Python has become the cornerstone of data science due to its simplicity and powerful ecosystem of libraries. These tools empower data scientists to perform a wide range of tasks, from data manipulation and visualization to advanced machine learning.
This article serves as a Python data science handbook for working with data, focusing on the critical components: NumPy, Pandas, Matplotlib, and Scikit-learn. Whether you’re a beginner or an experienced professional, this comprehensive guide will deepen your understanding of Python’s capabilities in data science.
Essential Skills for Python Data Scientists
Beyond mastering libraries, data scientists need a robust understanding of specific skills and techniques.
1. Data Cleaning and Preprocessing
Raw data is often messy and incomplete. Learning to clean and preprocess data is fundamental. This includes handling missing values, normalizing data, and encoding categorical variables.
2. Exploratory Data Analysis (EDA)
EDA involves summarizing the main characteristics of a dataset. Techniques like visualization and statistical analysis help uncover patterns, trends, and anomalies.
3. Feature Engineering
Feature engineering transforms raw data into meaningful inputs for machine learning models. This involves techniques like scaling, encoding, and dimensionality reduction.
4. Machine Learning and Model Evaluation
Understanding machine learning algorithms and how to evaluate their performance is crucial. Metrics like accuracy, precision, recall, and F1 score are commonly used.
5. Big Data and Cloud Integration
Working with large-scale data often requires tools like Apache Spark or cloud platforms like AWS and Google Cloud. Python libraries such as PySpark enable seamless integration.
Introduction to NumPy
NumPy, short for Numerical Python, is the foundation of scientific computing in Python. It provides an efficient way to handle numerical data and perform mathematical operations.
Key Features of NumPy
- N-Dimensional Arrays: NumPy introduces the ndarray, a powerful data structure that supports multi-dimensional arrays.
- Mathematical Functions: From basic arithmetic to advanced operations like linear algebra and Fourier transforms, NumPy simplifies complex calculations.
- Broadcasting: Allows element-wise operations on arrays of different shapes, eliminating the need for explicit loops.
- Integration: Serves as a backbone for other libraries like Pandas, Scikit-learn, and TensorFlow.
Example: Creating and Manipulating Arrays
import numpy as np
# Creating an array
arr = np.array([1, 2, 3, 4])
# Performing mathematical operations
arr_squared = arr ** 2
print(arr_squared) # Output: [1 4 9 16]
NumPy is particularly useful for tasks like handling large datasets, performing simulations, and supporting high-speed computations in machine learning workflows.
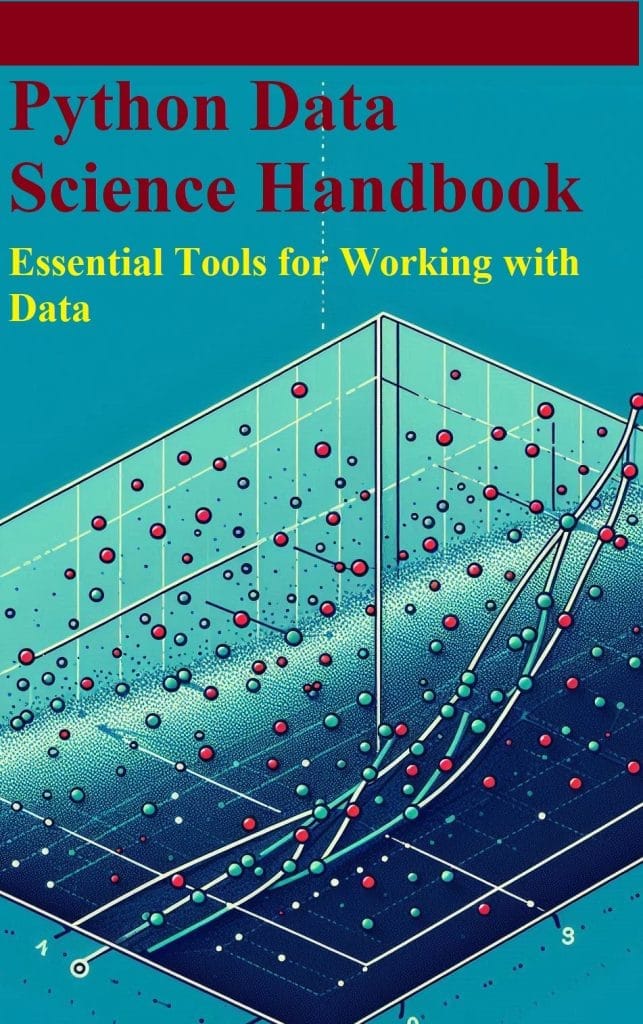
Download PDF: Python Data Science Handbook
Data Manipulation with Pandas
Pandas is an essential library for data manipulation and analysis. It introduces data structures like Series and DataFrames, making it easy to clean, transform, and analyze datasets.
Key Features of Pandas
- Data Cleaning: Handle missing or inconsistent data with functions like fillna() and dropna().
- Data Transformation: Tools for reshaping, merging, and aggregating data.
- Integration with Other Formats: Load and save data in formats like CSV, Excel, SQL, and JSON.
Example: Basic DataFrame Operations
import pandas as pd
# Creating a DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35]}
df = pd.DataFrame(data)
# Adding a new column
df['Age in 5 Years'] = df['Age'] + 5
print(df)
Pandas is invaluable for tasks like exploratory data analysis (EDA) and preprocessing data before applying machine learning models.
Visualization with Matplotlib
Visualization is a critical step in data analysis. Matplotlib, a versatile library, allows you to create static, animated, and interactive plots.
Key Features of Matplotlib
- Wide Range of Plot Types: Line, scatter, bar, histogram, and pie charts.
- Customization: Control over colors, labels, axes, and legends.
- Integration with Pandas: Plot directly from DataFrames for streamlined workflows.
Example: Creating a Line Plot
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4]
y = [10, 20, 30, 40]
# Creating a line plot
plt.plot(x, y, marker='o')
plt.title('Sample Line Plot')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.show()
Matplotlib’s flexibility makes it ideal for creating professional-quality visualizations to communicate insights effectively.
Machine Learning with Python
Machine learning is at the core of modern data science. It involves using algorithms to make predictions or uncover patterns in data. Python provides extensive support for machine learning through libraries like Scikit-learn.
Categories of Machine Learning
- Supervised Learning: Models are trained on labeled data (e.g., regression, classification).
- Unsupervised Learning: Algorithms uncover patterns in unlabeled data (e.g., clustering, dimensionality reduction).
- Reinforcement Learning: Agents learn optimal actions through trial and error in dynamic environments.
Introducing Scikit-learn
Scikit-learn is a comprehensive library that simplifies the implementation of machine learning algorithms.
Key Features of Scikit-learn
- A wide range of algorithms (e.g., linear regression, decision trees, support vector machines).
- Tools for preprocessing data, such as scaling and encoding.
- Methods for evaluating and tuning models.
Example: Training a Model in Scikit-learn
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import train_test_split
# Sample data
X = [[1], [2], [3], [4]]
y = [10, 20, 30, 40]
# Splitting data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
# Training a model
model = LinearRegression()
model.fit(X_train, y_train)
print("Model Coefficients:", model.coef_)
Scikit-learn’s simplicity and versatility make it a favorite among data scientists for building and deploying machine learning models.
Hyperparameters and Model Validation
Model performance depends on selecting the right hyperparameters, such as learning rates or the number of decision tree splits. Scikit-learn provides tools for hyperparameter tuning and validation.
Example: Grid Search for Hyperparameter Tuning
from sklearn.model_selection import GridSearchCV
# Defining a parameter grid
param_grid = {'fit_intercept': [True, False]}
grid = GridSearchCV(estimator=LinearRegression(), param_grid=param_grid, cv=3)
# Fitting the grid search
grid.fit(X_train, y_train)
print("Best Parameters:", grid.best_params_)
Hyperparameter tuning ensures models are optimized for accuracy without overfitting.
Feature Engineering
Feature engineering involves transforming raw data into meaningful features that improve model performance. Common techniques include:
- Scaling: Standardizing data ranges using libraries like StandardScaler from Scikit-learn.
- Encoding: Converting categorical data into numerical formats using one-hot encoding or label encoding.
- Dimensionality Reduction: Techniques like PCA (Principal Component Analysis) to simplify datasets without losing critical information.
Example: Feature Scaling in Scikit-learn
from sklearn.preprocessing import StandardScaler
# Scaling features
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
print(X_scaled)
Feature engineering is often the difference between a mediocre and a high-performing model.
Advanced Topics in Python Data Science
As you advance in your data science journey, you’ll encounter specialized topics that require deeper exploration:
1. Time Series Analysis
Time series data is prevalent in finance, economics, and IoT applications. Python libraries like statsmodels and Prophet are excellent tools for forecasting and analyzing time-dependent data.
2. Natural Language Processing (NLP)
NLP focuses on extracting meaningful insights from textual data. Libraries like NLTK, SpaCy, and Hugging Face Transformers are commonly used for tasks such as sentiment analysis and text summarization.
3. Deep Learning and Neural Networks
Deep learning enables models to learn from large volumes of unstructured data. Frameworks like TensorFlow and PyTorch provide advanced capabilities for building neural networks.
4. Automation and Pipeline Building
Automating repetitive tasks and creating data pipelines ensures efficiency in workflows. Tools like Apache Airflow and Luigi integrate well with Python for this purpose.
Conclusion
The Python Data Science Handbook is a vital resource for anyone working with data. By mastering essential tools like NumPy, Pandas, Matplotlib, and Scikit-learn, you can streamline your workflows and tackle complex problems with ease. From data manipulation to machine learning, Python provides a robust framework for every step of the data science pipeline.
As you delve deeper into Python’s capabilities, focus on practicing with real-world datasets and exploring advanced topics like deep learning, time series analysis, and big data integration. With persistence and continuous learning, you can unlock the full potential of Python for data science.