Linear models are foundational to statistical modeling and machine learning. They serve as a cornerstone for predictive analytics, offering simplicity, interpretability, and effectiveness. This comprehensive guide delves into the core concepts of linear models with Python, such as estimation, inference, prediction, dealing with predictor issues, model selection, shrinkage methods, and handling missing data, with practical Python implementations.
What Are Linear Models?
Linear models are statistical techniques used to predict an outcome, or dependent variable, based on one or more input variables known as predictors or independent variables. These models assume a straight-line relationship between the predictors and the outcome. The key components include:
- Outcome Variable: The target variable you aim to predict.
- Predictors: Independent variables that influence the outcome.
- Intercept: The baseline value of the outcome when all predictors are zero.
- Coefficients: Values that measure the impact of each predictor.
- Error Term: The unexplained variation not captured by the predictors.
Linear models are widely applied for tasks like regression, which predicts continuous values, classification for categorical outcomes, and time-series analysis for forecasting trends. Their interpretability and simplicity make them an essential tool in statistical modeling and machine learning.
Core Concepts in Linear Models
1. Estimation
Estimation involves determining the coefficients (β) in a linear model. These coefficients represent the relationship between the predictors and the outcome. The Ordinary Least Squares (OLS) method is commonly used, as it minimizes the discrepancy between actual and predicted values, resulting in a “best-fit” line. Accurate estimation ensures better predictions and overall model performance.
Python Implementation:
from sklearn.linear_model import LinearRegression
# Fitting the model
linear_model = LinearRegression()
linear_model.fit(X_train, y_train)
# Coefficients
print("Intercept:", linear_model.intercept_)
print("Coefficients:", linear_model.coef_)
2. Inference
Inference focuses on understanding the significance of predictors and their relationships with the outcome. Statistical inference involves hypothesis testing, confidence intervals, and p-values to determine the impact of predictors.
Using statsmodels for inference:
import statsmodels.api as sm
X_train_sm = sm.add_constant(X_train) # Adding a constant for the intercept
ols_model = sm.OLS(y_train, X_train_sm).fit()
print(ols_model.summary())
The summary() method provides insights into:
- Coefficient estimates: The values that multiply the predictors to predict the outcome.
- P-values: To test whether the predictors are significantly related to the outcome. A low p-value indicates that the predictor has a meaningful relationship with the outcome.
- Confidence intervals for coefficients: These provide a range of values within which the true coefficient is likely to fall, offering insight into the precision of the estimates.
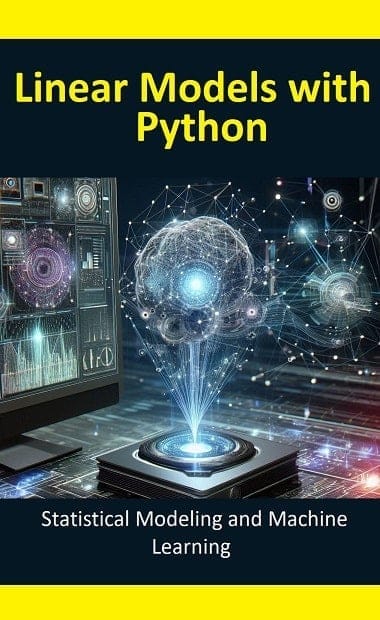
3. Prediction
Prediction is one of the primary objectives of linear models. Once a model is trained, it can be used to forecast outcomes on new, unseen data. The ability to predict allows for real-world applications such as forecasting sales, estimating house prices, or predicting customer behavior. Accurate predictions are essential for informed decision-making and risk management.
Python Implementation:
# Predicting on test data
y_pred = linear_model.predict(X_test)
# Evaluating predictions
from sklearn.metrics import mean_squared_error, r2_score
print("Mean Squared Error:", mean_squared_error(y_test, y_pred))
print("R-squared:", r2_score(y_test, y_pred))
In this example, the model predicts target values for the test dataset, and the performance is evaluated using metrics such as Mean Squared Error (MSE) and R-squared. These metrics help assess how well the model generalizes to unseen data, providing insights into its accuracy.
4. Problems with the Predictors
Linear models are sensitive to various issues with predictors, which can affect the accuracy and reliability of the model. Addressing these issues is crucial for ensuring robust and interpretable results.
A. Multicollinearity
Multicollinearity occurs when independent variables are highly correlated with each other. This makes it difficult to isolate the effect of each predictor, leading to unstable coefficient estimates. High multicollinearity can inflate standard errors, making it harder to determine the significance of predictors. To address this:
- Variance Inflation Factor (VIF) can be used to quantify how much the variance of a regression coefficient is inflated due to multicollinearity.
- If VIF values are too high (typically above 5 or 10), it may be beneficial to drop one of the correlated predictors to reduce multicollinearity.
from statsmodels.stats.outliers_influence import variance_inflation_factor
# Calculate VIF
vif_data = pd.DataFrame()
vif_data["VIF"] = [variance_inflation_factor(X.values, i) for i in range(X.shape[1])]
vif_data["Feature"] = X.columns
print(vif_data)
B. Outliers
Outliers are data points that significantly deviate from the general pattern of the dataset. These points can disproportionately influence model coefficients and predictions, leading to biased or misleading results. To handle outliers:
- Robust regression techniques like Ridge or Lasso regression can be used to reduce the impact of outliers by introducing penalty terms that limit the influence of extreme values.
- Data transformation, such as applying a logarithmic or square root transformation, can also help in reducing the effect of outliers on the model.
5. Model Selection
Selecting the right set of predictors is crucial for creating an optimal model. Choosing relevant features can significantly improve model accuracy and generalizability. Techniques include:
a. Stepwise Selection
Stepwise selection is an iterative procedure where predictors are added or removed from the model based on statistical significance. This method helps to avoid overfitting by selecting only the most influential variables.
b. Cross-Validation
Use techniques like k-fold cross-validation to evaluate model performance across different data splits.
from sklearn.model_selection import cross_val_score
cv_scores = cross_val_score(linear_model, X, y, cv=5, scoring='r2')
print("Cross-Validation R-squared Scores:", cv_scores)
print("Average R-squared Score:", cv_scores.mean())
6. Shrinkage Methods
Shrinkage methods help prevent overfitting by adding penalties to the model’s complexity, thereby reducing the magnitude of the model’s coefficients. This is particularly helpful when working with high-dimensional datasets or when there’s a risk of model overfitting.
a. Ridge Regression
Ridge regression applies an L2 penalty to the coefficients, effectively reducing their values but never setting them exactly to zero. This method helps when predictors are highly correlated, improving model generalization by minimizing large coefficient estimates. It is effective in controlling multicollinearity.
from sklearn.linear_model import Ridge
ridge_model = Ridge(alpha=1.0)
ridge_model.fit(X_train, y_train)
print("Ridge Coefficients:", ridge_model.coef_)
b. Lasso Regression
Lasso regression adds an L1 penalty, which can shrink some coefficients to zero, performing automatic feature selection. By penalizing the absolute values of the coefficients, Lasso helps identify the most important predictors and discard irrelevant ones, leading to simpler and more interpretable models.
from sklearn.linear_model import Lasso
lasso_model = Lasso(alpha=0.01)
lasso_model.fit(X_train, y_train)
print("Lasso Coefficients:", lasso_model.coef_)
7. Handling Missing Data
Missing data is a common challenge that can introduce bias and reduce the accuracy of predictive models. Various strategies can help handle missing data to prevent model performance degradation:
a. Imputation
Imputation is the process of filling in missing values with statistical estimates. Common techniques include replacing missing values with the mean, median, or mode of the existing data.
from sklearn.impute import SimpleImputer
imputer = SimpleImputer(strategy='mean')
X_imputed = imputer.fit_transform(X)
b. Removing Rows/Columns
Another approach is to remove rows or columns with a high percentage of missing data. This method is best suited for situations where the missing data is not critical, and the remaining data can provide a robust model. But use this method cautiously to avoid information loss.
c. Advanced Imputation
Advanced imputation methods, such as predictive models, can provide more accurate estimates of missing values by modeling relationships in the data. Techniques like Iterative Imputation use machine learning algorithms to predict missing values based on other available features.
from sklearn.experimental import enable_iterative_imputer
from sklearn.impute import IterativeImputer
iter_imputer = IterativeImputer()
X_imputed_advanced = iter_imputer.fit_transform(X)
Conclusion
Linear models are versatile and foundational for predictive modeling. With tools like Python, you can easily estimate coefficients, perform inference, predict outcomes, handle challenges with predictors, apply shrinkage methods, and manage missing data effectively. By addressing these challenges and optimizing your model, you can unlock valuable insights and make informed decisions.