In the fast-paced world of web development, creating robust, scalable, and secure applications is a necessity. Python, known for its simplicity and power, has been a favorite among developers for years. With the release of Django 5, the framework continues to empower developers to build powerful python web applications with minimal hassle. In this article, we will delve into how you can use Django 5 to create powerful and reliable Python web applications from scratch, with practical examples and high-value insights.
Why Django 5?
Django 5 builds upon the solid foundation of its predecessors, offering new features that streamline the development process. It’s known for its “batteries-included” philosophy, providing everything you need out of the box—ranging from an ORM (Object-Relational Mapper) to an admin interface and built-in security features. With Django 5, developers can quickly build web applications that are not only functional but also secure, scalable, and easy to maintain.
Getting Started with Django 5
1. Setting Up Your Development Environment
Before diving into development, setting up your environment correctly is crucial. You’ll need Python installed, along with virtual environments to manage your dependencies. Here’s a quick setup guide:
- Install Python: Ensure Python 3.9 or later is installed.
- Create a Virtual Environment: Use python -m venv env to create a virtual environment.
- Install Django 5: Activate your environment and run pip install django to get Django 5 up and running.
2. Creating Your First Django Project
Starting a new Django project is straightforward. Use the following command:
django-admin startproject mysite
This command will create a new directory with the necessary files to get your project started. Within this directory, you’ll find files like settings.py (for configuration), urls.py (for routing), and manage.py (a command-line utility for various tasks).
3. Building a Simple Web Application
One of Django’s greatest strengths is its ability to quickly transform an idea into a functional web application. To demonstrate this, we’ll build a basic blog application that highlights Django’s structure and workflow.
Step 1: Create a New App
Django projects are composed of multiple apps, which are modular and reusable components. To create a new app named blog
, run the following command:
python manage.py startapp blog
This command generates a new directory with essential files, such as models.py
, views.py
, and admin.py
, which define the app’s functionality. Apps in Django are modular and reusable, making them perfect for larger projects.
Step 2: Define the Database Models
The models.py
file contains the database structure. Let’s define a simple Post
model for our blog:
from django.db import models
class Post(models.Model):
title = models.CharField(max_length=100)
content = models.TextField()
date_posted = models.DateTimeField(auto_now_add=True)
def __str__(self):
return self.title
Here, we define three fields:
title
– A short text field (maximum 100 characters).content
– A longer text field for blog content.date_posted
– Automatically stores the date and time when the post is created.
Step 3: Migrate the Database
After defining the model, apply the changes to the database using:
python manage.py makemigrations
python manage.py migrate
This will generate the necessary tables in the database, preparing the app to store blog posts efficiently.
4. Django 5’s Advanced Features
Django 5 introduces several powerful features that enhance performance, security, and scalability, making it a top choice for modern web development. Let’s dive into three key improvements: Asynchronous Views, an Improved ORM, and Enhanced Security Features.
1. Asynchronous Views
A major feature in Django 5 is the support for asynchronous views, which allows for non-blocking I/O operations. Traditional Django views are synchronous, meaning the server can handle only one request at a time per thread. However, with asynchronous views using async def
, multiple requests can be processed concurrently, leading to better performance for applications involving I/O-bound tasks like API calls or file processing.
This is particularly useful for real-time applications, high-traffic websites, and those dealing with numerous external data sources. Instead of being stalled by slow operations, asynchronous views let Django applications manage more requests simultaneously, improving responsiveness and scalability.
For example, if your application makes multiple external API calls or needs to perform long-running tasks like processing uploaded files, asynchronous views can prevent these operations from blocking other requests, keeping the app smooth and efficient.
async def async_view(request):
await some_async_task()
return JsonResponse({'status': 'Completed'})
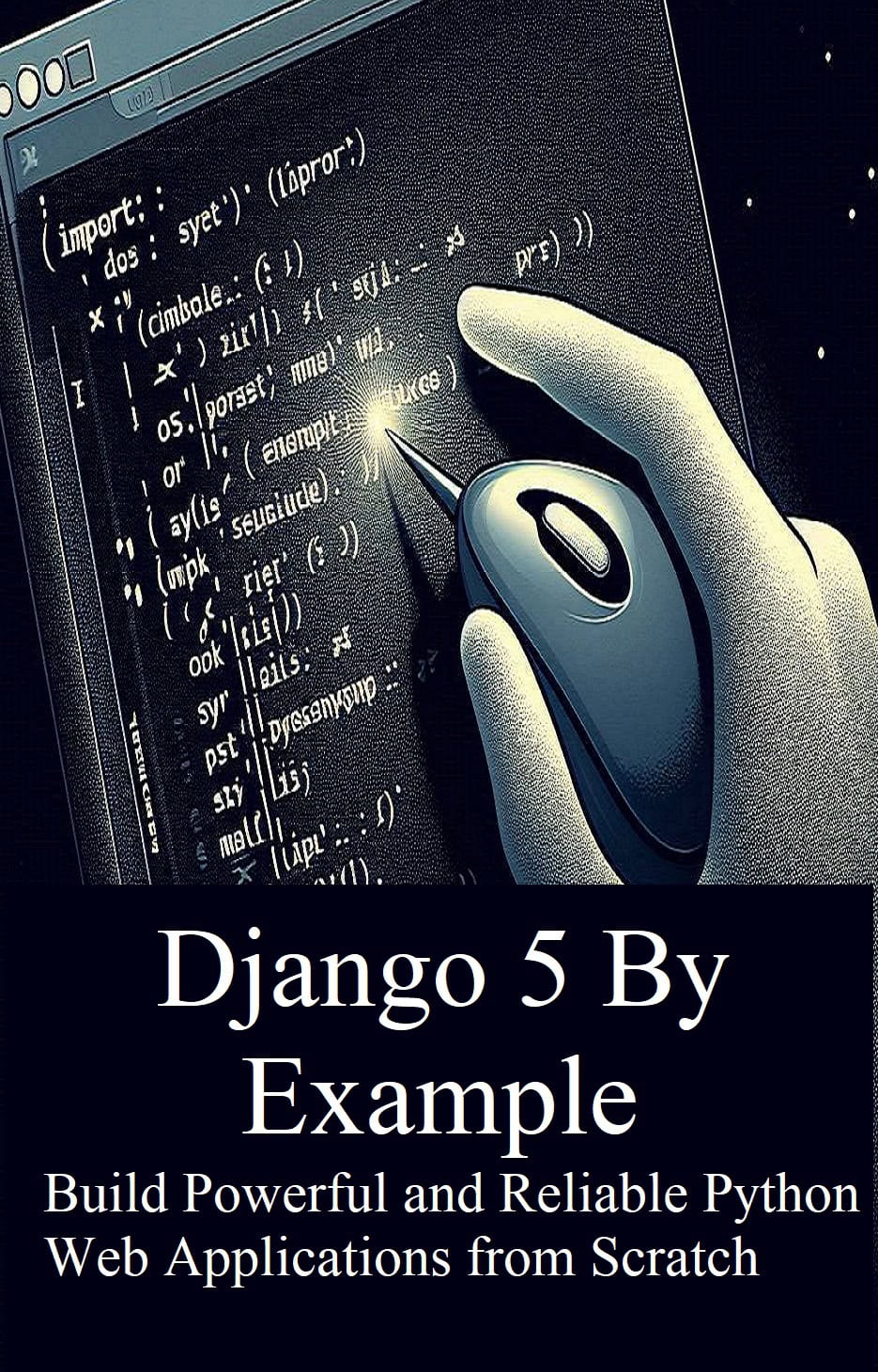
2. Improved ORM
Django 5’s Object-Relational Mapper (ORM) has received substantial improvements, making it easier and faster to interact with the database. The enhancements focus on simplifying complex queries while boosting performance. This allows developers to write more efficient database operations without needing to rely on raw SQL, making applications more maintainable and scalable.
The improved ORM supports complex features like window functions, subqueries, and advanced expressions, making it easier to execute powerful database queries without leaving the Django framework. Whether retrieving data or performing updates, the enhanced ORM helps optimize database interactions, leading to faster queries and more efficient data management.
For example, an advanced query involving multiple conditions and joins can now be handled more efficiently, making Django 5 well-suited for data-heavy applications like e-commerce, analytics, or social media platforms.
recent_orders = Order.objects.filter(status='completed').annotate(total_price=F('quantity') * F('product__price'))
3. Enhanced Security Features
Django has always prioritized security, and Django 5 strengthens its security features even further. It includes protections against common vulnerabilities like SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). In Django 5, password management has been enhanced with stronger hashing algorithms and policies, ensuring better protection for user credentials.
Django 5 also improves authentication mechanisms, supporting modern workflows like multi-factor authentication (MFA) and OAuth with ease. Additionally, the security middleware has been updated to enforce stricter HTTP security headers, such as Content Security Policy (CSP) and Strict-Transport-Security (HSTS), which mitigate common attacks and enforce HTTPS connections.
For applications that handle sensitive data, such as financial or healthcare platforms, these features are critical. Django 5’s built-in security tools provide peace of mind, allowing developers to focus on building their apps while the framework handles many essential security tasks.
5. Deploying Your Django Application
Once your Django application is complete, deploying it is the next crucial step. Django 5 offers flexibility for deployment across various platforms, including traditional VPS setups and cloud-based solutions like AWS, Heroku, and Google Cloud Platform. Let’s explore the essentials of deploying your Django application.
1. Choosing a Hosting Platform
Django 5 supports deployment on both traditional VPS setups and modern cloud-based solutions.
Traditional VPS: Platforms like DigitalOcean or Linode provide full control over your server. This requires hands-on management of server security, scalability, and maintenance.
Cloud Platforms: AWS, Heroku, and Google Cloud Platform are ideal for developers who need scalability and automated infrastructure management. Heroku is especially popular for its simplicity, allowing you to deploy applications with a few commands. AWS and Google Cloud, on the other hand, offer more extensive services for larger applications.
2. Using Gunicorn for Deployment
For handling HTTP requests in production, Gunicorn (Green Unicorn) is a highly recommended WSGI server for Python applications.
To install Gunicorn, run:
pip install gunicorn
After installation, you can start Gunicorn with:
gunicorn myproject.wsgi:application
Gunicorn is often used in conjunction with Nginx, a reverse proxy server, which handles static files and provides additional security.
# Example Nginx configuration
server {
listen 80;
server_name yourdomain.com;
location / {
proxy_pass http://127.0.0.1:8000;
}
}
Gunicorn can also be managed by tools like systemd or supervisord to ensure it runs continuously and restarts if needed.
3. Database Management
For production, switching from SQLite to a robust database system is recommended. Django 5 supports PostgreSQL, MySQL, and other popular databases.
PostgreSQL is the preferred choice due to its performance, scalability, and advanced features that work well with Django’s ORM.
MySQL is also widely used for high-traffic applications requiring reliability and performance.
Once your production database is ready, run migrations to apply your application’s database schema:
python manage.py migrate
Make sure the database is optimized for performance (e.g., indexing for faster queries) and secured with proper credentials and encryption.
4. Monitoring and Performance Optimization
Once your Django application is live, it’s important to continuously monitor its performance.
New Relic provides in-depth insights into response times, server performance, and database queries. It allows you to monitor application health and set performance alerts.
Django Debug Toolbar is useful for identifying performance bottlenecks during development.
Caching: Implementing caching strategies like Memcached or Redis helps reduce the load on your database by storing frequently accessed data in memory, significantly improving performance.
5. Security Considerations
HTTPS: Secure your site by serving it over HTTPS. Obtain an SSL certificate through services like Let’s Encrypt to encrypt data between the server and users.
Environment Variables: Use environment variables to store sensitive information like API keys and database credentials securely.
Static and Media Files: In production, serve static files through Nginx or cloud storage services like Amazon S3 to handle large-scale traffic.
Conclusion
Django 5 by Example: Building Powerful and Reliable Python Web Applications from Scratch is more than just a framework—it’s a powerful tool that can take your web development skills to the next level. By mastering Django 5, you can design, develop, and deploy applications that are not only powerful but also secure and scalable. The new features in Django 5 make it easier than ever to create web applications that meet the demands of modern users.