The world of image processing has been revolutionized by the integration of machine learning, enabling systems to analyze, interpret, and manipulate visual data. From detecting palm lines to classifying complex images using advanced algorithms, these technologies have a wide array of applications. This article explores machine learning and image processing fundamentals, Python libraries, and advanced techniques, culminating in real-world use cases to highlight the transformative potential of these tools.
Introduction to Image Processing
Image processing involves transforming and analyzing visual data—photos or videos—to extract meaningful insights or improve their quality. Historically, image processing relied on rule-based techniques like filtering, thresholding, and segmentation. However, as datasets grew and computational power increased, machine learning transformed image processing into a more dynamic, automated field.
Applications range from medical imaging and autonomous vehicles to biometric security systems and virtual reality environments. With the power of machine learning, image processing has moved from static analysis to predictive and adaptive operations.
Basics of Python and Scikit-Image
Python has become the go-to programming language for image processing due to its simplicity, versatility, and rich ecosystem of libraries. Among these libraries, Scikit-Image stands out as a powerful tool for handling a wide range of image processing tasks. In addition to Scikit-Image, Python supports libraries like OpenCV and Pillow (PIL), which complement each other in providing diverse functionalities for both beginners and professionals.
Key Features of Scikit-Image
Scikit-Image is designed to make complex image processing tasks intuitive and efficient. Built on NumPy and SciPy, it ensures compatibility with other Python scientific computing libraries, making it ideal for both academic research and real-world applications.
- Image Manipulation: It offers straightforward methods for resizing, cropping, rotating, and rescaling images, enabling users to preprocess images effectively.
- Filters: Built-in tools for edge detection, noise reduction, and blurring make it easy to enhance image quality and highlight essential features.
- Segmentation: Advanced segmentation techniques allow for the isolation and analysis of specific objects or regions within an image.
Example: Loading and Displaying an Image with Scikit-Image
from skimage import io
# Load and display an image
image = io.imread('example.jpg')
io.imshow(image)
io.show()
With Scikit-Image, users can efficiently perform preprocessing, such as filtering or resizing, before applying more complex machine learning models or image transformations. Its clean API and extensive documentation make it a favorite for beginners and experts alike.
Advanced Image Processing Using OpenCV
OpenCV (Open Source Computer Vision) is one of the most widely used libraries for real-time computer vision tasks. Designed for efficiency and flexibility, OpenCV provides developers with powerful tools to process and analyze images, detect features, and apply complex transformations. With a rich collection of pre-built functions and algorithms, it simplifies the implementation of advanced image processing tasks.
1. Blending Two Images
Image blending involves combining two images to create a seamless effect, commonly used in creative tasks like overlays, watermarking, or image compositing. OpenCV makes blending simple with its addWeighted function, which assigns weights to two images and combines them pixel by pixel. By adjusting these weights, you can control the visibility of each image in the final result.
Key Application:
Blending is widely used in photo editing software, logo overlays, and image stitching in panorama creation.
Example Code:
import cv2
# Read two images
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
# Resize images to the same size
img2 = cv2.resize(img2, img1.shape[1::-1])
# Blend images
blended = cv2.addWeighted(img1, 0.7, img2, 0.3, 0)
# Display blended image
cv2.imshow('Blended Image', blended)
cv2.waitKey(0)
cv2.destroyAllWindows()
Example Code Explanation:
- cv2.imread: Reads the two input images.
- cv2.resize: Ensures the images are the same size for blending.
- cv2.addWeighted: Combines images with specified weights.
The resulting image showcases a smooth blend, ideal for enhancing aesthetic appeal or creating artistic effects.
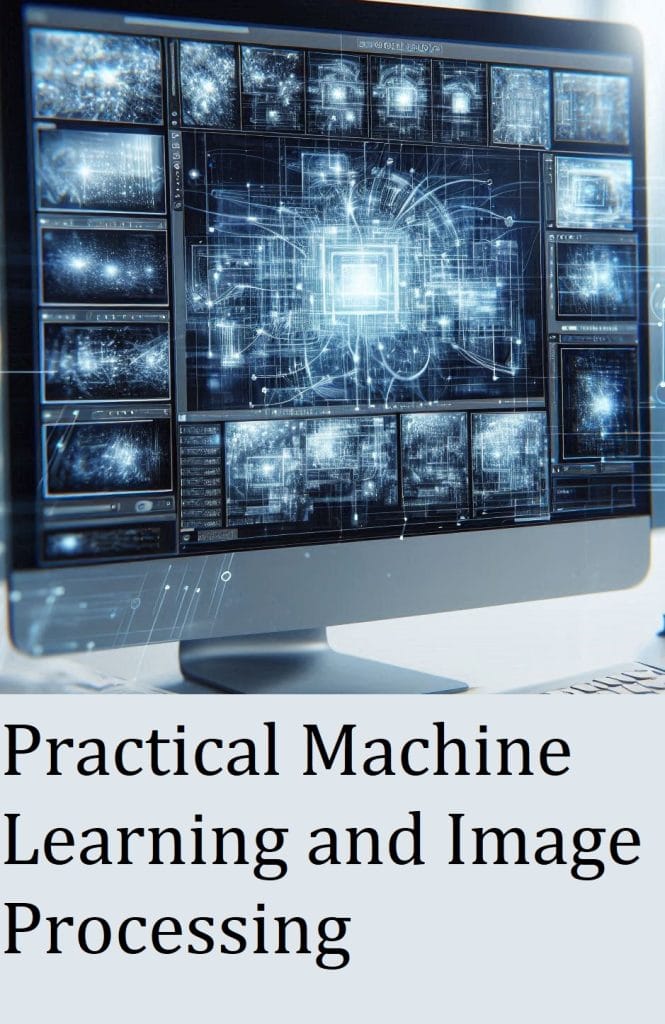
2. Changing Contrast and Brightness
Modifying contrast and brightness can significantly enhance an image’s clarity. Contrast adjusts the difference between dark and light areas, while brightness adds or subtracts overall intensity. These operations are crucial for preprocessing poorly captured images or enhancing specific details.
Practical Use Cases:
- Improving visibility in underexposed photos.
- Enhancing medical images for better diagnostics.
- Preparing data for machine learning by normalizing image properties.
Example Code:
# Change contrast (alpha) and brightness (beta)
adjusted = cv2.convertScaleAbs(img1, alpha=1.5, beta=50)
cv2.imshow('Adjusted Image', adjusted)
cv2.waitKey(0)
cv2.destroyAllWindows()
Example Code Explanation:
- The alpha parameter controls contrast, where values >1 increase contrast and values <1 decrease it.
- The beta parameter adds a constant value to all pixels, adjusting brightness.
By manipulating these parameters, OpenCV enables tailored adjustments for diverse applications. OpenCV simplifies operations like face detection, motion tracking, and even lane detection, making it indispensable for developers.
Image Processing Using Machine Learning
Machine learning enhances image processing by enabling systems to learn from data patterns, adapt to complex scenarios, and automate decision-making processes. Traditional rule-based algorithms often fall short when dealing with large-scale, dynamic datasets. Machine learning addresses these limitations by creating adaptive models capable of handling intricate tasks like feature detection, image alignment, and classification. Below are some advanced ML techniques widely used in image processing:
1. Feature Mapping Using the SIFT Algorithm
The Scale-Invariant Feature Transform (SIFT) algorithm is a cornerstone in feature detection and matching. Developed by David Lowe, SIFT identifies key points in an image that remain consistent despite changes in scale, rotation, or illumination. It generates robust descriptors for each key point, enabling precise feature matching across images.
How It Works:
- The algorithm detects scale-space extrema using Gaussian filters, isolating key points in an image.
- For each key point, it calculates orientation and scale, making the descriptors invariant to transformations.
- Finally, SIFT creates a distinctive descriptor for each key point by analyzing the local gradient orientation around it.
Applications:
- Object Recognition: Matching features of objects in real-world scenes.
- Robotics: Visual navigation by identifying landmarks.
- Cultural Heritage: Aligning and analyzing historical or architectural images for conservation purposes.
2. Image Registration Using the RANSAC Algorithm
Image registration is the process of aligning multiple images into a unified coordinate system, critical in fields like remote sensing, medical imaging, and computer vision. The RANSAC (Random Sample Consensus) algorithm is a robust technique for finding the optimal transformation between corresponding points in images, even when a dataset contains outliers.
How It Works:
- RANSAC iteratively selects random subsets of points to estimate a transformation model.
- It evaluates this model against all data points, identifying the set that best fits.
- This approach ensures accurate registration, even in noisy datasets.
Applications:
- Medical Imaging: Aligning scans from different modalities, such as MRI and CT, for comprehensive diagnostics.
- Cartography: Stitching aerial or satellite images to create accurate maps.
- Augmented Reality (AR): Overlaying virtual elements on real-world environments.
3. Image Classification Using Artificial Neural Networks (ANNs)
Artificial Neural Networks (ANNs) have revolutionized image classification by mimicking the human brain’s ability to recognize patterns. ANNs, especially when paired with convolutional layers (CNNs), excel at processing pixel data to identify objects, animals, or scenes in images.
How It Works:
ANNs are trained on labeled datasets, where each image is associated with a specific class. During training, the network learns to extract meaningful features (e.g., shapes, textures) and associates them with their respective labels. Once trained, the model can classify new, unseen images accurately.
Example Code:
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense, Flatten, Conv2D, MaxPooling2D
# Define CNN model
model = Sequential([
Conv2D(32, kernel_size=(3, 3), activation='relu', input_shape=(64, 64, 3)),
MaxPooling2D(pool_size=(2, 2)),
Flatten(),
Dense(128, activation='relu'),
Dense(10, activation='softmax') # 10 classes
])
# Compile and train model
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# Assume 'train_images' and 'train_labels' are preprocessed
model.fit(train_images, train_labels, epochs=10, batch_size=32)
This code demonstrates how to build and train a convolutional neural network using TensorFlow. Such models are widely used in applications like medical imaging for disease detection or e-commerce for product categorization.
Example Use Case: Online retailers use ANNs to automatically categorize products based on their images, improving search efficiency and user experience.
Real-Time Use Cases in Image Processing
Image processing has grown into a pivotal technology, seamlessly integrating with real-time applications to reshape industries and enhance everyday life. Its adaptability to dynamic environments has unlocked innovative solutions across various domains. Below are some of the most impactful real-time use cases.
1. Finding Palm Lines
Palm line detection is a unique application of image processing that uses edge-detection algorithms to analyze intricate line patterns on the human palm. These lines are studied in two primary contexts:
- Chiromancy (Palmistry): By extracting detailed features of palm lines, image processing assists in interpreting these patterns for astrological or cultural beliefs.
- Medical Diagnostics: In healthcare, the texture and depth of palm lines can reveal skin conditions or aid in biometric studies to detect diseases. Tools like Canny Edge Detection and Sobel Filters are commonly used for this task, ensuring precision in line extraction.
2. Detecting Faces
Facial recognition has become synonymous with real-time image processing. Using Haar cascades for feature detection or advanced deep learning models, systems can quickly identify and authenticate faces. Real-world implementations include:
- Smartphone Authentication: Features like Face ID rely on machine learning models to compare captured facial data with stored templates.
- Security and Surveillance: Airports, shopping malls, and public spaces deploy facial recognition to enhance safety by identifying individuals on watchlists or detecting suspicious activity in real-time.
3. Tracking Movement
Motion tracking is a combination of image processing techniques like background subtraction, optical flow analysis, and object detection. This technology plays a critical role in:
- Surveillance Systems: Monitoring unauthorized access or unusual activity in secured premises.
- Sports Analytics: Capturing player movements during games to provide performance insights and refine strategies.
4. Detecting Lanes
Lane detection is fundamental to autonomous vehicles, enabling them to navigate roads safely. By leveraging the Hough Transform for line detection and color segmentation for boundary identification, systems can ensure real-time responsiveness. Key features include:
- Identifying straight and curved lanes in diverse weather conditions.
- Assisting Advanced Driver Assistance Systems (ADAS) to prevent accidents by issuing lane departure warnings.
The following Python code demonstrates basic lane detection using OpenCV, showcasing how image processing is applied in real-world scenarios:
import cv2
import numpy as np
# Load and preprocess image
image = cv2.imread('road.jpg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
edges = cv2.Canny(gray, 50, 150)
# Detect lines using Hough Transform
lines = cv2.HoughLinesP(edges, 1, np.pi/180, threshold=100, minLineLength=50, maxLineGap=10)
for line in lines:
x1, y1, x2, y2 = line[0]
cv2.line(image, (x1, y1), (x2, y2), (0, 255, 0), 3)
cv2.imshow('Lane Detection', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
From personal devices to critical systems, these real-time use cases highlight how image processing enhances functionality, safety, and decision-making across diverse fields.
Conclusion
Image processing powered by machine learning is a cornerstone of modern technology, offering solutions to challenges across industries. By mastering Python libraries like Scikit-Image and OpenCV and implementing advanced algorithms like SIFT and RANSAC, developers can build efficient, cutting-edge applications. Real-time use cases such as lane detection, motion tracking, and facial recognition illustrate the practicality and scope of these innovations.
This fusion of machine learning and image processing not only enhances automation but also opens the door to exciting possibilities in fields ranging from healthcare to entertainment. As technology continues to evolve, mastering these tools will be crucial for staying ahead in the digital age.