The evolution of machine learning (ML) has transformed industries, providing solutions to problems that were previously unsolvable. Whether it’s automating workflows, enhancing customer experiences, or solving complex analytical challenges, machine learning is a driving force behind modern technological innovation.
This article will explore a hands on machine learning with Scikit Learn, key techniques like classification, support vector machines, decision trees, ensemble learning, and advanced topics like dimensionality reduction and training deep neural networks.
The Machine Learning Landscape
Machine learning can be categorized into three primary types based on the learning paradigm:
- Supervised Learning: Involves training models on labeled datasets to perform tasks like classification and regression.
- Unsupervised Learning: Focuses on discovering patterns in unlabeled data, often using clustering or dimensionality reduction techniques.
- Reinforcement Learning: Involves training agents to make sequential decisions by maximizing rewards from interactions with an environment.
These categories encompass diverse tasks, from predicting outcomes and classifying data to discovering hidden patterns in complex datasets.
Classification: A Key Machine Learning Task
Classification is one of the most common machine learning tasks, where the objective is to assign data points to predefined categories. For instance, email spam detection classifies emails as “spam” or “not spam.”
Example Using Scikit-Learn:
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import classification_report
# Load dataset
data = load_iris()
X, y = data.data, data.target
# Train-test split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Train classifier
clf = RandomForestClassifier(random_state=42)
clf.fit(X_train, y_train)
# Evaluate
y_pred = clf.predict(X_test)
print(classification_report(y_test, y_pred))
Training Models
Training a model involves feeding data into a learning algorithm and iteratively improving its parameters to minimize error. In traditional machine learning, models like linear regression or logistic regression are frequently used, while deep learning focuses on artificial neural networks.
Important Considerations During Training:
- Overfitting: When the model performs well on training data but poorly on unseen data.
- Underfitting: When the model is too simple to capture the underlying patterns in data.
- Cross-Validation: Splitting data into multiple folds to ensure robust evaluation.
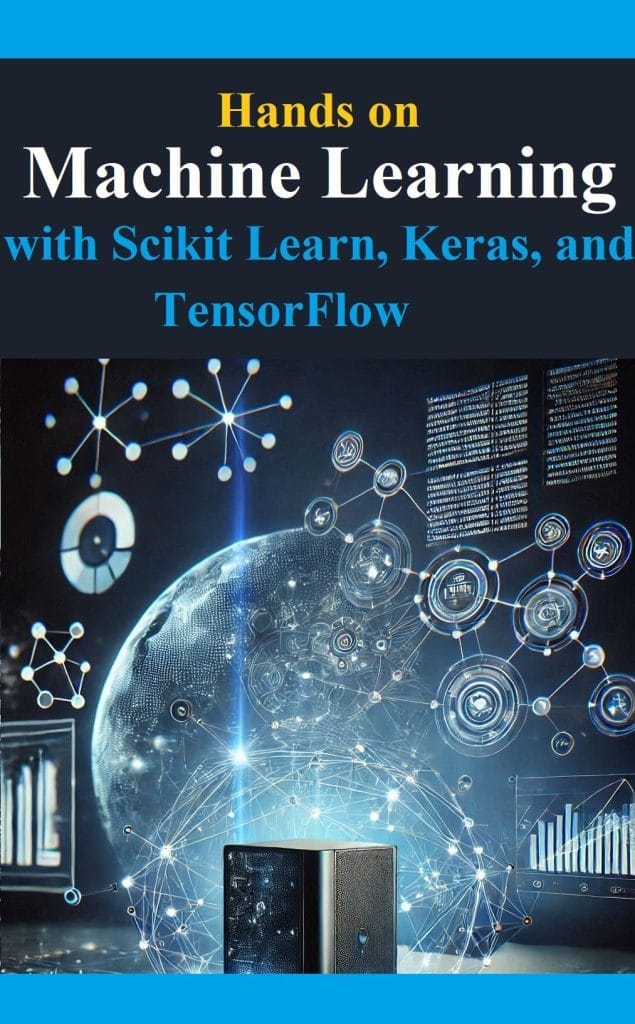
Support Vector Machines (SVMs)
Support Vector Machines are powerful supervised learning algorithms used for both classification and regression tasks. They work by finding the hyperplane that best separates classes in the feature space.
SVM Example with Scikit-Learn:
from sklearn.svm import SVC
# Train an SVM
svm_model = SVC(kernel='linear', random_state=42)
svm_model.fit(X_train, y_train)
# Predict and evaluate
y_pred_svm = svm_model.predict(X_test)
print(classification_report(y_test, y_pred_svm))
Decision Trees
Decision Trees are interpretable models that split data into subsets based on feature values. Each node represents a decision rule, making them ideal for non-linear data.
Decision Tree Example Using Scikit-Learn:
from sklearn.tree import DecisionTreeClassifier
# Train a decision tree
dt_model = DecisionTreeClassifier(max_depth=5, random_state=42)
dt_model.fit(X_train, y_train)
# Evaluate
y_pred_dt = dt_model.predict(X_test)
print(classification_report(y_test, y_pred_dt))
While decision trees are prone to overfitting, they form the basis for more robust ensemble methods like Random Forests.
Ensemble Learning and Random Forests
Ensemble learning combines multiple models to improve performance. Among ensemble methods, Random Forests are particularly popular for their ability to reduce overfitting by aggregating predictions from multiple decision trees. Random Forests are versatile and often outperform single decision trees on complex datasets.
Random Forest Example:
from sklearn.ensemble import RandomForestClassifier
# Train a random forest
rf_model = RandomForestClassifier(n_estimators=100, random_state=42)
rf_model.fit(X_train, y_train)
# Evaluate
y_pred_rf = rf_model.predict(X_test)
print(classification_report(y_test, y_pred_rf))
Dimensionality Reduction
In high-dimensional datasets, reducing the number of features can improve model performance and reduce computational complexity. Techniques like Principal Component Analysis (PCA) and t-SNE are commonly used. Dimensionality reduction not only improves model efficiency but also aids in uncovering hidden patterns in the data.
PCA Example:
from sklearn.decomposition import PCA
# Apply PCA
pca = PCA(n_components=2)
X_reduced = pca.fit_transform(X)
print(f"Explained variance ratio: {pca.explained_variance_ratio_}")
Introduction to Artificial Neural Networks
Artificial Neural Networks (ANNs) mimic the human brain’s structure and are the foundation of deep learning. ANNs consist of layers of interconnected nodes (neurons), where each node applies a mathematical operation to its inputs.
Keras ANN Example:
from keras.models import Sequential
from keras.layers import Dense
# Build a simple ANN
model = Sequential()
model.add(Dense(64, activation='relu', input_dim=4))
model.add(Dense(3, activation='softmax'))
# Compile model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Summary
model.summary()
Keras makes it simple to design, train, and deploy neural networks.
Training Deep Neural Nets
Training deep neural networks is more complex than traditional ML models, requiring techniques like:
- Dropout: Prevents overfitting by randomly disabling neurons during training.
- Batch Normalization: Normalizes layer outputs to accelerate training.
- Learning Rate Scheduling: Dynamically adjusts the learning rate during training.
Example Using TensorFlow:
import tensorflow as tf
from tensorflow.keras import layers
# Define a deep neural network
model = tf.keras.Sequential([
layers.Dense(128, activation='relu', input_shape=(4,)),
layers.Dense(64, activation='relu'),
layers.Dense(3, activation='softmax')
])
# Compile model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Summary
model.summary()
Deep neural networks are ideal for tasks like image recognition, natural language processing, and time-series forecasting.
Conclusion
The machine learning landscape offers a wide array of techniques and tools to address diverse challenges. From foundational tasks like classification and dimensionality reduction to advanced methods like training deep neural networks, frameworks like Scikit-Learn, Keras, and TensorFlow simplify the development process.
By mastering key concepts like ensemble learning, dimensionality reduction, and artificial neural networks, you can unlock the true potential of machine learning to tackle real-world problems effectively.