Machine learning has become an indispensable tool for solving real-world problems in fields like healthcare, finance, and e-commerce. Python, with its simplicity and robust ecosystem of libraries, is one of the most popular languages for implementing machine learning models.
This Machine Learning with Python Tutorial covers essential topics to help you understand and apply machine learning with Python effectively. It also discusses machine learning terminology, loading datasets using scikit-learn, implementing classifiers and neural networks, and working with regression trees.
Machine Learning Terminology
Understanding the basic terminology of machine learning is crucial for building robust models and interpreting their behavior effectively. Below are some key concepts explained in detail:
Dataset: A dataset is the foundation of any machine learning project, consisting of data points used to train and test the model. It is usually divided into:
- Training Set: A subset of data used to teach the model by allowing it to learn patterns and relationships.
- Test Set: A separate subset used to evaluate the model’s performance and ensure it generalizes well to unseen data.
Features: These are the input variables (independent variables) used to predict the output. Features can be numerical, categorical, or derived from preprocessing raw data.
Target/Label: This is the output variable (dependent variable) that the model aims to predict, such as a classification label or a regression value.
Model: A mathematical representation that processes input features to predict the target variable.
Overfitting: Occurs when the model captures noise or random fluctuations in the training data, leading to poor performance on new data.
Underfitting: Happens when the model is too simple to capture the underlying patterns in the data.
Epochs: Refers to the number of complete passes through the training dataset during the learning process. More epochs can lead to better learning but may risk overfitting.
Working with Datasets in scikit-learn
Scikit-learn provides a collection of built-in datasets that are highly useful for practicing and testing machine learning algorithms. These datasets come preloaded with features and labels, making it easy to focus on implementing algorithms rather than data preprocessing. Two commonly used datasets are the Iris dataset and the Digits dataset, which are excellent for classification problems.
Loading the Iris Dataset with Scikit-learn
The Iris dataset is a well-known dataset in the machine learning community. It contains 150 samples of iris flowers categorized into three species: Iris-setosa, Iris-versicolor, and Iris-virginica. Each sample has four features: sepal length, sepal width, petal length, and petal width.
Here’s how to load and explore the dataset:
from sklearn.datasets import load_iris
import pandas as pd
iris = load_iris()
X = pd.DataFrame(iris.data, columns=iris.feature_names)
y = iris.target
print(X.head())
print(f"Target classes: {iris.target_names}")
This dataset is commonly used to understand classification techniques like k-Nearest Neighbors and decision trees.
Loading Digits Data
The Digits dataset is another popular dataset, primarily used for image classification tasks. It contains 1,797 samples of handwritten digits (0–9), represented as 8×8 grayscale images. Each pixel value ranges from 0 to 16. This dataset is perfect for experimenting with algorithms like Support Vector Machines and Neural Networks.
Example:
from sklearn.datasets import load_digits
import matplotlib.pyplot as plt
digits = load_digits()
plt.imshow(digits.images[0], cmap='gray')
plt.title(f"Digit: {digits.target[0]}")
plt.show()
These datasets provide an excellent starting point for learning and experimenting with machine learning techniques.
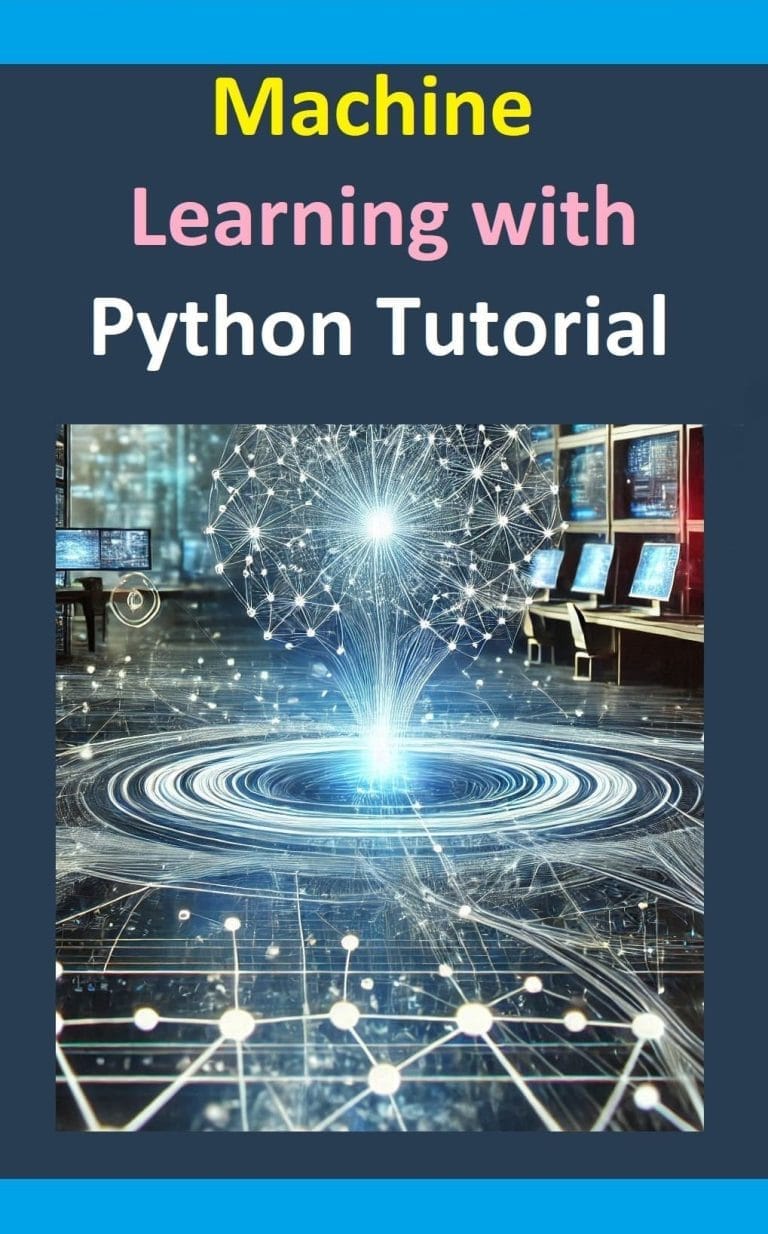
Key Algorithms in Machine Learning
k-Nearest Neighbor Classifier (k-NN)
The k-Nearest Neighbor (k-NN) algorithm is one of the simplest yet most effective methods for classification and regression. It works by finding the k closest data points (neighbors) to a query point and assigning the majority class (in classification) or averaging the labels (in regression). The k-NN method is non-parametric, meaning it makes no assumption about the data distribution, making it highly versatile.
When using k-NN, two key considerations are:
- Choice of k: The number of neighbors significantly impacts the model’s performance. A small k can lead to overfitting, while a large k may oversmooth the decision boundary.
- Distance Metric: Euclidean distance is commonly used, but other metrics like Manhattan or Minkowski can be applied depending on the dataset.
Here’s how to implement a k-NN classifier in Python using scikit-learn:
from sklearn.model_selection import train_test_split
from sklearn.neighbors import KNeighborsClassifier
from sklearn.metrics import accuracy_score
# Split data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train k-NN model
knn = KNeighborsClassifier(n_neighbors=3)
knn.fit(X_train, y_train)
# Evaluate the model
y_pred = knn.predict(X_test)
print(f"Accuracy: {accuracy_score(y_test, y_pred):.2f}")
The simplicity of k-NN makes it an excellent choice for quick prototyping. However, it can become computationally expensive for large datasets since predictions require calculating the distance to every training sample.
Neural Networks in Python: Structure, Weights, and Backpropagation
Neural networks are inspired by the human brain and consist of layers of interconnected neurons. These neurons process data by applying mathematical operations to learn patterns.
• Weights: Adjusted during training to minimize error by determining the importance of each connection between neurons. Weights are updated using optimization algorithms like gradient descent.
• Backpropagation: A technique to update weights based on the gradient of the loss function, which calculates the error between predicted and actual values. This process helps minimize the error over time.
Running a Neural Network in Python
Using TensorFlow to create and train a simple neural network:
import tensorflow as tf
model = tf.keras.Sequential([
tf.keras.layers.Dense(10, activation='relu'),
tf.keras.layers.Dense(3, activation='softmax')
])
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
model.fit(X_train, y_train, epochs=10)
Networks with Multiple Hidden Layers and Epochs
Adding more layers increases model complexity, while more epochs allow the model to learn better but can risk overfitting.
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(3, activation='softmax')
])
model.fit(X_train, y_train, epochs=50)
Advanced Algorithms in Machine Learning
Naive Bayes Classifier with Scikit-learn
A probabilistic model based on Bayes’ theorem, often used for text classification and spam detection. The algorithm is particularly effective for problems involving categorical data or text data, like email spam filters and sentiment analysis. Naive Bayes classifiers assume independence among features, making them computationally efficient. It is also highly scalable, making it useful for large datasets.
from sklearn.naive_bayes import GaussianNB
nb = GaussianNB()
nb.fit(X_train, y_train)
y_pred = nb.predict(X_test)
print(f"Accuracy: {accuracy_score(y_test, y_pred)}")
Regression Trees
Regression Trees predict continuous values by splitting data into regions. Each region corresponds to a specific value of the output variable. By making decisions at each node based on the feature that minimizes the error, regression trees help identify underlying trends in data and perform well for tasks like predicting house prices or stock prices. However, they can suffer from overfitting if not pruned effectively.
Building Regression Trees from Scratch
Using Python to implement a basic regression tree.
from sklearn.tree import DecisionTreeRegressor
reg_tree = DecisionTreeRegressor(max_depth=3)
reg_tree.fit(X_train, y_train)
print(f"Prediction: {reg_tree.predict(X_test[:5])}")
Regression Trees with scikit-learn
Scikit-learn offers a powerful implementation of regression trees, allowing for easy training and evaluation. By controlling parameters like maximum depth, you can avoid overfitting and ensure that the model generalizes well to unseen data. This implementation leverages decision trees to predict continuous values efficiently.
from sklearn.datasets import load_boston
boston = load_boston()
X, y = boston.data, boston.target
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
reg_tree = DecisionTreeRegressor(max_depth=4)
reg_tree.fit(X_train, y_train)
print(f"R^2 Score: {reg_tree.score(X_test, y_test)}")
Conclusion
Machine learning with Python offers endless opportunities to solve complex problems. This tutorial covered foundational concepts like machine learning terminology, working with datasets, implementing classifiers, neural networks, and regression trees. Python libraries like scikit-learn, TensorFlow, and others make implementing machine learning models efficient and scalable. By mastering these concepts and Python tools, you can build, train, and evaluate sophisticated machine learning models.
Download PDF: Machine Learning With Python Tutorial – For Beginners and Professionals