Python is one of the most versatile and user-friendly programming languages, widely recognized for its simplicity and extensive library ecosystem. However, when it comes to performance-intensive applications, developers often face challenges in maximizing Python’s efficiency due to its interpreted nature.
In this comprehensive guide, we’ll explore strategies, tools, and practices for optimizing Python performance, ensuring of high-performance Python applications development which are both powerful and efficient. Understanding how to optimize Python code not only improves execution speed but also reduces computational costs – key for businesses scaling cloud-based applications.
1. Choose the Right Data Structures in Python
Efficient data structures are the cornerstone of high-performance Python programming. Selecting the right data structure in Python can significantly enhance both speed and memory efficiency. The collections module provides versatile tools tailored for specific needs:
- deque: A double-ended queue, ideal for scenarios requiring rapid append and pop operations at both ends. It outperforms lists in such cases.
- defaultdict: Simplifies dictionary operations by eliminating key existence checks, making it perfect for grouping or categorization tasks.
- Counter: Streamlines counting operations, such as word frequency analysis.
- namedtuple: Provides immutable, lightweight objects with named fields, combining the efficiency of tuples with the clarity of dictionaries.
For large-scale data processing, libraries like NumPy and Pandas excel. NumPy arrays allow vectorized operations, reducing loop overhead, while Pandas DataFrames enable efficient handling of tabular data with optimized indexing and querying. Using these advanced tools instead of traditional structures can dramatically improve computational performance.
2. Utilize Built-In Functions and Libraries
Python’s built-in functions, implemented in C, are inherently faster than custom Python code for similar tasks. Functions like map(), filter(), and sum() operate at a lower level, offering optimized performance for operations like iteration, filtering, and aggregation. Using these functions not only saves time but also ensures more readable and concise code.
In addition, Python’s rich ecosystem of specialized libraries provides pre-optimized solutions for complex tasks. For example:
- NumPy accelerates numerical computations with powerful array manipulation tools.
- Pandas simplifies data manipulation and analysis with its intuitive DataFrame structures.
- SciPy extends Python’s scientific computing capabilities.
- Cython compiles Python code into C, dramatically improving execution speed.
These libraries significantly reduce the overhead of Python’s interpreted runtime, making applications faster and more efficient.
3. Python Profiling Tools: Profiling Your Code for Bottlenecks
Profiling is a crucial step in understanding how your Python code performs under different conditions. By identifying bottlenecks, you can focus on optimizing the most resource-intensive sections of your application. Python offers several robust Python profiling tools:
- cProfile: A built-in tool that provides an overview of execution time, helping you analyze the time spent in each function.
- line_profiler: Offers detailed, line-by-line execution insights, ideal for pinpointing slow code sections.
- memory_profiler: Monitors memory consumption, helping reduce unnecessary memory usage.
For example, using cProfile:
import cProfile
cProfile.run('your_function()')
Outputs a report highlighting the time spent in each part of the function, making it easier to prioritize optimization efforts. Profiling ensures that your improvements target real performance constraints.
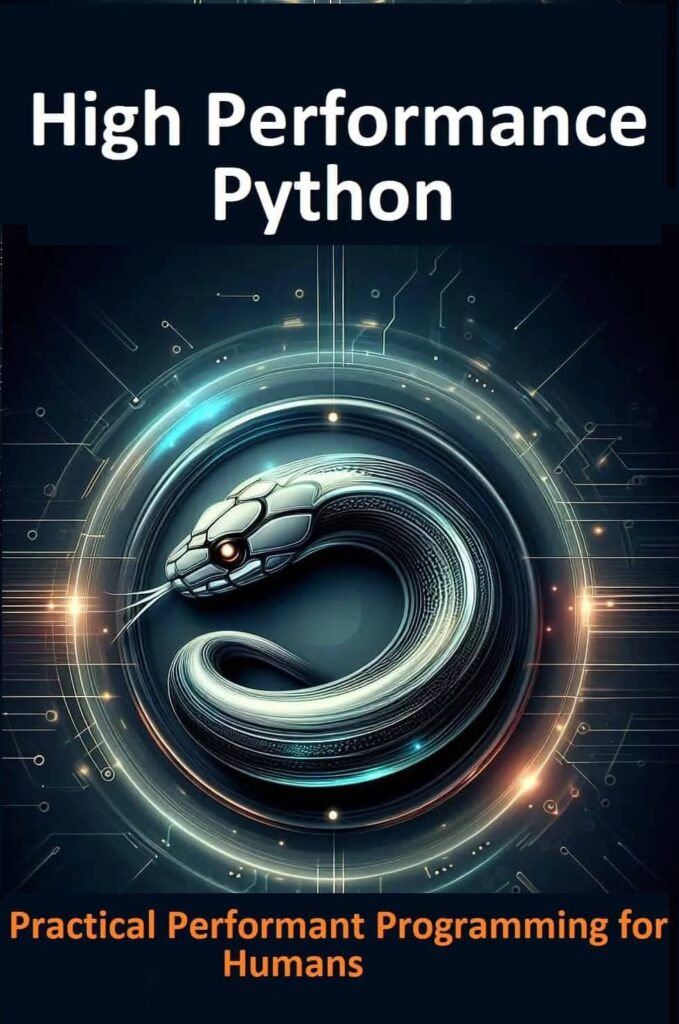
Download PDF: High Performance Python
4. Python Parallel Programming: Leveraging Parallelism and Concurrency
Python’s Global Interpreter Lock (GIL) restricts the execution of multiple threads within a single Python process, often becoming a bottleneck for multi-threaded programs. However, several strategies can help mitigate this limitation:
- Threading: Effective for I/O-bound tasks such as file handling or network requests, where threads can perform operations concurrently while waiting for I/O responses.
- Multiprocessing: Launches multiple independent processes, each with its own Python interpreter and memory space, making it suitable for CPU-bound tasks like data crunching or mathematical computations.
- Asyncio: Facilitates asynchronous programming, excelling in tasks requiring high concurrency like web scraping, API calls, or event-driven architectures.
For compute-heavy workloads, libraries like Numba (for JIT compilation) and Dask (for parallelizing large computations) provide significant performance boosts.
5. Optimizing Code Execution with Cython
Cython is a powerful tool that compiles Python code into C, significantly enhancing execution speed by bridging the gap between Python’s simplicity and C’s efficiency. It works seamlessly with existing Python code, making it ideal for optimizing performance-critical functions. To start:
- Install Cython using pip install cython.
- Convert .py files to .pyx by adding Cython-specific annotations where necessary.
- Compile these .pyx files into C code using cythonize.
For example, this simple Python function:
def add_numbers(a, b):
return a + b
Can be transformed into a Cython equivalent for optimized performance, reducing execution time for computationally intensive tasks. Cython also supports type declarations, allowing for even greater speed-ups.
6. Implementing Just-In-Time Compilation
For compute-intensive tasks, Just-In-Time (JIT) compilation can revolutionize performance by dynamically translating Python code into efficient machine code during execution. Tools like Numba make this process seamless, enabling developers to optimize functions without rewriting them in a lower-level language. By simply adding the @jit decorator, Numba analyzes the function and compiles it into optimized machine code, bypassing Python’s interpreter overhead.
For example:
from numba import jit
@jit(nopython=True)
def compute():
for i in range(1000000):
pass
Here, the nopython=True argument ensures maximum performance by forcing strict type inference. This technique is especially beneficial for numerical computations and repetitive operations, delivering speed-ups comparable to C or Fortran in many cases.
7. Memory Optimization in Python: Reducing Memory Usage
Memory optimization in Python is as crucial as speed, especially in applications handling large datasets or running on resource-constrained environments. Efficient memory usage can drastically improve performance and scalability. Here are some practical tips to minimize memory consumption in Python programs:
- Use Generators: Generators allow you to yield items one at a time instead of loading all items into memory. This is particularly useful for processing large data files or streaming data. For example, replacing a list comprehension with a generator can reduce memory overhead substantially.
Example: Replace [x**2 for x in range(1000000)] with (x**2 for x in range(1000000)). - Prefer Tuples Over Lists: When working with immutable data, opt for tuples instead of lists. Tuples require less memory and offer faster access times due to their immutability.
- Apply __slots__ in Custom Classes: By defining __slots__, you prevent the creation of a default dynamic attribute dictionary (__dict__) for instances of the class. This reduces memory usage by pre-allocating space only for the specified attributes.
Example:
class MyClass:
__slots__ = ('attr1', 'attr2')
def __init__(self, attr1, attr2):
self.attr1 = attr1
self.attr2 = attr2
Compared to:
class MyClass:
def __init__(self, attr1, attr2):
self.attr1 = attr1
self.attr2 = attr2
The use of __slots__ can save considerable memory in scenarios involving thousands of instances, making it an invaluable tool for memory-constrained applications. By incorporating these strategies, you can achieve significant improvements in memory efficiency without compromising functionality.
8. Using Python’s Fast Libraries
When performance is critical, replace pure Python implementations with libraries written in C or C++. Libraries like:
- PyTorch or TensorFlow for machine learning provide highly optimized functions that allow efficient training and deployment of deep learning models.
- FastAPI for web frameworks is designed to offer rapid performance while enabling asynchronous processing for fast responses.
- Blaze or Vaex for big data processing can handle massive datasets efficiently, using memory-mapping and lazy evaluation to perform operations without loading the entire dataset into memory at once. These libraries offer pre-optimized solutions for complex tasks.
9. Avoiding Common Pitfalls
Here are a few performance-draining practices to avoid:
- Using Global Variables: Global variables require Python to perform a lookup in the global scope, which is slower than local lookups. Limit their usage, and prefer passing variables explicitly to functions.
- Excessive Loop Nesting: Deeply nested loops can significantly slow down execution. Replace these with vectorized operations or use libraries like NumPy, which allow operations on entire arrays in a single call, reducing the need for loops.
- Unnecessary Object Creation: Creating objects repeatedly within functions can cause unnecessary overhead. Instead, reuse existing objects or use more efficient data structures.
10. Cache Results with Memoization
Memoization is a powerful technique that stores the results of expensive function calls and reuses them when the same inputs occur again. By using functools.lru_cache, you can save computation time by preventing the re-execution of costly recursive functions. This is especially helpful in algorithms like Fibonacci, where overlapping subproblems are common. Memoization helps optimize performance without changing the structure of the code.
from functools import lru_cache
@lru_cache(maxsize=None)
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
This approach drastically reduces computation time for recursive functions.
11. Integrating Low-Level Languages
When Python doesn’t meet performance needs, integrate it with lower-level languages:
- C++ with Boost.Python or pybind11, which provide seamless interoperability between Python and C++ for computationally intensive tasks.
- Rust with PyO3, a modern alternative that allows for the creation of high-performance Python extensions with Rust.
These integrations allow you to retain Python’s simplicity while leveraging the raw performance of compiled languages, making them ideal for performance-critical applications.
12. Testing and Benchmarking
Continuous testing and benchmarking ensure your performance optimizations are effective. By regularly testing your code, you can verify that each optimization leads to tangible improvements. Use libraries like:
- pytest-benchmark: For benchmarking test suites, ensuring consistent performance across different test scenarios.
- timeit: For measuring execution times, helping to identify potential bottlenecks.
For instance:
import timeit
print(timeit.timeit("x = sum(range(1000))", number=1000))
13. Deploying Efficiently
Finally, deploying Python applications efficiently is critical. Use:
- Docker: For consistent environments, Docker ensures that your application runs reliably across different platforms, eliminating the “it works on my machine” issue.
- Gunicorn or uWSGI: For deploying web applications, these tools provide high-performance WSGI servers that handle multiple requests concurrently, ensuring fast response times.
- Cloud Optimization: Optimize your code to reduce costs on platforms like AWS, Azure, or Google Cloud by leveraging serverless architectures and auto-scaling features.
Conclusion
High-performance Python is achievable with the right practices and tools. From selecting efficient data structures to leveraging JIT compilers and low-level integrations, the strategies outlined in this guide will empower you to write performant Python code. Remember, optimizing code is not just about speed—it’s about resource efficiency, scalability, and cost reduction.