In the digital age, marketing research and analytics have become crucial for businesses aiming to stay competitive. The integration of technology into these processes allows marketers to uncover valuable insights and make data-driven decisions. With its wide range of libraries and capabilities, Python is reshaping how businesses understand their customers, analyze trends, and optimize campaigns. This article explores how we can leverage Python for marketing research and analytics, focusing on three essential topics: data collection and cleaning, data analysis and visualization, and advanced techniques.
Essential Python Libraries for Marketing Analytics
Python’s rich ecosystem of libraries simplifies marketing research and analytics. Below are some key libraries and their applications:
- Pandas: This library mainly use for handling, cleaning, and analyzing data, offering robust tools for filtering, aggregating, and manipulating datasets.
- NumPy: Perfect for numerical computations, NumPy provides efficient tools for working with large datasets and performing mathematical operations.
- Matplotlib and Seaborn: These libraries are essential for creating visually appealing graphs and charts to communicate insights effectively.
- Scikit-learn: A powerful library for implementing machine learning algorithms, ideal for tasks like predictive modeling and clustering.
- NLTK and TextBlob: Popular for natural language processing, these libraries help in sentiment analysis, text classification, and keyword extraction.
- BeautifulSoup and Scrapy: Used for web scraping, they extract structured data from websites for competitive analysis and trend tracking.
- Statsmodels: Provides advanced tools for statistical modeling, hypothesis testing, and time series analysis.
- Google API Client: Enables seamless integration with Google Analytics, Ads, and other platforms to automate data extraction and reporting.
Data Collection and Cleaning with Python
Before delving into analysis, the first step in marketing research is gathering and preparing data. Python offers tools and libraries that streamline this process, ensuring marketers work with clean, reliable datasets.
1. Web Scraping for Competitive Analysis
Web scraping involves extracting data from websites to gain insights into competitors’ strategies, pricing, and customer preferences. Python libraries like BeautifulSoup and Scrapy make it easy to collect structured data from various sources.
Example Use Case:
A business can scrape competitor websites to monitor product pricing trends, reviews, and promotional campaigns.
Code Snippet for Web Scraping:
from bs4 import BeautifulSoup
import requests
# Fetch webpage content
url = "https://competitor-website.com/products"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# Extract product titles and prices
products = [
{"title": item.text, "price": item.find_next('span', class_='price').text}
for item in soup.find_all('h2', class_='product-title')
]
print(products)
2. Data Parsing and Cleaning
After collecting data, the next step is cleaning and structuring it. Raw data often contains inconsistencies, duplicates, or missing values. Python’s Pandas library provides robust tools for parsing, cleaning, and transforming data into a usable format.
Example Use Case:
An e-commerce company can clean scraped customer reviews by removing null values, standardizing date formats, and handling duplicates.
Code Snippet for Data Cleaning:
import pandas as pd
# Load raw data
data = pd.read_csv('raw_reviews.csv')
# Drop duplicates and fill missing values
data = data.drop_duplicates().fillna({'review_text': 'No review'})
# Standardize date format
data['review_date'] = pd.to_datetime(data['review_date'])
print(data.head())
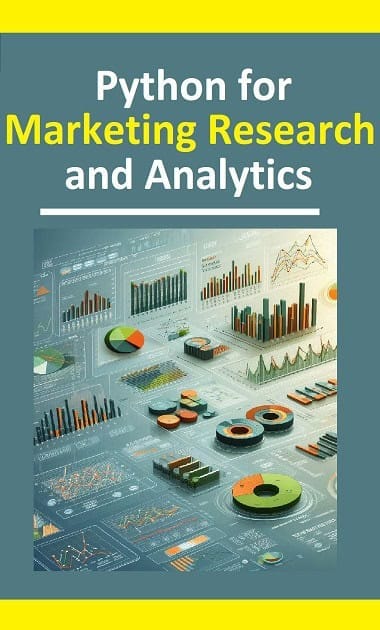
Data Analysis and Visualization with Python
Once the data is clean, analyzing and visualizing it allows marketers to uncover actionable insights. Python’s ecosystem includes powerful tools for these tasks.
1. Pandas for Data Analysis
Pandas is an essential library for data manipulation and analysis. It allows marketers to filter, group, and summarize data with ease.
Example Use Case:
A marketing team can use Pandas to analyze campaign performance metrics like click-through rates (CTR) and conversion rates (CR).
Code Snippet for Data Analysis:
# Calculate campaign metrics
campaign_data = data.groupby('campaign_name').agg(
CTR=('clicks', lambda x: sum(x) / sum(data['impressions']) * 100),
CR=('conversions', lambda x: sum(x) / sum(data['clicks']) * 100)
)
print(campaign_data)
2. Matplotlib and Seaborn for Visualization
Visualizing data helps convey complex insights in a digestible format. Libraries like Matplotlib and Seaborn offer extensive options for creating charts and graphs.
Example Use Case:
Marketers can use visualizations to present campaign trends, customer segmentation, or sales performance.
Code Snippet for Visualization:
import seaborn as sns
import matplotlib.pyplot as plt
# Plot CTR by campaign
sns.barplot(x=campaign_data.index, y=campaign_data['CTR'])
plt.title('CTR by Campaign')
plt.xlabel('Campaign')
plt.ylabel('Click-Through Rate (%)')
plt.show()
Advanced Techniques in Python for Marketing Research
For marketers aiming to take their analysis to the next level, Python provides advanced techniques like machine learning and natural language processing (NLP).
1. Machine Learning Applications For Marketing
Machine learning (ML) enables predictive analytics, helping marketers anticipate customer behavior, optimize ad spend, and personalize campaigns. Python’s Scikit-learn and TensorFlow libraries enable marketers to build predictive models for sales forecasting, customer churn prediction, and more.
Example Use Case: Predictive Analytics: A subscription-based business can predict which customers are likely to cancel their subscriptions and proactively engage them with retention offers.
Code Snippet for Churn Prediction:
from sklearn.ensemble import RandomForestClassifier
from sklearn.model_selection import train_test_split
# Prepare data
X = data[['age', 'monthly_spend', 'engagement_score']]
y = data['churn']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3)
# Train model
model = RandomForestClassifier()
model.fit(X_train, y_train)
# Predict churn
predictions = model.predict(X_test)
print(predictions)
2. Natural Language Processing (NLP) for Text Analysis
NLP helps analyze unstructured text data like customer reviews, survey responses, and social media posts. Understanding customer sentiment is critical for shaping marketing strategies. Python’s Natural Language Toolkit (NLTK) and TextBlob libraries allow businesses to analyze customer reviews, social media posts, and survey responses.
Example Use Case:
An e-commerce company can analyze customer reviews to identify common pain points and improve product offerings.
Code Snippet for Sentiment Analysis Using Python:
from textblob import TextBlob
# Analyze sentiment
reviews = ["Great product!", "Terrible customer service.", "Average experience."]
sentiments = [TextBlob(review).sentiment.polarity for review in reviews]
for review, sentiment in zip(reviews, sentiments):
print(f"Review: {review}, Sentiment Score: {sentiment}")
3. Customer Segmentation
Customer segmentation involves dividing a customer base into groups based on shared characteristics. Python simplifies this process with libraries like Pandas and Scikit-learn.
Example Use Case:
A retail business can use Python to segment customers based on purchase history, browsing behavior, and demographics. By identifying high-value customers, the company can create targeted campaigns to boost revenue.
Code Snippet for K-Means Clustering:
from sklearn.cluster import KMeans
import pandas as pd
# Load data
data = pd.read_csv('customer_data.csv')
# Apply K-Means clustering
kmeans = KMeans(n_clusters=5)
data['Segment'] = kmeans.fit_predict(data[['Annual_Spend', 'Purchase_Frequency']])
# View segmented data
print(data.head())
Integrating Python with Marketing Platforms
Python’s versatility extends to its integration capabilities. With APIs, marketers can connect Python scripts to platforms like Google Analytics, Facebook Ads, and CRMs for seamless data extraction and reporting.
Example Use Case:
A marketing team can automate the retrieval of performance data from Google Analytics for weekly reporting.
Code Snippet for API Integration:
from googleapiclient.discovery import build
# Connect to Google Analytics API
analytics = build('analyticsreporting', 'v4', developerKey='API_KEY')
# Fetch report
response = analytics.reports().batchGet(body={
'reportRequests': [{
'viewId': '12345678',
'dateRanges': [{'startDate': '7daysAgo', 'endDate': 'today'}],
'metrics': [{'expression': 'ga:sessions'}, {'expression': 'ga:goalCompletionsAll'}]
}]
}).execute()
print(response)
Conclusion
Python has revolutionized marketing research and analytics, offering tools to streamline every stage of the process. From collecting and cleaning data to advanced analytics like machine learning and NLP, Python equips marketers with the capabilities to make data-driven decisions and stay competitive. By embracing Python, marketers can unlock deeper insights, automate workflows, and elevate their strategies to new heights.
Leave a Reply