Bayesian statistics is an essential branch of modern computational statistics, offering a robust framework for analyzing data, making predictions, and performing decision analysis. In this article, we explore key concepts and practical applications of Bayesian statistics in Python, focusing on topics such as computational statistics, estimation, odds and addends, decision analysis, prediction, approximate Bayesian computation, and hypothesis testing.
Unlike traditional methods, Bayesian approaches integrate prior knowledge with observed data, enabling dynamic updates to predictions as new information becomes available. Python, with its extensive libraries, is an excellent platform for implementing Bayesian methods.
What is Bayesian Statistics?
Bayesian statistics is a branch of statistics that focuses on updating beliefs or probabilities as new data becomes available. It operates on the principle of combining prior knowledge with observed evidence to refine predictions or estimates. This approach allows for dynamic adjustments in statistical models, making it particularly powerful for real-time decision-making and analysis. Bayesian methods are widely used in computational statistics, where they form the basis for many modern techniques in prediction, estimation, and hypothesis testing.
Core Topics in Bayesian Statistics
1. Computational Statistics
Bayesian statistics heavily relies on computational techniques, as closed-form solutions are often impractical for complex models. Python’s libraries, such as PyMC3 and TensorFlow Probability, leverage methods like Markov Chain Monte Carlo (MCMC) and Hamiltonian Monte Carlo (HMC) to approximate posterior distributions.
Example of Computational Statistics in Python:
import pymc3 as pm
import numpy as np
# Generate data
data = np.random.normal(0, 1, 100)
# Bayesian model
with pm.Model() as model:
mean = pm.Normal("mean", mu=0, sigma=1)
std = pm.HalfNormal("std", sigma=1)
likelihood = pm.Normal("obs", mu=mean, sigma=std, observed=data)
trace = pm.sample(1000)
pm.plot_posterior(trace)
2. Estimation
Bayesian estimation focuses on estimating parameters by integrating prior knowledge with observed data. Unlike point estimates in frequentist statistics, Bayesian methods provide a distribution for the parameter, reflecting uncertainty.
Example: Estimating Mean and Variance
with pm.Model() as model:
mu = pm.Normal("mu", mu=0, sigma=10)
sigma = pm.HalfNormal("sigma", sigma=10)
obs = pm.Normal("obs", mu=mu, sigma=sigma, observed=data)
trace = pm.sample(2000)
pm.summary(trace)
3. Odds and Addends
Odds ratios are pivotal in Bayesian statistics, particularly in fields like medicine and finance. They quantify the likelihood of one event relative to another.
Bayesian Odds:
The Bayesian framework allows us to compute the odds of different hypotheses dynamically as new data is introduced, offering greater flexibility compared to traditional methods.
4. Decision Analysis
Bayesian decision analysis enables rational decision-making under uncertainty by maximizing expected utility. Decisions are informed by the posterior distribution of outcomes.
Example: Decision Tree Analysis
In Python, decision analysis is often integrated with Bayesian methods to model the expected payoffs of different strategies. PyMC3 and custom utility functions can facilitate this process.
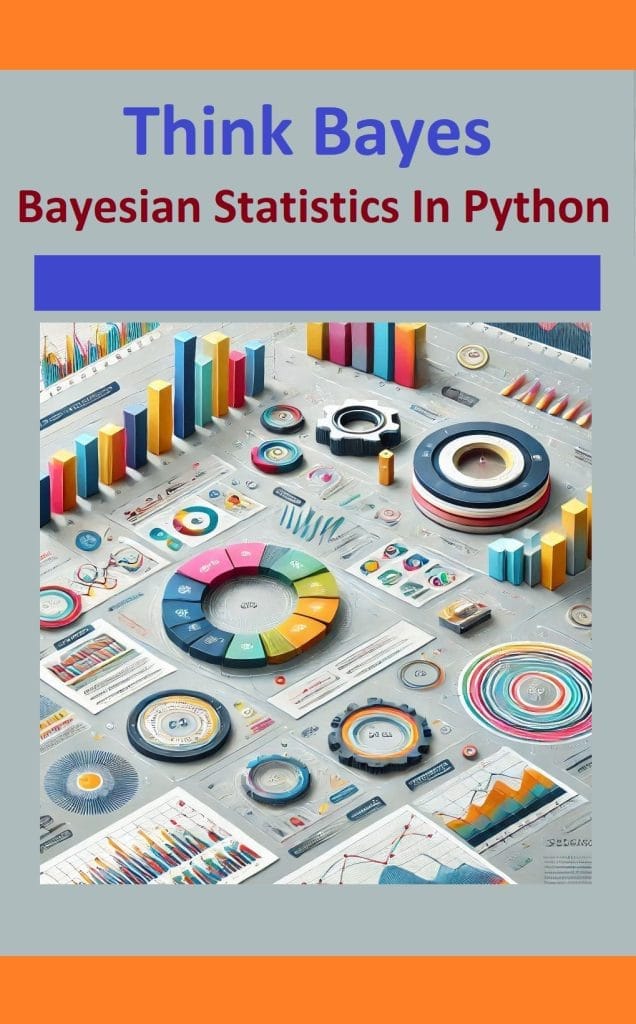
Download PDF: Think Bayes – Bayesian Statistics in Python
5. Prediction
Bayesian methods excel at prediction because they consider both the uncertainty in the data and the parameters. Probabilistic models generate predictions as distributions, providing richer insights than single-point estimates.
Example: Bayesian Predictive Modeling
with pm.Model() as model:
a = pm.Normal("a", mu=0, sigma=10)
b = pm.Normal("b", mu=0, sigma=10)
y_obs = pm.Normal("y_obs", mu=a + b * data, sigma=1, observed=data)
trace = pm.sample(1000)
predicted = pm.sample_posterior_predictive(trace, model=model)
print(predicted)
6. Approximate Bayesian Computation (ABC)
ABC is a technique for performing Bayesian inference when likelihood functions are computationally expensive or unavailable. It relies on simulating data from the prior and comparing it to observed data using distance metrics.
Example of ABC:
from abcpy.continuousmodels import Normal
from abcpy.inferences import ApproximateBayesianComputation
# Define the model
model = Normal(mean_prior=(0, 1), std_prior=(1, 1))
# Perform inference
abc = ApproximateBayesianComputation(model, observed_data=data, distance_function='euclidean')
result = abc.infer()
print(result)
7. Hypothesis Testing
Bayesian hypothesis testing evaluates the probability of a hypothesis given the data, contrasting with frequentist approaches that focus on p-values.
Example: Bayesian A/B Testing
import pymc3 as pm
import numpy as np
# Simulated data
group_a = np.random.binomial(1, 0.4, 100)
group_b = np.random.binomial(1, 0.5, 100)
# Define Bayesian model
with pm.Model() as model:
p_a = pm.Beta("p_a", alpha=1, beta=1)
p_b = pm.Beta("p_b", alpha=1, beta=1)
obs_a = pm.Binomial("obs_a", n=1, p=p_a, observed=group_a)
obs_b = pm.Binomial("obs_b", n=1, p=p_b, observed=group_b)
trace = pm.sample(2000)
pm.plot_posterior(trace, var_names=["p_a", "p_b"], ref_val=0)
Conclusion
Bayesian statistics, with its ability to incorporate uncertainty and prior knowledge, is revolutionizing computational statistics, decision analysis, and hypothesis testing. Python’s powerful libraries make these methods accessible, enabling robust and dynamic statistical modeling across industries.