The rapid evolution of technology has revolutionized how we interact with spatial data, making Geographic Information Systems (GIS) and remote sensing crucial tools in multiple industries. With Python 3’s extensive libraries and capabilities, learning geospatial analysis has become both accessible and powerful for beginners and experts alike.
This article explores how to perform geospatial analysis with Python, focusing on high-value applications, tools, and resources. Additionally, we will discuss its integration with remote sensing techniques and GIS platforms, empowering you to unlock the potential of spatial data.
What Is Geospatial Analysis?
Geospatial analysis refers to analyzing and interpreting data that has a geographical or spatial component. It allows users to identify patterns, relationships, and trends within datasets tied to specific locations on Earth’s surface. Applications span from urban planning, environmental monitoring, and transportation optimization to business site selection and disaster management.
GIS and Remote Sensing: A Brief Overview
- Geographic Information Systems (GIS): GIS refers to frameworks that gather, manage, and analyze spatial data. It integrates layers of data for visualizations, modeling, and decision-making. GIS is widely used in industries like agriculture, logistics, and government planning.
- Remote Sensing: This is the process of acquiring data about the Earth’s surface using satellite or aerial sensors. It provides critical data for environmental monitoring, weather prediction, and land-use mapping.
Combining GIS with remote sensing creates a powerful toolkit for understanding and addressing real-world challenges. And Python, with its extensive libraries, makes it easier to achieve this integration.
Key Python Libraries for GIS and Remote Sensing
1. Geopandas
Geopandas extends the functionalities of pandas to work with geospatial data. It simplifies operations like spatial joins, buffering, and projection handling.
Example use case: Loading a shapefile and visualizing it.
import geopandas as gpd
import matplotlib.pyplot as plt
# Load shapefile
gdf = gpd.read_file("path_to_shapefile.shp")
# Plot shapefile
gdf.plot()
plt.show()
2. Rasterio
Rasterio is a powerful library for working with raster data, such as satellite images. It supports reading, writing, and transforming raster datasets.
Example use case: Reading a satellite image and plotting its data.
import rasterio
import matplotlib.pyplot as plt
# Open raster file
raster = rasterio.open("path_to_raster.tif")
# Display raster
plt.imshow(raster.read(1), cmap="gray")
plt.show()
3. Shapely
Shapely enables geometric operations on spatial data. Use it for tasks like creating buffers, calculating areas, or finding intersections.
Example: Creating a buffer around a point.
from shapely.geometry import Point
from shapely.geometry import Polygon
# Create a point
point = Point(1.0, 1.0)
# Create a buffer
buffer = point.buffer(1.0)
print(buffer)
4. Folium
Folium integrates well with Python to create interactive maps. You can overlay data on web maps for dynamic visualizations.
Example: Plotting a location on a map.
import folium
# Create a map centered at a specific location
map = folium.Map(location=[40.7128, -74.0060], zoom_start=12)
# Add a marker
folium.Marker([40.7128, -74.0060], popup="New York City").add_to(map)
map.save("map.html")
5. EarthPy
- Description: EarthPy simplifies tasks like handling spatial data, visualizing rasters, and working with shapefiles.
- Applications: Processing satellite imagery and time-series analysis.
Applications of Python 3 in GIS and Remote Sensing
1. Urban Planning
Python’s geospatial libraries like geopandas
and shapely
allow planners to analyze spatial data effectively for urban development. By overlaying demographic data, proximity to transportation networks, and existing infrastructure, planners can pinpoint optimal locations for housing projects, commercial zones, or green spaces. Additionally, Python integrates with platforms like QGIS, enabling seamless workflows for zoning analysis, traffic modeling, and noise pollution studies. This integration helps governments and organizations design smarter, more sustainable cities.
2. Environmental Monitoring
Python streamlines environmental monitoring by processing and visualizing satellite imagery with libraries like rasterio
and earthpy
. For instance, it helps track deforestation by calculating NDVI (Normalized Difference Vegetation Index) or monitor air and water quality in real-time. The Google Earth Engine Python API provides access to global-scale datasets, enabling researchers to map changes in forest cover, glacier retreat, and urban heat islands over time.
3. Disaster Management
Python aids disaster preparedness and response by analyzing remote sensing data. Using machine learning algorithms with libraries like scikit-learn
, researchers can predict flooding based on rainfall patterns or assess damage post-earthquake by detecting structural changes in satellite images. Additionally, Python’s visualization tools, such as folium
, can create real-time maps of disaster-prone areas, helping authorities optimize evacuation routes and resource allocation.
4. Agriculture
In agriculture, Python enables precision farming by integrating satellite and IoT data for informed decision-making. For example, Python can calculate vegetation indices like NDVI to assess crop health or use weather APIs to predict irrigation needs. Libraries like pandas
and matplotlib
support the analysis and visualization of soil composition, pest distribution, and yield forecasts. This empowers farmers to manage resources efficiently, reduce costs, and maximize productivity.
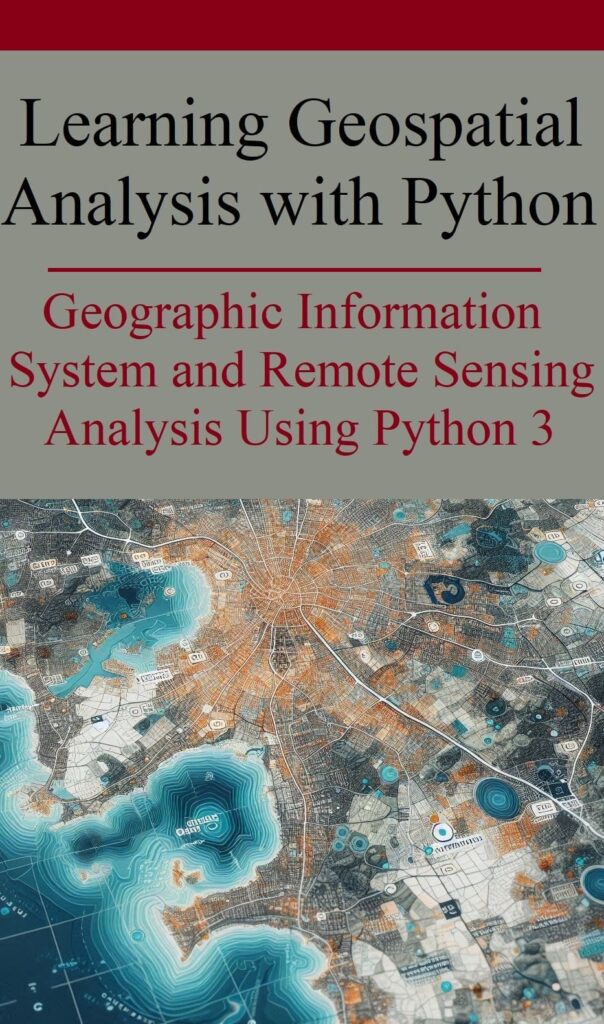
Download PDF: Learning Geospatial Analysis with Python
Step-by-Step Guide to GIS and Remote Sensing Analysis Using Python 3
Performing GIS and remote sensing analysis with Python involves a systematic approach. Here’s an expanded step-by-step guide:
Step 1: Installing Required Libraries
Before diving into geospatial analysis, ensure Python 3 is installed. Next, use pip
to install essential libraries such as geopandas
for vector data, rasterio
for raster processing, shapely
for geometric operations, folium
for interactive mapping, and earthpy
for managing spatial datasets. These tools form the backbone of geospatial workflows.
Command:
pip install geopandas rasterio shapely folium earthpy
Step 2: Loading and Visualizing Data
Load geospatial datasets using geopandas
for shapefiles or rasterio
for raster data. Visualize the data with matplotlib
for static maps or folium
for interactive, web-friendly maps. This step ensures you understand your dataset’s structure and spatial distribution.
Step 3: Preprocessing Data
Data preprocessing involves cleaning, clipping, filtering, and reprojection to ensure uniform coordinate systems and compatibility between datasets. Preprocessing is crucial for accurate analysis.
Step 4: Analysis
Perform tasks like spatial joins, buffer creation, or raster calculations. For instance, calculate vegetation indices or analyze spatial relationships within the data.
Step 5: Visualization
Create insightful visualizations to present your analysis. Use folium
to generate interactive maps with pop-ups, markers, and overlays, or matplotlib
for publication-ready visuals.
Step 6: Automating Workflows
Streamline repetitive tasks by scripting workflows for data ingestion, preprocessing, and analysis. Automation reduces errors and improves efficiency, especially for large datasets.
Case Study: Mapping Deforestation Using Python
Deforestation is a critical environmental issue, and satellite imagery offers an efficient way to monitor and quantify changes in forest cover over time. In this case study, we explore how to map deforestation using Landsat imagery and Python. The process involves classifying land cover changes by calculating vegetation health using the Normalized Difference Vegetation Index (NDVI).
Steps to Map Deforestation
Acquire Landsat Data:
Landsat imagery can be downloaded from sources like USGS Earth Explorer or NASA’s Earthdata platform. These datasets typically include multiple spectral bands, such as Near-Infrared (NIR) and Red, essential for vegetation analysis.Preprocess the Data:
Preprocessing includes clipping, filtering, and aligning the raster images to your area of interest. Tools likerasterio
are ideal for handling this. Preprocessing ensures the data is clean and ready for analysis.Analyze NDVI:
NDVI is calculated to assess vegetation health. Healthy vegetation reflects more NIR light and absorbs Red light. The NDVI formula is:NDVI= (NIR−Red) / (NIR+Red)
A high NDVI value indicates dense, healthy vegetation, while lower values suggest sparse or degraded vegetation, often associated with deforestation.
Visualize Results:
The NDVI results can be visualized using Python libraries likematplotlib
for static maps orfolium
for interactive web-based maps. Highlighting areas with low NDVI values can help identify deforestation hotspots.
By following these steps, you can effectively monitor forest loss and support environmental conservation efforts. This case study demonstrates Python’s power in geospatial analysis and environmental monitoring.
Example Python code:
import rasterio
import numpy as np
import matplotlib.pyplot as plt
# Open raster bands
nir_band = rasterio.open("path_to_nir_band.tif")
red_band = rasterio.open("path_to_red_band.tif")
# Calculate NDVI
nir = nir_band.read(1).astype(float)
red = red_band.read(1).astype(float)
ndvi = (nir - red) / (nir + red)
# Display NDVI
plt.imshow(ndvi, cmap="RdYlGn")
plt.colorbar()
plt.title("NDVI Map")
plt.show()
Future Trends in Python-Based Geospatial Analysis
The field of geospatial analysis continues to evolve, driven by advancements in Python and related technologies.
Artificial Intelligence Integration: Machine learning models, implemented using Python libraries like TensorFlow, PyTorch, and Scikit-learn, are transforming geospatial analysis. AI-powered applications now classify land cover, predict urban growth patterns, and detect changes in environmental features. For instance, convolutional neural networks (CNNs) are widely used for object detection in satellite imagery, enabling precise identification of infrastructure or natural features.
Cloud Computing: Platforms like Google Earth Engine (GEE) and AWS Lambda facilitate large-scale geospatial computations. These cloud platforms enable processing of massive spatial datasets, such as satellite imagery, without local hardware limitations. GEE’s Python API simplifies data retrieval and analysis, making it a go-to tool for environmental scientists and urban planners.
Real-Time Analysis: Python’s integration with IoT devices and APIs empowers real-time spatial data analysis. Applications range from monitoring traffic flow and disaster management to tracking environmental changes using live sensor data and automated pipelines.
Conclusion
Python 3 has revolutionized the way GIS and Remote Sensing analysis is conducted, offering unparalleled flexibility, scalability, and cost-efficiency. By leveraging Python’s extensive libraries and tools, professionals and researchers can derive actionable insights from geospatial data, contributing to sustainable development and informed decision-making. As the technology evolves, Python will remain a cornerstone in geospatial analysis, driving innovation and enabling transformative solutions.
Leave a Reply