Data visualization with python plays a crucial role in the field of finance, where the ability to quickly interpret and act on data-driven insights is essential. Financial Planning & Analysis (FP&A) teams are tasked with analyzing financial data, forecasting trends, and making recommendations to guide strategic decision-making. Effective data visualization techniques can turn complex datasets into clear, actionable visuals, enabling FP&A professionals to communicate findings more effectively.
Python, with its powerful libraries and ease of use, has become a go-to tool for financial analysts seeking to create impactful data visualizations. In this article, we will explore how Python can be utilized for data visualization in finance, particularly in the context of FP&A. We will delve into the best practices for creating financial visuals, the key Python libraries used, and practical examples to help you get started.
Key Python Libraries for Data Visualization in Finance
Python offers several libraries specifically designed for data visualization. Here are the most commonly used libraries in financial analysis:
1. Matplotlib
Matplotlib is a foundational plotting library in Python, known for its simplicity and versatility. It provides a wide range of plotting options, including line charts, bar graphs, scatter plots, and histograms. Its flexibility makes it ideal for creating customized financial visuals.
2. Seaborn
Seaborn is built on top of Matplotlib and offers a more visually appealing interface with themes and color palettes that make graphs easier to interpret. It’s particularly useful for statistical plots and can be used for creating more sophisticated visuals, such as heatmaps and violin plots.
3. Plotly
Plotly is an interactive graphing library that allows users to create dynamic, web-based visualizations. This library is ideal for creating dashboards and visualizations that require interactivity, such as drill-down capabilities in financial data.
4. Pandas Visualization
Pandas, a powerful data manipulation library, also offers built-in plotting capabilities that are simple and easy to use for quick visualizations. This feature is particularly useful for financial analysts working directly with data in DataFrames.
5. Altair
Altair is a declarative statistical visualization library for Python. It enables analysts to create complex visualizations with concise syntax, making it easier to build layered and interactive plots, which are particularly useful in FP&A for exploring multiple dimensions of financial data.
Best Practices for Financial Data Visualization
Creating effective financial visualizations requires more than just knowing how to use Python libraries. Here are some best practices to follow:
1. Understand Your Audience
Before creating any visual, consider who will be viewing it. Executives may prefer high-level dashboards, while analysts might need detailed charts. Tailor your visuals to the needs of your audience to ensure the information is both relevant and understandable.
2. Keep It Simple
Simplicity is key in data visualization. Avoid cluttering your charts with unnecessary elements. Focus on the most important data points and use clear labels, legends, and titles to guide the viewer.
3. Choose the Right Chart Type
Different data types require different visual representations. For instance:
- Line charts are great for showing trends over time.
- Bar charts are ideal for comparing different categories.
- Scatter plots can be used to show relationships between variables.
Choosing the right chart type helps convey your message more effectively.
4. Use Consistent Scales and Colors
Consistency in scales and color schemes makes it easier for viewers to compare data across different visuals. For example, using the same color for revenue across multiple charts helps maintain clarity.
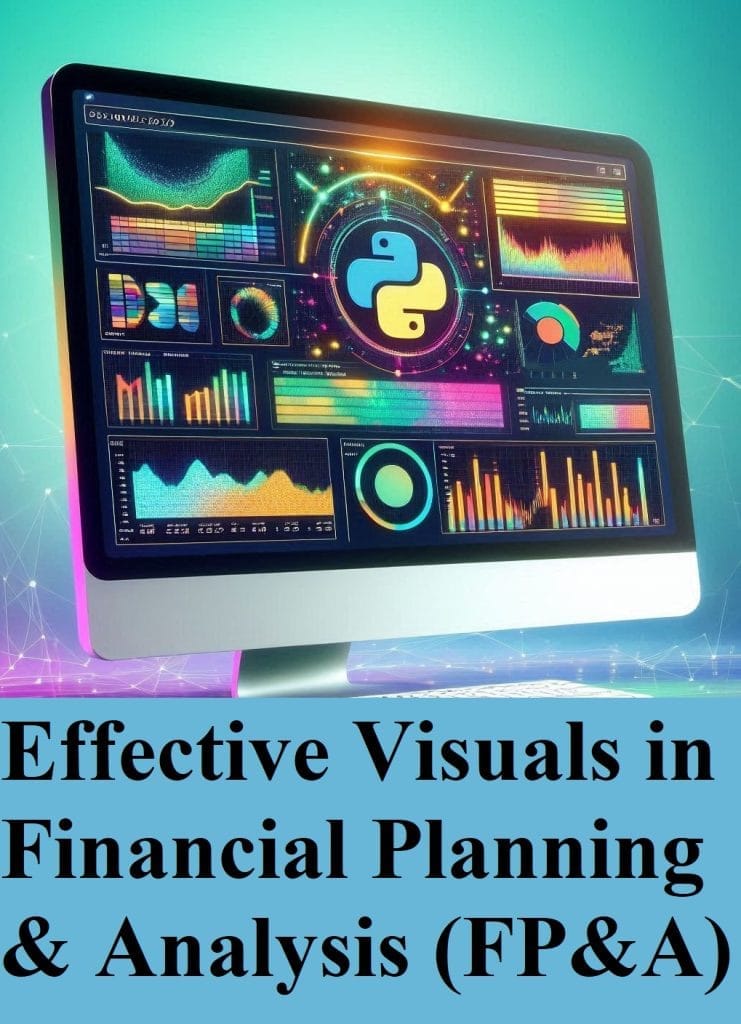
5. Highlight Key Insights
Use color and annotations to draw attention to key insights in your data. For example, highlight a sudden drop in revenue or a spike in expenses to ensure viewers immediately understand the significance of the data.
6. Test Your Visuals
Always test your visuals with a sample audience or peers to ensure they are clear, accurate, and convey the intended message. Feedback can help you refine your charts before presenting them to a broader audience.
Advanced Data Visualization Techniques
1. Plot a time series
Time series plots are essential for tracking financial metrics over time, such as revenue, expenses, or stock prices. These plots can help FP&A teams identify trends, seasonality, and potential anomalies in the data.
import matplotlib.pyplot as plt
import pandas as pd
# Sample time series data
data = {'Date': pd.date_range(start='2024-01-01', periods=12, freq='M'),
'Revenue': [100000, 120000, 130000, 125000, 140000, 150000, 155000, 160000, 170000, 180000, 190000, 200000]}
df = pd.DataFrame(data)
plt.plot(df['Date'], df['Revenue'], marker='o')
plt.title('Monthly Revenue Trend')
plt.xlabel('Date')
plt.ylabel('Revenue ($)')
plt.grid(True)
plt.xticks(rotation=45)
plt.show()
This time series plot shows monthly revenue trends, making it easier to spot seasonal patterns or changes in growth rates.
2. Correlation Matrix in Python
A correlation matrix is a useful tool for examining the relationships between multiple financial variables. It helps in understanding how different variables, such as revenue, expenses, and profits, are correlated with each other.
import seaborn as sns
import numpy as np
# Sample data
data = {'Revenue': [100000, 120000, 130000, 125000, 140000],
'Profit': [20000, 25000, 30000, 28000, 35000],
'Expenses': [80000, 95000, 100000, 97000, 105000]}
df = pd.DataFrame(data)
# Generate correlation matrix
corr = df.corr()
sns.heatmap(corr, annot=True, cmap='coolwarm')
plt.title('Correlation Matrix of Financial Metrics')
plt.show()
This correlation matrix visualizes the relationships between revenue, profit, and expenses, helping FP&A teams identify which variables move together.
3. Histogram
Histograms are used to display the distribution of a single financial variable, such as the distribution of daily returns on a stock or the frequency of different expense amounts. This helps in understanding the data’s spread and identifying any skewness or outliers.
# Sample data
data = {'Returns': np.random.normal(0, 1, 1000)}
df = pd.DataFrame(data)
plt.hist(df['Returns'], bins=30, color='blue', alpha=0.7)
plt.title('Distribution of Daily Returns')
plt.xlabel('Returns')
plt.ylabel('Frequency')
plt.show()
The histogram above shows the distribution of daily returns, allowing analysts to assess the risk and volatility of an investment.
4. Scatter Plot
Scatter plots are used to examine the relationship between two financial variables. For example, a scatter plot can show the relationship between revenue and profit, helping to determine if higher revenues consistently lead to higher profits.
# Sample data
data = {'Revenue': [100000, 120000, 130000, 125000, 140000],
'Profit': [20000, 25000, 30000, 28000, 35000]}
df = pd.DataFrame(data)
plt.scatter(df['Revenue'], df['Profit'], color='green')
plt.title('Revenue vs. Profit')
plt.xlabel('Revenue ($)')
plt.ylabel('Profit ($)')
plt.grid(True)
plt.show()
This scatter plot visualizes the relationship between revenue and profit, showing a positive correlation.
5. Bar Chart
Bar charts are ideal for comparing financial data across different categories, such as expenses by department or revenue by product line. They are useful for highlighting key differences and trends within categorical data.
# Sample data
expenses = {'Department': ['Marketing', 'Salaries', 'Operations', 'R&D'],
'Amount': [50000, 150000, 80000, 40000]}
df_expenses = pd.DataFrame(expenses)
sns.barplot(x='Department', y='Amount', data=df_expenses)
plt.title('Expense Distribution by Department')
plt.ylabel('Amount ($)')
plt.show()
This bar chart compares expenses across different departments, providing a clear view of where the most resources are allocated.
6. Pie Chart
Pie charts are used to show the proportion of categories in a whole, such as the distribution of asset classes in a portfolio. Although less favored for precise comparisons, pie charts can be useful for presenting a high-level view of data distribution.
# Sample data
portfolio = {'Asset Class': ['Stocks', 'Bonds', 'Real Estate', 'Cash'],
'Allocation': [50, 20, 20, 10]}
df_portfolio = pd.DataFrame(portfolio)
plt.pie(df_portfolio['Allocation'], labels=df_portfolio['Asset Class'], autopct='%1.1f%%')
plt.title('Portfolio Allocation')
plt.show()
This pie chart shows how a portfolio is allocated across different asset classes, providing a visual representation of diversification.
7. Box and Whisker Plot
Box and whisker plots are effective for visualizing the spread and outliers in financial data, such as quarterly returns or expense distributions. They provide a clear view of the data’s central tendency, variability, and outliers.
# Sample data
data = {'Quarterly Returns': np.random.normal(10, 5, 100)}
df = pd.DataFrame(data)
sns.boxplot(data=df, x='Quarterly Returns')
plt.title('Quarterly Returns Distribution')
plt.xlabel('Returns (%)')
plt.show()
This box and whisker plot displays the distribution of quarterly returns, highlighting the median, quartiles, and any outliers.
8. Risk Heatmaps
Risk heatmaps are used to assess and visualize the risk associated with different financial metrics or investments. They help FP&A teams identify high-risk areas that require attention.
# Sample risk data
risk_data = {'Investment': ['Stock A', 'Stock B', 'Stock C', 'Stock D'],
'Risk Level': [0.9, 0.5, 0.7, 0.2]}
df_risk = pd.DataFrame(risk_data)
sns.heatmap(df_risk[['Risk Level']].T, annot=True, cmap='Reds', cbar=False)
plt.title('Investment Risk Heatmap')
plt.show()
This heatmap visualizes the risk levels associated with different investments, making it easier to prioritize risk management efforts. From tracking time series data to assessing risk with heatmaps, these visualization techniques enable a deeper understanding of financial data and support strategic financial planning. By leveraging these tools effectively, organizations can enhance their financial reporting, optimize decision-making, and ultimately improve their financial health.
Practical Examples of Data Visualization Using Python in FP&A
1. Revenue Trend Analysis with Matplotlib
Revenue trend analysis is a fundamental task in FP&A. By visualizing revenue data over time, analysts can identify seasonal patterns, growth rates, and anomalies.
import matplotlib.pyplot as plt
import pandas as pd
# Sample data
data = {'Month': ['Jan', 'Feb', 'Mar', 'Apr', 'May'],
'Revenue': [100000, 120000, 130000, 125000, 140000]}
df = pd.DataFrame(data)
plt.plot(df['Month'], df['Revenue'], marker='o')
plt.title('Monthly Revenue Trend')
plt.xlabel('Month')
plt.ylabel('Revenue ($)')
plt.grid(True)
plt.show()
This simple line chart illustrates the trend in monthly revenue, allowing FP&A teams to easily communicate revenue performance to stakeholders.
2. Expense Distribution with Seaborn
Understanding the distribution of expenses is crucial for budget management. Seaborn can create visually appealing bar charts to represent expense categories.
import seaborn as sns
# Sample data
expenses = {'Category': ['Marketing', 'Salaries', 'Operations', 'R&D'],
'Amount': [50000, 150000, 80000, 40000]}
df_expenses = pd.DataFrame(expenses)
sns.barplot(x='Category', y='Amount', data=df_expenses)
plt.title('Expense Distribution')
plt.ylabel('Amount ($)')
plt.show()
This bar chart helps in visualizing how expenses are distributed across different categories, aiding in expense management and optimization.
3. Interactive Financial Dashboard with Plotly
For more advanced needs, such as creating interactive dashboards, Plotly is an excellent choice. It allows users to explore data through interactive elements, making it easier to drill down into specific areas of interest.
import plotly.express as px
# Sample data
df = pd.DataFrame({
"Month": ["Jan", "Feb", "Mar", "Apr", "May"],
"Revenue": [100000, 120000, 130000, 125000, 140000],
"Profit": [20000, 25000, 30000, 28000, 35000]
})
fig = px.line(df, x="Month", y=["Revenue", "Profit"], title="Revenue and Profit Over Time")
fig.show()
This interactive line chart allows users to toggle between revenue and profit data, offering a dynamic view of financial performance.
Conclusion
Advanced data visualization is a powerful tool in the arsenal of FP&A professionals. By leveraging Python’s visualization libraries, analysts can turn complex financial data into clear, insightful visuals that drive better decision-making. From simple line charts to interactive dashboards, Python provides the flexibility and power needed to enhance financial planning and analysis efforts.
By following best practices in data visualization using Python, you can significantly improve your ability to communicate financial insights and add value to your organization. Whether you are forecasting revenue, managing expenses, or optimizing investments, effective data visualization is key to achieving your FP&A goals.