious in the raw data.
Example:
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
X = df[['feature1', 'feature2']]
y = df['target']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
model = LinearRegression()
model.fit(X_train, y_train)
predictions = model.predict(X_test)
Common Challenges and How to Overcome Them
- Handling Large Datasets
Solution: Use libraries like Dask or PySpark for distributed data processing. These libraries enable parallel computation, allowing you to handle datasets too large to fit in memory by dividing the data across multiple workers or nodes. - Dealing with Missing Data
Solution: Use imputation techniques or advanced machine learning models to predict missing values. Imputation can be done using statistical methods like mean or median imputation, or advanced techniques like K-Nearest Neighbors (KNN) or regression-based models. - Scaling and Deployment
Solution: Integrate your Python scripts with cloud platforms like AWS or Google Cloud for scalability. These platforms provide scalable resources such as storage, computation, and APIs, enabling smooth deployment of large-scale data analysis tasks in a production environment.
Conclusion
Python is a powerful tool for data analysis, offering unparalleled flexibility and efficiency. By leveraging its vast ecosystem of libraries and following best practices, you can streamline your workflows and gain meaningful insights from data. Whether you’re a beginner or an experienced analyst, Python’s capabilities will empower you to tackle data challenges with confidence.
Very nice opportunity for us. Thank you.