Python is one of the most popular programming languages in the world due to its simplicity and readability. However, even experienced developers encounter errors while coding. Understanding troubleshooting, error handling, and debugging in Python code is essential for writing efficient and bug-free programs. This guide will help you navigate the common errors in Python, effective debugging strategies, and best practices to improve your code quality. By the end, you’ll have a solid grasp of Python debugging tools, syntax error resolution, and efficient bug fixing in Python.
Types of Errors in Python
Errors in Python generally fall into three categories: Syntax Errors, Runtime Errors (Exceptions), and Logical Errors. Each type of error affects the execution of a program differently. Understanding these errors and knowing how to handle them effectively is crucial for writing reliable and efficient Python programs. Below, we explore each category in detail, including their common causes, examples, and methods to fix them.
1. Syntax Errors
A syntax error occurs when Python’s interpreter encounters incorrect syntax, making it unable to execute the code. Syntax errors often result from mistakes in code structure, punctuation, or indentation.
Common Causes of Syntax Errors:
- Missing colons (:) in function or loop definitions.
- Unmatched parentheses ( or {.
- Incorrect indentation, most important in Python.
- Using reserved keywords as variable names.
Example of a Syntax Error:
def greet()
print("Hello, World!")
Error Explanation: The missing colon (:) after greet() causes a SyntaxError, making the function definition incomplete.
How to Fix Syntax Errors
- Always ensure proper indentation in blocks of code.
- Use a Python syntax checker (such as an IDE’s built-in checker) before running the code.
- Carefully read error messages, as Python often points to the exact line where the issue exists.
2. Runtime Errors (Exceptions)
During Code execution runtime error occurs. If runtime error occurs then the normal flow of the program disrupts. Unlike syntax errors, runtime errors appear only after execution starts.
Common Types of Runtime Errors:
- ZeroDivisionError – This error occur when dividing by zero.
- NameError – Raised when a variable is not defined.
- TypeError – Occurs when an operation is applied to an inappropriate data type.
- IndexError – Happens when accessing an index that does not exist in a list.
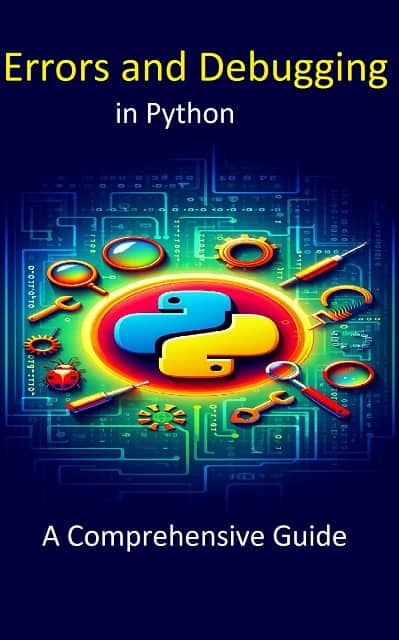
Example of a Runtime Error:
num = 10 / 0
Error Message: ZeroDivisionError: division by zero
How to Fix:
- Use exception handling in Python with try-except blocks.
- Validate inputs before performing operations.
Example of Fix:
try:
num = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero")
3. Logical Errors
Logical errors do not cause the program to crash, but they produce incorrect results. Since there are no error messages, they often go unnoticed until thorough testing or debugging is performed.
Example of a Logical Error:
def add_numbers(a, b):
return a - b # Incorrect operation
In this example, subtraction (-) is mistakenly used instead of addition (+). As a result, the function will return incorrect values, which can lead to inaccurate computations in the program.
How to Fix Logical Errors
- Use print() statements to track variable values at different points in the program.
- Utilize Python debugging tools like pdb or IDE debuggers to step through the code.
- Write unit tests using the unittest or pytest frameworks to verify expected outputs.
- Manually review the logic to ensure that operations and conditions align with the intended program logic.
Effective Debugging Techniques in Python
The process of identifying and solving errors called Debugging. Below some process explain to debug Python code easily.
1. Using Print Statements
This is the simplest technique to debug the python code. By inserting print() statements at different points in your program, you can track variable values, execution flow, and identify where errors occur.
x = 5
y = 10
print(f"x: {x}, y: {y}") # Helps track variable values
2. Leveraging Python Debugger (pdb)
For systematic, step-by-step debugging, Python provides the pdb (Python Debugger) module, which allows developers to pause program execution and inspect variables at specific points. This is particularly useful for identifying runtime errors, tracking variable changes, and understanding program flow.
Example of Using pdb:
import pdb
def divide(a, b):
pdb.set_trace() # Debugger starts here
return a / b
divide(10, 2)
Commands in pdb:
- n (next) – For Immediate next line Execution.
- p (print) – Print variable values.
- q (quit) – Exit the debugger.
3. Using IDE Debugging Tools
Most modern Python Integrated Development Environments (IDEs) like PyCharm, Visual Studio Code, and Jupyter Notebook come with built-in debugging tools that make error detection and resolution easier. These tools offer features such as breakpoints, variable inspection, step-over execution, call stack visualization, and real-time debugging, allowing developers to identify and fix issues efficiently.
4. Handling Exceptions with Logging
The logging module in Python provides a structured way to record errors, making debugging easier. Unlike print statements, logging allows you to store error messages in a log file, track issues over time, and analyze recurring errors. This is useful for large applications and production environments where monitoring errors is crucial.
import logging
logging.basicConfig(filename='error.log', level=logging.ERROR)
try:
result = 10 / 0
except ZeroDivisionError as e:
logging.error("Error: Division by zero")
5. Using Assertions
Assertions help catch logical errors early by ensuring certain conditions hold true while the program runs. They act as internal self-checks, preventing unexpected issues in later stages of execution. If an assertion fails, Python raises an AssertionError, stopping the program.
def square(n):
assert n >= 0, "Number must be non-negative"
return n * n
Using assertions is particularly helpful during debugging but should not replace proper error handling in production code.
Conclusion
Errors in Python are inevitable, but mastering error handling in Python, troubleshooting Python code, and efficient debugging strategies will help you write clean and error-free programs. By using debugging tools in Python, handling exceptions properly, and following best practices, you can ensure that your code runs smoothly and efficiently.
Learn More: Errors and Debugging in Python