Python has revolutionized the way we approach statistical analysis by providing powerful tools and libraries that simplify data manipulation, visualization, and modeling. With its ease of use and extensive functionality, Python is an indispensable tool for statisticians, data scientists, and analysts. This article explores statistics with Python, focusing on libraries such as pandas, statsmodels, and seaborn, and delving into key concepts such as data display, probability distributions, hypothesis testing, and statistical modeling.
Pandas: Data Structures for Statistics
At the core of statistical analysis lies efficient data management. The pandas library provides flexible and intuitive data structures, such as Series and DataFrames, which are ideal for organizing and analyzing data. These structures enable users to manipulate and explore datasets efficiently, making them fundamental for statistical tasks.
Key Features of pandas for Statistical Analysis
- Series and DataFrames:
- Series: One-dimensional arrays capable of holding any data type, such as integers, floats, or strings. They include labels (index) for each element, enabling intuitive data slicing and indexing.
- DataFrame: A two-dimensional labeled data structure resembling a spreadsheet, with rows and columns. Columns can hold data of varying types, making it perfect for real-world datasets.
- Data Cleaning and Manipulation:
pandas simplifies operations like filtering rows, imputing missing values, renaming columns, and reshaping data, saving time during preprocessing. - Descriptive Statistics:
pandas includes methods like .mean(), .std(), and .describe() to compute summary statistics, offering insights into data distributions.
Example
import pandas as pd
data = {'Age': [25, 30, 35, 40], 'Salary': [50000, 60000, 70000, 80000]}
df = pd.DataFrame(data)
# Descriptive Statistics
print(df.describe())
The df.describe() function provides key metrics like count, mean, standard deviation, min, and max for numerical columns.
Statsmodels: Tools for Statistical Modeling
The statsmodels library is a robust Python package tailored for statistical modeling, hypothesis testing, and data exploration. It provides a comprehensive suite of statistical tools, allowing users to build and validate models while performing diagnostic checks. Its flexibility and functionality make it an essential library for tasks like regression analysis, ANOVA, and time-series forecasting.
Key Features of statsmodels
- Linear and Nonlinear Regression:
Statsmodels supports models like Ordinary Least Squares (OLS) for simple linear regression and Generalized Linear Models (GLM) for more complex relationships. These tools are critical for understanding and predicting relationships between variables. - Hypothesis Testing:
Includes statistical tests such as t-tests for comparing means, ANOVA for group comparisons, and likelihood ratio tests to evaluate model fit. - Time Series Analysis:
Statsmodels offers tools for decomposition, ARIMA modeling, and seasonal adjustments, making it ideal for analyzing temporal data trends and forecasting.
Example: Linear Regression with statsmodels
import statsmodels.api as sm
X = [1, 2, 3, 4]
y = [2, 4, 6, 8]
X = sm.add_constant(X) # Adding a constant term for the intercept
model = sm.OLS(y, X).fit()
print(model.summary())
This example showcases how simple it is to fit a regression model, evaluate coefficients, and interpret results using statsmodels.
Seaborn: Data Visualization
Visualizing data is critical for understanding and presenting statistical findings. Seaborn, a library built on top of Matplotlib, simplifies the creation of visually appealing and insightful graphics. It offers a high-level interface for drawing attractive and informative statistical plots, which are essential for identifying trends, patterns, and anomalies in datasets.
Key Features of Seaborn
- Distribution Plots:
Tools like histograms, box plots, and violin plots are used to understand data spread, central tendency, and outliers. These plots are invaluable for assessing the distribution of data at a glance. - Relational Plots:
Scatter plots and line plots enable the analysis of relationships and trends between two or more variables, offering insights into correlations and dependencies. - Heatmaps:
Heatmaps provide a visual representation of data density and correlations. They are particularly useful for exploring large datasets with multiple variables.
By combining simplicity and functionality, Seaborn enhances data visualization in Python, making it indispensable for statistical analysis.
Example: Visualization of Data Distribution
import seaborn as sns
import matplotlib.pyplot as plt
data = [5, 10, 15, 20, 25]
sns.histplot(data, kde=True)
plt.title("Distribution Plot")
plt.show()
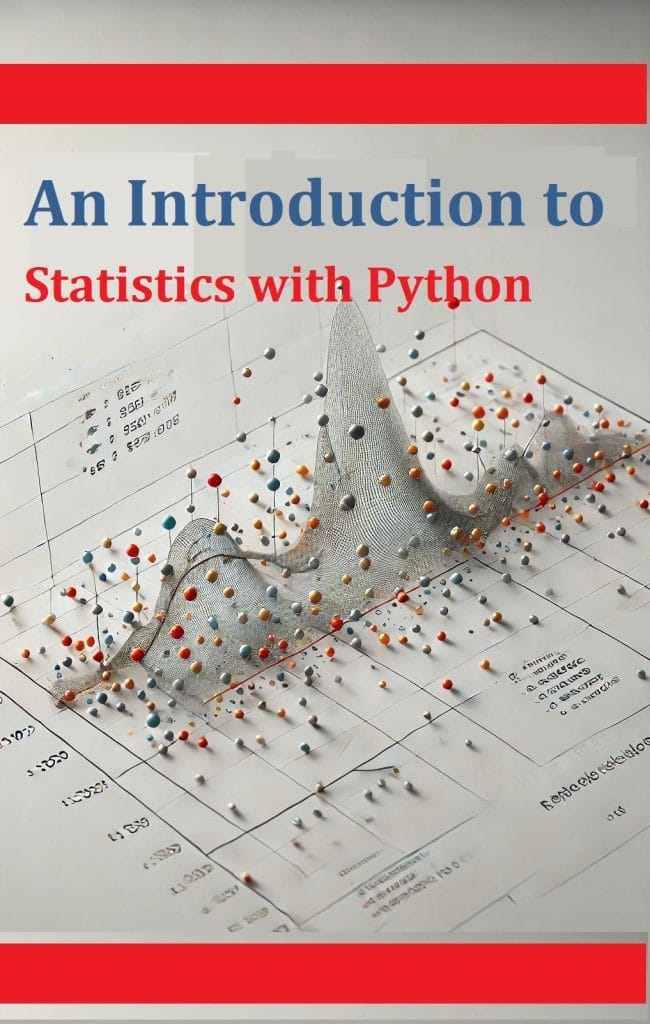
Display of Statistical Data
Data types in Python
Understanding data types is fundamental for statistical analysis, as it determines the operations and analyses applicable to a dataset. Python supports a variety of data types, making it versatile for statistical tasks:
- Numerical Data: Includes integers (int) and floating-point numbers (float). These types are used for calculations, summaries, and regression analysis.
- Categorical Data: Comprises string values or predefined categories. Commonly used in segmentation, grouping, and chi-square tests.
- Boolean Data: Represents binary states (True/False), often used in logical operations and decision-making processes.
Plotting and Displaying Statistical Datasets
Data visualization is a powerful tool for revealing patterns, trends, and anomalies in a dataset. Python’s libraries, such as Matplotlib and Seaborn, offer intuitive and customizable plotting options:
- Bar Charts: Display frequencies or proportions of categorical data.
- Line Graphs: Illustrate trends over time or continuous variables.
- Box Plots: Highlight data spread, central tendency, and outliers.
These visualizations aid in communicating insights effectively.
Example: Box Plot in Seaborn
import seaborn as sns
data = {'Category': ['A', 'A', 'B', 'B'], 'Values': [10, 20, 15, 25]}
df = pd.DataFrame(data)
sns.boxplot(x='Category', y='Values', data=df)
plt.title("Box Plot Example")
plt.show()
Distribution and Hypothesis Tests
Statistical analysis relies heavily on understanding distributions and testing hypotheses to draw meaningful conclusions about data. This section covers key concepts such as populations and samples, probability distributions, and hypothesis testing.
1. Population and Samples
In statistics, a population refers to the entire group of individuals or items that are the subject of a study. For instance, if you are studying the heights of adults in a city, the population would include all adults in that city. However, analyzing an entire population is often impractical due to constraints like time, cost, or accessibility.
Instead, researchers use a sample, which is a subset of the population, to infer characteristics of the entire group. A well-chosen sample should represent the population accurately, minimizing bias and ensuring reliable results. Sampling techniques such as random sampling, stratified sampling, and systematic sampling are critical in this process.
2. Probability Distributions
Probability distributions describe how data is distributed across different values.
- Normal Distribution: A bell-shaped curve that represents data symmetrically around the mean. It is commonly used in natural and social sciences to model real-world variables.
- Binomial Distribution: Used for experiments with two possible outcomes, such as success or failure.
Example of Normal Distribution in Python:
import numpy as np
import matplotlib.pyplot as plt
data = np.random.normal(loc=0, scale=1, size=1000) # Mean=0, Std=1
plt.hist(data, bins=30, density=True)
plt.title("Normal Distribution")
plt.show()
3. Hypothesis Testing
Hypothesis testing is a statistical method used to evaluate assumptions about a dataset.
- Null Hypothesis (H₀): Proposes no significant effect or difference in the data.
- Alternative Hypothesis (H₁): Suggests a significant effect or difference.
A key element in hypothesis testing is the degree of freedom (df), which refers to the number of independent values in a dataset that can vary without affecting the overall outcome. The results of hypothesis tests, such as t-tests or chi-square tests, help determine if the null hypothesis should be rejected in favor of the alternative.
Example of a t-Test in Python:
from scipy.stats import ttest_1samp
data = [10, 12, 14, 16, 18]
t_stat, p_value = ttest_1samp(data, 15)
print(f"T-statistic: {t_stat}, P-value: {p_value}")
If the p-value is below a predefined threshold (commonly 0.05), the null hypothesis is rejected, indicating a statistically significant result.
Understanding these concepts is essential for conducting robust statistical analyses.
Statistical Modeling
Statistical modeling refers to the process of using mathematical formulas to represent and analyze relationships between variables in a dataset. This approach helps uncover patterns, make predictions, and test hypotheses. Below are four key techniques commonly used in statistical modeling:
1. Linear Regression Models
Linear regression is a foundational technique for modeling the relationship between one dependent variable (target) and one or more independent variables (predictors). The simplest form, simple linear regression, assumes a linear relationship between the two variables. The model fits a line to the data that minimizes the difference between observed and predicted values.
Linear regression is widely used in applications such as sales forecasting, trend analysis, and risk assessment. It provides interpretable results, such as coefficients that indicate how much the dependent variable changes for a unit increase in the independent variable.
Example
from sklearn.linear_model import LinearRegression
X = [[1], [2], [3], [4]]
y = [2, 4, 6, 8]
model = LinearRegression()
model.fit(X, y)
print(f"Coefficient: {model.coef_[0]}, Intercept: {model.intercept_}")
2. Multivariate Data Analysis
Multivariate data analysis (MDA) is a powerful statistical technique that enables the examination of multiple variables at once to uncover relationships, dependencies, and patterns in complex datasets. Unlike univariate or bivariate analysis, which focuses on single or pairwise variables, MDA accounts for interactions between several variables simultaneously, making it ideal for high-dimensional data.
Key techniques in MDA include:
- Principal Component Analysis (PCA): PCA is used for dimensionality reduction by transforming correlated variables into a smaller set of uncorrelated variables called principal components. These components capture the maximum variance in the data, allowing analysts to visualize and interpret large datasets more effectively.
- Factor Analysis: Similar to PCA, factor analysis seeks to identify underlying factors or latent variables that explain the correlations among observed variables. This technique is commonly used in psychology, market research, and other fields to uncover hidden patterns and reduce noise in data.
- Multivariate Regression: This method extends linear regression to multiple dependent variables, allowing analysts to model relationships between several independent and dependent variables simultaneously. It is particularly useful in fields like economics, health sciences, and social sciences.
By applying MDA techniques, researchers can detect clusters, identify patterns, and explain variability in large datasets, which is especially valuable in fields like marketing, genomics, and economics. Multivariate methods enhance the ability to make informed predictions, find structure in complex data, and guide decision-making based on multiple influencing factors.
3. Tests on Discrete Data
Tests on discrete data, like the chi-square test, evaluate the relationship between categorical variables. This is essential in scenarios such as market research or clinical trials, where associations between factors are critical.
Example
from scipy.stats import chi2_contingency
data = [[10, 20], [20, 40]]
chi2, p, dof, expected = chi2_contingency(data)
print(f"Chi-square: {chi2}, P-value: {p}")
4. Bayesian Statistics
Bayesian statistics incorporates prior knowledge or beliefs into the analysis, updating them as new evidence emerges. Unlike traditional frequentist methods, Bayesian approaches provide a probabilistic interpretation, making them particularly useful in uncertain or dynamic environments. Bayesian techniques are frequently applied in fields like machine learning, finance, and medicine.
Conclusion
Python is an essential tool for statistical analysis, offering libraries like pandas, statsmodels, and seaborn to streamline tasks ranging from data manipulation to visualization and modeling. By mastering Python for statistical techniques such as hypothesis testing, probability distributions, and linear regression, analysts can uncover actionable insights and drive informed decisions.