Regression analysis forms the backbone of predictive modeling and statistical inference in data science. By identifying relationships between variables, regression models enable data scientists to make informed predictions, uncover underlying trends, and perform data-driven decision-making. When implemented in R, these models offer unmatched flexibility and efficiency, thanks to R’s extensive suite of statistical tools and libraries.
This article provides a detailed exploration of regression models for data science in R, focusing on critical concepts like Ordinary Least Squares, regression to the mean, statistical linear regression models, residuals, regression inference, multivariable regression analysis, multiple variables, model selection, and Generalized Linear Models.
What Are Regression Models?
Regression models predict the value of a dependent variable based on one or more independent variables. They are essential for analyzing the relationships between variables, understanding causal influences, and forecasting future outcomes.
For example, in a business setting, regression analysis might be used to predict sales revenue based on marketing expenditure or to identify factors affecting employee productivity.
Key Concepts in Regression Analysis
Understanding the following foundational concepts is crucial for implementing regression models in R:
1. Ordinary Least Squares (OLS)
OLS is the most commonly used method for estimating the coefficients of linear regression models. It minimizes the sum of squared residuals (differences between observed and predicted values) to ensure the best fit for the data.
Implementation in R:
# Example using the Boston housing dataset
library(MASS)
data <- Boston
model <- lm(medv ~ lstat + rm, data = data)
summary(model)
Applications:
- Predicting housing prices
- Analyzing marketing campaign effectiveness
- Modeling economic trends
2. Regression to the Mean
Regression to the mean refers to the phenomenon where extreme observations tend to move closer to the mean in subsequent measurements. This concept is vital for interpreting regression results accurately, as it helps avoid misattributing natural variations to causal relationships.
Example:
If students who score extremely high on a test perform closer to the average on a second attempt, this is a regression to the mean.
# Simulate Data
set.seed(123)
data <- data.frame(before = rnorm(100, mean = 50, sd = 10))
data$after <- 0.5 * data$before + rnorm(100, mean = 0, sd = 5)
# Plot Regression
plot(data$before, data$after, main = "Regression to the Mean", xlab = "Before", ylab = "After")
abline(lm(after ~ before, data = data), col = "red")
3. Statistical Linear Regression Models
Linear regression models are fundamental tools in statistical analysis, used to explore and quantify the relationship between a dependent variable (outcome) and one or more independent variables (predictors). These models are particularly useful for predicting outcomes and understanding the strength and nature of associations in data.
Linear regression models operate under several key assumptions:
- Linearity: The relationship between predictors and the outcome is linear.
- Independence of Errors: Residuals (differences between observed and predicted values) are independent of each other.
- Homoscedasticity: Residual variance is constant across all levels of the predictors.
- Normality of Residuals: Residuals are normally distributed.
Implementation in R:
# Statistical Linear Regression Model
model <- lm(medv ~ lstat + rm + age, data = data)
summary(model)
Diagnostics:
- Residual plots to check assumptions
- R-squared and Adjusted R-squared for model fit
4. Residuals: The Heart of Regression Diagnostics
Residuals represent the difference between observed and predicted values in a regression model. Analyzing residuals is critical for diagnosing model performance and identifying patterns missed by the model.
Key Points:
- Residuals should have no pattern (random scatter) when plotted against fitted values.
- Non-random patterns may indicate model misspecification or violations of assumptions.
Plotting Residuals in R
Creating residual plots is an effective way to visualize these issues. A typical plot of residuals versus fitted values helps detect patterns or irregularities:
# Residual analysis
residuals <- resid(model)
plot(fitted(model), residuals)
abline(h = 0, col = "red")
Common Issues Identified Through Residuals
- Non-Linearity: Residual patterns that curve or cluster suggest a need for non-linear models or transformation of variables.
- Heteroscedasticity: An unequal spread of residuals indicates a violation of constant variance assumptions.
- Outliers: Extreme residuals can unduly influence the model, skewing results and reducing robustness.
Residual analysis is a crucial step in refining regression models and ensuring their reliability in practical applications.
5. Regression Inference
Regression inference involves using statistical tests to evaluate the significance of model parameters. Common metrics include:
- P-values: Assess whether a predictor is statistically significant.
- Confidence intervals: Provide a range of plausible values for coefficients.
Example in R:
# Confidence intervals for coefficients
confint(model)
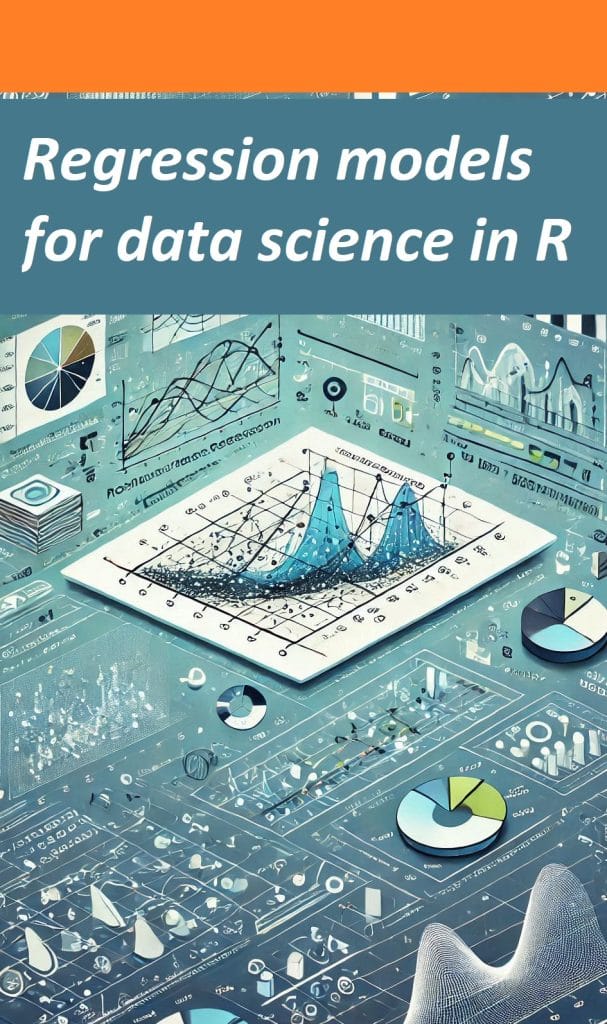
6. Multivariable Regression Analysis
Residuals play a crucial role in assessing the quality of multivariable regression models. They represent the difference between the observed and predicted values, offering insights into the model’s accuracy. In multivariable regression, residual analysis helps detect issues such as non-linearity, outliers, or heteroscedasticity that may affect the model’s validity. For instance, plotting residuals against fitted values can reveal whether the model assumptions hold true.
Benefits:
- Captures the combined effects of multiple predictors.
- Improves model accuracy for real-world applications.
Example in R:
# Plot Residuals for Multivariable Regression
plot(multi_model, which = 1, main = "Residuals vs Fitted for Multivariable Regression")
Importance of Residual Analysis:
- Detect Patterns: Identifies systematic errors that the model fails to capture.
- Validate Assumptions: Ensures residuals are randomly distributed with constant variance.
- Enhance Model Reliability: Provides a foundation for improving predictions and avoiding bias.
By focusing on residuals, data scientists can refine multivariable models for robust insights.
7. Multiple Variables and Model Selection
When building models with multiple variables, selecting the best subset of predictors is crucial to avoid overfitting and improve interpretability. Techniques include:
- Stepwise selection: Automated addition/removal of predictors based on criteria like AIC or BIC.
- Lasso regression: Shrinks some coefficients to zero, effectively performing the variable selection.
Example in R:
# Stepwise model selection
library(MASS)
stepwise_model <- stepAIC(model, direction = "both")
summary(stepwise_model)
8. Generalized Linear Models (GLMs)
Generalized linear models (GLMs) offer a flexible extension of linear regression, enabling the analysis of response variables that do not follow a normal distribution. GLMs consist of two key components:
- Link Function: This function establishes the relationship between the mean of the dependent variable and the linear predictors. Common link functions include the logit (for binary outcomes) and log (for count data). The link function transforms the data to fit within the desired model, making it possible to model a wide range of response distributions.
- Distribution: GLMs allow for various distributions to be used depending on the nature of the dependent variable. These distributions include Gaussian (normal), binomial (for binary outcomes), and Poisson (for count data). The flexibility to choose the appropriate distribution helps address the distinct characteristics of the data being modeled.
Binary GLMs:
Binary GLMs, often logistic regression, are used to model binary outcomes, where the dependent variable takes only two possible values, such as success/failure or yes/no. The logistic function is the most commonly used link function for binary GLMs, ensuring that predicted values remain between 0 and 1, which is ideal for probabilities.
Implementation in R:
# Binary GLM
binary_model <- glm(vs ~ wt + hp, data = mtcars, family = binomial)
summary(binary_model)
Count Data:
For modeling count data, GLMs often use the Poisson or negative binomial distribution. These models are suitable when the response variable represents non-negative integer counts, such as the number of occurrences of an event within a fixed period.
# Count Data Regression
count_model <- glm(am ~ wt + qsec, data = mtcars, family = poisson)
summary(count_model)
Steps for Building a Regression Model in R
Building a regression model in R involves a systematic approach to ensure robust and reliable results. Here’s a detailed breakdown of the key steps:
1. Data Exploration and Cleaning
Begin by understanding the dataset through exploratory data analysis (EDA). Use scatter plots and correlation matrices to identify relationships between variables. Address missing values using imputation techniques like mean substitution or predictive modeling, and manage outliers by capping, transformation, or removal. This step ensures the dataset is clean and ready for analysis.
2. Splitting Data
To evaluate model performance effectively, divide the dataset into training and testing sets. This helps prevent overfitting and ensures the model generalizes well to unseen data. For instance:
set.seed(123)
train_index <- sample(1:nrow(data), 0.7 * nrow(data))
train_data <- data[train_index, ]
test_data <- data[-train_index, ]
3. Model Training
Fit the regression model using the training dataset. Depending on the use case, choose between linear regression, logistic regression, or generalized linear models (lm()
or glm()
functions).
4. Model Evaluation
Evaluate the model’s performance using appropriate metrics. For regression tasks, use Mean Squared Error (MSE), and for classification models, measure accuracy or AUC. Example:
predictions <- predict(model, test_data)
mse <- mean((test_data$medv - predictions)^2)
print(mse)
5. Optimization and Selection
Refine the model by iterating on variable selection or hyperparameter tuning. Use techniques like stepwise selection or cross-validation to enhance the model’s predictive power and reduce overfitting.
Conclusion
Regression models are indispensable tools for data science, offering insights into relationships between variables and enabling accurate predictions. With R, practitioners can leverage a variety of regression techniques, from simple linear models to advanced GLMs, to tackle real-world problems effectively. By understanding concepts like OLS, residuals, multivariable regression, and model selection, you can build robust models tailored to your dataset. Combine these with R’s powerful libraries and visualization capabilities to achieve data-driven success.
Leave a Reply