In the era of data-driven decisions, analyzing and interpreting data is crucial. Statistics and machine learning in Python have become important and a dominant tool for scientific computing, statistics, machine learning, and deep learning. With its extensive libraries and frameworks, Python allows developers, researchers, and data scientists to perform complex data analysis and build robust machine learning models efficiently.
This article explores the use of scientific Python tools for data manipulation, statistical analysis, and machine learning, while also delving into advanced deep learning techniques.
Scientific Python: Core Tools for Data Analysis
Python’s ecosystem of scientific libraries provides robust tools for analyzing and visualizing data, making it the preferred choice for data scientists and researchers. These libraries allow for efficient data handling, statistical analysis, and insightful visualizations, enabling users to derive meaningful patterns and trends.
1. NumPy: Arrays and Matrices
NumPy is the backbone of scientific computing in Python. It introduces multi-dimensional array objects and a range of mathematical functions to operate on these arrays efficiently. Unlike Python’s native lists, NumPy arrays are optimized for performance, especially for large datasets. NumPy also supports advanced linear algebra operations such as matrix inversion, determinant calculation, and eigenvalue decomposition. For instance:
Creating Arrays:
import numpy as np
array = np.array([1, 2, 3, 4])
print(array)
Matrix Operations:
matrix = np.array([[1, 2], [3, 4]])
print(np.linalg.inv(matrix)) # Matrix inversion
2. Pandas: Data Manipulation
Pandas is indispensable for working with structured data. It simplifies tasks such as cleaning, transforming, and analyzing tabular data. DataFrames, one of Pandas’ primary structures, offer intuitive functionality for slicing, aggregating, and summarizing data. For example:
DataFrame Creation and Manipulation:
import pandas as pd
data = {'Name': ['Alice', 'Bob'], 'Age': [25, 30]}
df = pd.DataFrame(data)
print(df.describe()) # Summary statistics
3. Data Visualization: Matplotlib and Seaborn
Visualization is a cornerstone of data analysis, and Python’s Matplotlib and Seaborn libraries make this task efficient and aesthetically pleasing.
- Matplotlib: A powerful yet flexible tool for creating static, animated, and interactive plots. For example:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3], [4, 5, 6])
plt.title('Line Plot')
plt.show()
- Seaborn: Seaborn: Built on Matplotlib, Seaborn simplifies creating complex visualizations such as heatmaps, boxplots, and pairplots with minimal code:
import seaborn as sns
sns.boxplot(x=['A', 'B', 'C'], y=[5, 8, 2])
These tools collectively form the foundation of Python’s capabilities for analyzing and visualizing scientific data.
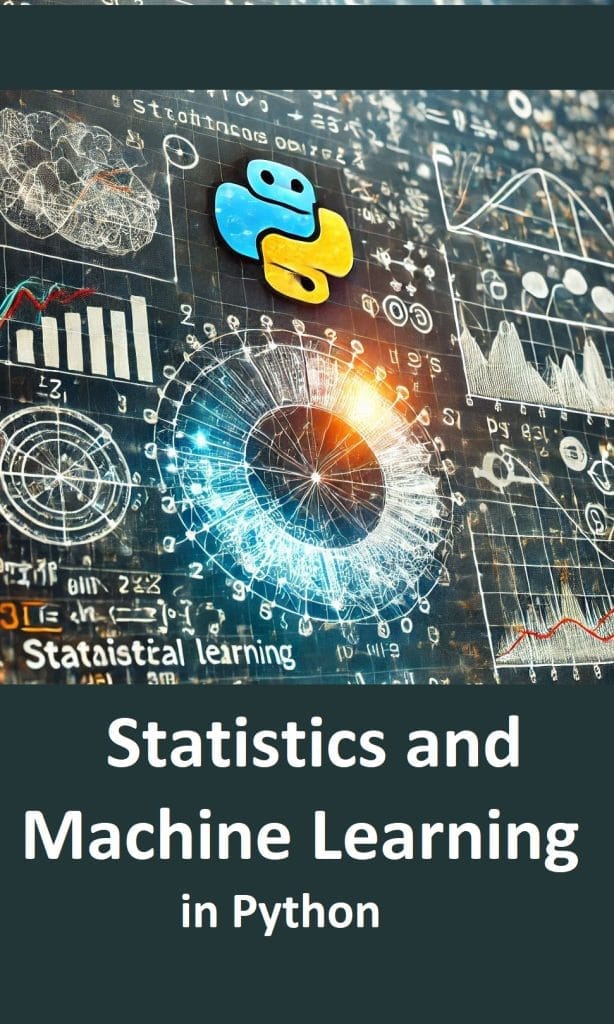
Download PDF: Statistics and Machine Learning in Python
Statistics in Python
Statistics is a cornerstone of data analysis, providing tools to summarize, interpret, and infer patterns from data. Python offers extensive libraries to perform both basic and advanced statistical analysis efficiently. Below, we expand on various statistical techniques that Python supports.
1. Univariate Statistics
Univariate analysis explores one variable at a time. Using Python, you can calculate measures like mean, median, and variance. Using Python libraries such as scipy and numpy, calculations become straightforward. For instance, the scipy.stats.describe function offers a quick overview, including metrics like skewness and kurtosis, helping you understand the distribution of the data.
Example:
from scipy.stats import describe
data = [4, 7, 1, 8, 3]
print(describe(data))
2. Brain Volumes Study
Analyzing brain volume data can help identify patterns and anomalies in neurological studies, such as detecting the effects of diseases like Alzheimer’s. Python’s scipy.stats module facilitates hypothesis testing, such as t-tests and ANOVA, to compare brain volumes between groups. These tests provide statistical evidence to support or reject hypotheses about group differences, offering actionable insights in clinical and research settings.
Hypothesis Testing: Use scipy.stats for t-tests and ANOVA.
from scipy.stats import ttest_ind
t_stat, p_val = ttest_ind([3.2, 3.5, 3.1], [3.8, 4.0, 3.6])
print(p_val)
3. Linear Mixed Models
Linear mixed models (LMMs) are invaluable for datasets with hierarchical structures, such as repeated measures or multi-level experiments. Python’s statsmodels library provides tools to fit LMMs, allowing researchers to model both fixed and random effects. These models are particularly useful in biomedical studies and social sciences where data often exhibit nested dependencies.
Library: Use statsmodels for implementation.
4. Multivariate Statistics
Multivariate statistics examine relationships among multiple variables simultaneously, providing insights that univariate analysis cannot. Techniques like Principal Component Analysis (PCA) reduce dimensionality while retaining essential data variance. Python’s sklearn library simplifies PCA, making it accessible for applications in exploratory data analysis, image compression, and feature selection in machine learning.
Principal Component Analysis (PCA):
from sklearn.decomposition import PCA
pca = PCA(n_components=2)
transformed_data = pca.fit_transform([[1, 2], [3, 4], [5, 6]])
5. Time Series Analysis
Time series analysis focuses on sequential data, identifying trends, seasonality, and forecasting future values. Python’s statsmodels and pandas libraries are widely used for handling and modeling time series. For example, ARIMA (AutoRegressive Integrated Moving Average) models allow for robust forecasting, which is vital in industries like finance, weather prediction, and inventory management.
Example:
from statsmodels.tsa.arima.model import ARIMA
model = ARIMA([1, 2, 3, 4], order=(1, 1, 1))
fitted = model.fit()
print(fitted.summary())
Machine Learning in Python
Machine learning is a branch of artificial intelligence focused on developing algorithms that allow systems to learn patterns from data and make decisions without being explicitly programmed. Python’s vast ecosystem of libraries and tools makes it an ideal language for implementing machine learning techniques across various domains. Below, we explore essential topics and methods in machine learning, focusing on dimension reduction, clustering, linear and non-linear models, resampling, ensemble learning, and optimization techniques.
1. Linear Dimension Reduction and Feature Extraction
High-dimensional data can lead to overfitting and computational inefficiency. Linear dimension reduction techniques like Principal Component Analysis (PCA) simplify data by retaining only the most significant features, enabling faster and more accurate modeling. PCA transforms data into a new coordinate system where the axes (principal components) capture the maximum variance in descending order. This technique is widely used in preprocessing pipelines to reduce noise and improve model performance. For implementation, refer to the Multivariate Statistics section.
2. Manifold Learning (Non-Linear Dimension Reduction)
While linear methods like PCA are effective, real-world data often lies on non-linear manifolds. Techniques such as t-Distributed Stochastic Neighbor Embedding (t-SNE) and Locally Linear Embedding (LLE) uncover complex structures within data, making them suitable for tasks like visualization and clustering. Manifold learning provides a way to project data onto a low-dimensional space while preserving relationships that linear methods might miss.
t-SNE:
from sklearn.manifold import TSNE
tsne = TSNE(n_components=2)
reduced_data = tsne.fit_transform([[1, 2], [3, 4], [5, 6]])
3. Clustering
Clustering partitions data into groups with similar characteristics. Algorithms like k-Means and DBSCAN are popular for unsupervised learning tasks, such as customer segmentation and anomaly detection. These methods identify inherent patterns in unlabeled data, providing insights into group behaviors.
k-Means Clustering:
from sklearn.cluster import KMeans
kmeans = KMeans(n_clusters=3)
kmeans.fit([[1], [2], [3], [4], [5]])
4. Linear Models for Regression and Classification
Linear models form the backbone of machine learning due to their simplicity and interpretability. Linear regression predicts continuous outcomes by modeling relationships between variables. Logistic regression, on the other hand, is used for binary classification problems, estimating probabilities using a sigmoid function.
Linear Regression:
from sklearn.linear_model import LinearRegression
model = LinearRegression()
model.fit([[1], [2], [3]], [4, 6, 8])
Logistic Regression:
from sklearn.linear_model import LogisticRegression
model = LogisticRegression()
model.fit([[1, 0], [0, 1], [1, 1]], [0, 1, 1])
5. Non-Linear Models
Non-linear models handle more complex relationships within data. Decision trees create hierarchical decision-making structures, while support vector machines (SVMs) map data to high-dimensional spaces to find optimal classification boundaries. These models are versatile and can be applied to diverse tasks, including image recognition and text analysis
SVM Example:
from sklearn.svm import SVC
model = SVC()
model.fit([[1], [2], [3]], [0, 1, 0])
6. Resampling Methods
Resampling methods like cross-validation improve model evaluation by splitting data into training and testing sets multiple times. This approach ensures robust performance metrics, reducing the risk of overfitting and enhancing generalizability.
7. Ensemble Learning
Ensemble methods such as bagging, boosting, and stacking combine multiple models to achieve better accuracy and robustness. Boosting algorithms like Gradient Boosting and AdaBoost sequentially train weak learners, correcting their errors to build strong predictive models.
Boosting (e.g., Gradient Boosting):
from sklearn.ensemble import GradientBoostingClassifier
model = GradientBoostingClassifier()
8. Gradient Descent
Gradient descent is an optimization algorithm used to minimize a model’s error by iteratively adjusting parameters. By computing gradients of the loss function, gradient descent ensures convergence toward an optimal solution, playing a critical role in training machine learning and deep learning models.
Deep Learning in Python
Deep learning represents a subset of machine learning focusing on neural networks with multiple layers, often referred to as deep neural networks. Python’s ecosystem, particularly libraries like TensorFlow and PyTorch, makes it easier to implement and train these networks. Below, we expand on key aspects of deep learning in Python.
1. Backpropagation
Backpropagation is the cornerstone of deep learning, enabling neural networks to learn by adjusting weights based on the error gradient. It uses the chain rule of calculus to compute the gradient of the loss function concerning each weight in the network. This process iteratively minimizes the error, optimizing the model’s performance over time. Python frameworks like TensorFlow and PyTorch automate backpropagation, significantly reducing implementation complexity.
2. Multilayer Perceptron (MLP)
An MLP is a fully connected neural network composed of multiple layers of neurons. It includes an input layer, one or more hidden layers, and an output layer. MLPs are highly effective for supervised learning tasks such as classification and regression. They rely on activation functions like ReLU or sigmoid to introduce non-linearity, making the network capable of learning complex patterns.
Example Using Keras:
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
model = Sequential([Dense(10, activation='relu'), Dense(1)])
model.compile(optimizer='adam', loss='mse')
3. Convolutional Neural Networks (CNNs)
CNNs specialize in processing grid-like data structures, such as images, making them the preferred choice for tasks like image recognition and object detection. They use convolutional layers to detect features such as edges and textures, pooling layers for dimensionality reduction, and fully connected layers for classification. CNNs are scalable and have revolutionized computer vision by achieving human-like accuracy on various tasks.
Example:
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten
model.add(Conv2D(32, (3, 3), activation='relu'))
model.add(MaxPooling2D((2, 2)))
model.add(Flatten())
4. Transfer Learning Tutorial
Transfer learning allows you to leverage pre-trained models, such as VGG16, ResNet, or Inception, to solve new but related problems. By reusing the feature extraction layers of these models, you can reduce training time and achieve better accuracy even with limited data. You can fine-tune the pre-trained model or add custom layers for your specific task.
Using Pre-Trained Models:
from tensorflow.keras.applications import VGG16
base_model = VGG16(weights='imagenet', include_top=False)
Conclusion
Python’s capabilities in statistics, machine learning, and deep learning are unparalleled, thanks to its comprehensive libraries and frameworks. By mastering tools like NumPy, pandas, and scikit-learn, you can handle diverse data challenges efficiently. Furthermore, deep learning frameworks such as TensorFlow empower you to build sophisticated neural networks for real-world applications. Whether you’re a researcher, data scientist, or developer, Python provides all the tools you need to extract meaningful insights from data.