Data science is revolutionizing how we solve problems, make decisions, and optimize processes. Python, a versatile and powerful programming language, has become the backbone of modern data science. Its vast libraries and simplicity make Python an essential tool for data scientists, analysts, and anyone interested in working with data. In this article, we will explore the foundations of data science with Python, how Python plays a critical role in this domain, and provide key strategies and examples to help you kick-start your journey in data science.
What is Data Science?
Data science is an interdisciplinary field that extracts knowledge and insights from structured and unstructured data using scientific methods, processes, algorithms, and systems. It combines expertise from statistics, mathematics, computer science, and domain knowledge to analyze data and drive decisions.
The typical data science process includes:
- Data Collection: Gathering raw data from various sources such as databases, APIs, or data warehouses.
- Data Cleaning: Ensuring that the data is free from errors, missing values, and inconsistencies.
- Exploratory Data Analysis (EDA): Summarizing the main characteristics of the data using visual and statistical techniques.
- Modeling: Using machine learning algorithms to create models that predict outcomes or identify patterns.
- Interpretation: Analyzing the results of the models to draw conclusions and make data-driven decisions.
Key Foundations of Data Science with Python
1. Data Collection
The first step in any data science project is collecting data. Python offers several libraries that help you gather data from a variety of sources:
- Pandas: This powerful data manipulation library allows you to import data from CSV, Excel, databases, and even web APIs. Pandas enables you to perform data wrangling with ease.
- Requests: A simple yet effective library for making HTTP requests, allowing you to scrape data from the web or interact with APIs.
For example, you can use Pandas to read a CSV file and load it into a DataFrame:
import pandas as pd
data = pd.read_csv('your_dataset.csv')
2. Data Cleaning and Preprocessing
Real-world data is rarely perfect. It often contains missing values, duplicates, or outliers that can skew analysis. Data cleaning is the process of preparing the data for analysis, and Python provides several methods to achieve this:
- Handling Missing Values: Pandas offers functions like fillna() to replace missing values or dropna() to remove rows with missing values.
- Removing Duplicates: The drop_duplicates() function helps you eliminate duplicate rows in a dataset.
- Outlier Detection: Python’s libraries like NumPy and Scipy provide functions to detect and manage outliers in numerical data.
Example:
# Filling missing values with the mean
data['column_name'].fillna(data['column_name'].mean(), inplace=True)
3. Exploratory Data Analysis in Python (EDA)
EDA is the process of visualizing and summarizing the main characteristics of your dataset to uncover patterns, spot anomalies, and test hypotheses.
- Matplotlib and Seaborn: These libraries are essential for creating visualizations such as line graphs, bar charts, and heatmaps. Seaborn, built on top of Matplotlib, is particularly useful for more advanced visualizations like pair plots and distributions.
- Descriptive Statistics: Pandas provides functions such as describe() that return statistical summaries (mean, median, quartiles, etc.) of the dataset.
Example:
import seaborn as sns
sns.pairplot(data)
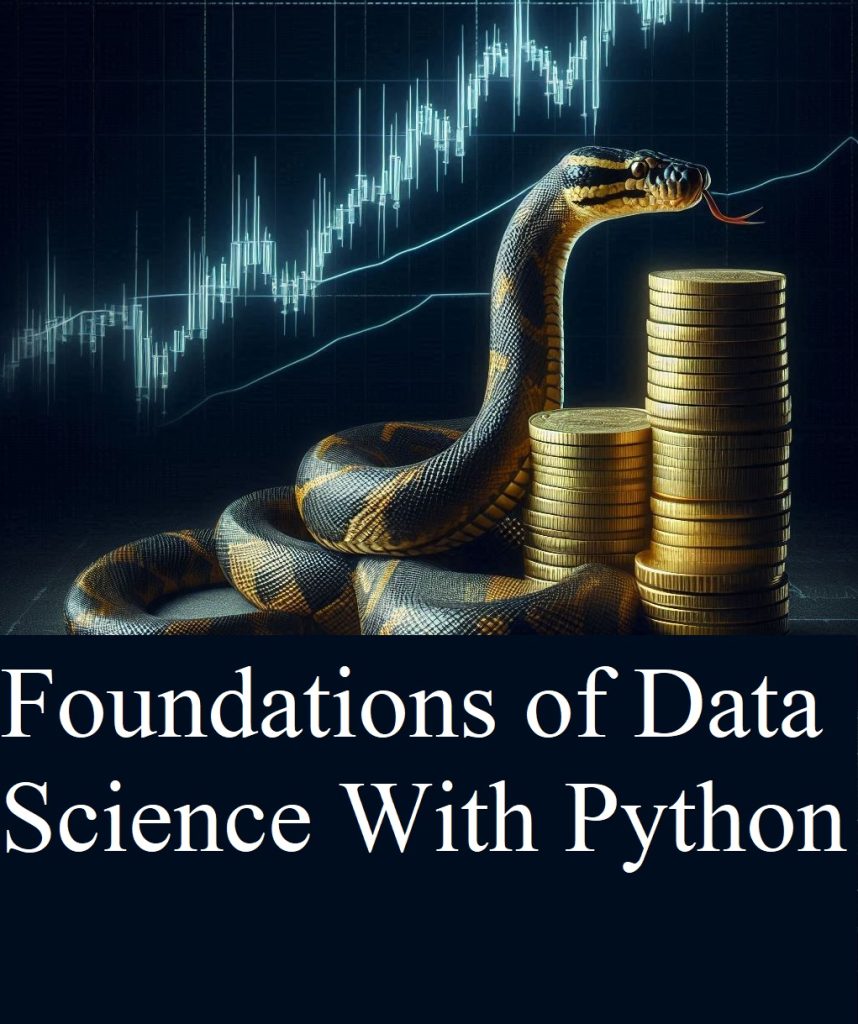
4. Feature Engineering for Data Science
Feature engineering involves creating new features from the existing data to improve the performance of machine learning models. Techniques like normalization, scaling, and encoding categorical variables are essential for preparing data for modeling.
- Scikit-learn: This library provides tools for preprocessing data, including scaling numerical data (StandardScaler) and encoding categorical data (LabelEncoder).
Example:
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
data_scaled = scaler.fit_transform(data)
5. Machine Learning Algorithms with Python
Once the data is prepared, the next step is to create models that can make predictions or classify data. Python’s Scikit-learn library is the go-to library for implementing machine learning algorithms like linear regression, decision trees, and support vector machines.
- Supervised Learning: In supervised learning, you provide the model with input-output pairs, and the model learns to map inputs to outputs. Algorithms like linear regression, decision trees, and support vector machines are examples.
- Unsupervised Learning: In unsupervised learning, the model identifies patterns and relationships in the data without predefined labels. Clustering algorithms such as K-means are common here.
Example:
from sklearn.linear_model import LinearRegression
model = LinearRegression()
model.fit(X_train, y_train)
predictions = model.predict(X_test)
6. Model Evaluation and Optimization
Once a model is trained, it’s crucial to evaluate its performance using appropriate metrics. For regression models, metrics like Mean Squared Error (MSE) and R-squared are used, while for classification models, you may use Accuracy, Precision, and Recall.
- Cross-validation: Scikit-learn’s cross_val_score() helps you test your model on multiple subsets of the dataset to avoid overfitting.
- Hyperparameter Tuning: Scikit-learn’s GridSearchCV is used to find the optimal hyperparameters for machine learning algorithms, further improving performance.
Example:
from sklearn.model_selection import cross_val_score
cv_scores = cross_val_score(model, X, y, cv=5)
7. Python Data Visualization and Reporting
The final step is to present your findings. Python provides several libraries for creating interactive visualizations:
- Plotly: This library allows you to create interactive plots that can be embedded in web applications.
- Dash: A framework built on top of Plotly that enables you to create web-based dashboards to showcase your data analysis in an interactive way.
Example:
import plotly.express as px
fig = px.scatter(data, x="column1", y="column2", color="category_column")
fig.show()
Practical Applications of Data Science with Python
1. Finance and Investment
Python is widely used in finance for predictive analytics, portfolio optimization, and algorithmic trading. Libraries such as TA-Lib allow you to perform technical analysis on stock data, while QuantLib enables the modeling of complex financial instruments.
2. Healthcare and Medicine
Data science is transforming healthcare by enabling the analysis of large datasets to predict disease outbreaks, optimize hospital operations, and provide personalized treatments. Python is often used to analyze electronic health records (EHRs), with libraries like Lifelines for survival analysis and PyMC3 for Bayesian inference.
3. Marketing and Retail
Data science is crucial for understanding customer behavior, segmenting markets, and predicting demand. Python’s machine learning capabilities are used in customer segmentation, recommendation engines, and sentiment analysis.
Conclusion
Data science with Python is a powerful combination that opens up endless possibilities for solving complex problems across industries. By mastering the foundations of data science with Python, you will be equipped with the tools and skills needed to thrive in today’s data-driven world. Whether you’re working in finance, healthcare, marketing, or any other field, Python’s versatility and wide range of libraries make it the ideal programming language for data analysis, machine learning, and visualization.