Machine learning, especially when implemented with Python, has become a cornerstone for predictive analysis, enabling businesses to make data-driven decisions. Predictive models analyze historical data to forecast future trends, solve business challenges, and improve processes.
To truly harness the potential of machine learning in Python, it’s crucial to focus on the right algorithms, data understanding, and model-building techniques. This article explores two essential algorithms for predictions, the importance of understanding the data, and the role of penalized linear regression in building predictive models that balance performance, complexity, and scalability.
Understanding the Problem by Understanding the Data
The success of any predictive model hinges on the quality and characteristics of the data being used. Data serves as the foundation upon which algorithms operate, making it imperative to deeply explore and understand it before diving into model selection or construction. A thorough examination of the dataset can reveal patterns, anomalies, and relationships that shape the choice of algorithms and preprocessing steps. Let’s explore the essential steps involved in understanding the data.
1. Descriptive Analysis
Descriptive analysis provides a summary of the dataset’s key attributes. This involves calculating measures like mean, median, mode, standard deviation, and variance for numerical variables. These metrics give an overview of the central tendencies and variability in the data, highlighting potential irregularities. Visualization techniques such as histograms and box plots further enhance understanding by showcasing data distribution and potential skewness. Descriptive statistics lay the groundwork for deeper exploration.
Example:
# Summary statistics
print(data.describe())
2. Identify Relationships
Understanding relationships between variables is crucial for predictive analysis. Correlation matrices help quantify the strength and direction of relationships between numerical variables, while scatter plots visually reveal trends and interactions. Strong correlations indicate potential predictors, whereas weak correlations may suggest irrelevant features. Identifying multicollinearity—a situation where independent variables are highly correlated—is also essential as it can distort model performance.
Example:
# Correlation matrix
sns.heatmap(data.corr(), annot=True)
plt.show()
3. Handle Missing Data
Missing data is a common challenge that can skew analysis and degrade model performance. It is crucial to address missing values using imputation techniques (mean, median, mode, or predictive methods) or by excluding affected rows or columns if appropriate. For large datasets, predictive imputation methods, such as regression or k-nearest neighbors (KNN), can help maintain dataset integrity. Handling missing values ensures a complete and accurate dataset for analysis.
Example:
# Fill missing values with mean
data.fillna(data.mean(), inplace=True)
4. Check for Outliers
Outliers are extreme values that deviate significantly from the dataset’s other observations. They can distort statistical measures and model outcomes. Identifying outliers through box plots, z-scores, or interquartile range (IQR) methods helps decide whether to remove or cap them based on their impact on analysis. Handling outliers appropriately enhances model robustness.
Example:
# Box plot to visualize outliers
sns.boxplot(data['feature'])
plt.show()
By following these steps, you can gain a deep understanding of your dataset, ensuring that the chosen algorithms and preprocessing techniques are well-suited to the problem at hand. Careful data exploration not only enhances model performance but also reduces the risk of errors in predictive analysis.
The Two Essential Algorithms for Making Predictions
Machine learning offers a wide range of algorithms for predictive analysis, each with unique strengths and applications. However, two algorithms – linear regression and penalized linear regression – stand out for their versatility, effectiveness, and foundational role in predictive modeling. These methods are particularly valuable in understanding and forecasting data trends.
1. Linear Regression
Linear regression is one of the simplest yet most powerful tools for predictive modeling, especially when the target variable is continuous. It works by modeling the relationship between the dependent variable (output) and one or more independent variables (inputs) using a straight-line equation.
Strengths:
- Simplicity: Linear regression is easy to implement and interpret, making it an excellent starting point for predictive analysis.
- Efficiency: It performs well with small to medium-sized datasets where relationships are linear.
- Insightful Coefficients: The coefficients indicate the magnitude and direction of influence each independent variable has on the dependent variable.
Challenges:
- Linearity Assumption: It assumes that relationships between variables are linear, which may not hold true in real-world scenarios.
- Sensitive to Multicollinearity: When independent variables are highly correlated, the model’s stability and accuracy can deteriorate.
- Underperformance in Complex Relationships: Linear regression struggles to capture non-linear patterns in data.
Example:
from sklearn.linear_model import LinearRegression
# Create and train the model
model = LinearRegression()
model.fit(X_train, y_train)
# Make predictions
predictions = model.predict(X_test)
Linear regression is particularly effective for straightforward problems, such as predicting house prices based on features like size and location.
2. Penalized Linear Regression (Ridge and Lasso)
Penalized linear regression extends the capabilities of standard linear regression by addressing its limitations, such as overfitting and multicollinearity. These techniques add a penalty term to the loss function, which constrains or regularizes the coefficient estimates.
Ridge Regression:
- Ridge regression applies an L2 penalty, which shrinks the coefficients toward zero but does not make them exactly zero.
- This method is ideal when all predictors are believed to contribute to the outcome but may suffer from multicollinearity.
- By reducing coefficient magnitudes, ridge regression prevents overfitting and enhances model generalization.
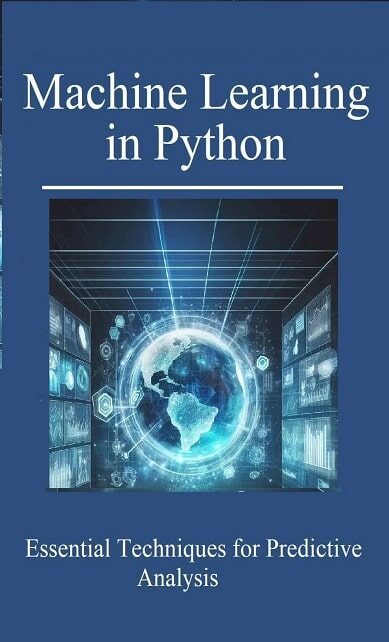
Lasso Regression:
- Lasso regression uses an L1 penalty, which forces some coefficients to become exactly zero.
- This approach is particularly useful for feature selection, as it automatically eliminates irrelevant or redundant features.
- Lasso is preferred when some predictors are expected to have minimal or no impact on the target variable.
Example:
from sklearn.linear_model import Ridge, Lasso
# Ridge Regression
ridge = Ridge(alpha=1.0)
ridge.fit(X_train, y_train)
# Lasso Regression
lasso = Lasso(alpha=0.1)
lasso.fit(X_train, y_train)
Penalized linear methods are powerful for handling high-dimensional datasets, ensuring interpretability, and improving predictive accuracy, especially in complex scenarios where standard linear regression falls short.
Predictive Model Building: Balancing Performance, Complexity, and Big Data
Building predictive models requires striking a balance between performance, complexity, and the challenges posed by big data. While overly complex models might fit the training data well, they risk overfitting, leading to poor generalization on new data. Conversely, overly simple models may fail to capture essential patterns, resulting in underfitting. Additionally, the exponential growth of data brings computational and storage challenges, making scalability a critical consideration for modern predictive modeling.
Steps for Effective Model Building
1. Feature Selection
Feature selection plays a pivotal role in simplifying models and improving their performance. By identifying the most influential features, predictive models can focus on the variables that contribute the most to the target outcome. Automated techniques, such as Lasso regression, inherently perform feature selection by shrinking irrelevant feature coefficients to zero. This reduces noise, enhances interpretability, and prevents overfitting, especially in high-dimensional datasets.
2. Hyperparameter Tuning
The performance of machine learning models heavily depends on hyperparameter tuning. Hyperparameters, unlike model parameters, are set before training and influence the model’s learning process. Effective tuning ensures that the model generalizes well to unseen data. Techniques like grid search and randomized search systematically explore the parameter space to find the optimal settings. For example, in Ridge or Lasso regression, adjusting the alpha parameter determines the level of regularization, balancing bias and variance for better predictions.
3. Cross-Validation
Cross-validation is essential to evaluate the robustness of a predictive model. Techniques like k-fold cross-validation divide the dataset into k subsets, using one subset for validation and the rest for training. This process is repeated k times, and the average performance across folds provides a reliable estimate of the model’s generalizability. Cross-validation prevents overfitting by ensuring the model is tested on multiple data splits, making it a crucial step in model development.
4. Scalability
The rise of big data necessitates scalable solutions for predictive modeling. Handling massive datasets efficiently requires distributed computing frameworks like Apache Spark and parallel processing libraries such as Dask. These tools enable processing large-scale data without sacrificing computational speed, ensuring models remain performant even as data volume grows. For Python users, integrating these frameworks is seamless, providing the scalability needed to manage real-world data challenges.
Example: Hyperparameter Tuning with Cross-Validation
from sklearn.model_selection import GridSearchCV
from sklearn.linear_model import Lasso
# Define the parameter grid
param_grid = {'alpha': [0.01, 0.1, 1, 10]}
lasso = Lasso()
# Perform grid search with cross-validation
grid_search = GridSearchCV(estimator=lasso, param_grid=param_grid, cv=5)
grid_search.fit(X_train, y_train)
print("Best Parameters:", grid_search.best_params_)
Balancing performance, complexity, and scalability ensures predictive models are both accurate and practical, paving the way for actionable insights.
Building Predictive Models Using Penalized Linear Methods
Penalized linear methods are crucial tools in machine learning, particularly when dealing with high-dimensional datasets or multicollinearity. These techniques modify standard linear regression by adding penalties to the cost function, discouraging overly complex models and reducing overfitting. By simplifying the model while retaining predictive power, penalized methods strike a balance between accuracy and interpretability.
Ridge Regression in Practice
Ridge regression is particularly effective in scenarios where multicollinearity – a high correlation between predictor variables – exists. In such cases, the coefficients in ordinary least squares regression become unstable, leading to unreliable predictions. Ridge regression addresses this by adding an L2 penalty, which imposes a constraint on the magnitude of the coefficients. This forces the algorithm to prioritize smaller coefficients, thereby stabilizing the model and reducing variance.
For example, consider a dataset with many highly correlated predictors. Ridge regression ensures that instead of assigning extreme values to coefficients, it distributes the weights more evenly. This helps in retaining all predictors’ contributions while ensuring the model remains robust.
Example Code:
from sklearn.linear_model import Ridge
ridge = Ridge(alpha=0.5) # L2 penalty
ridge.fit(X_train, y_train)
print("Ridge Coefficients:", ridge.coef_)
Lasso Regression in Practice
Lasso regression, on the other hand, is ideal for sparse datasets where only a subset of predictors significantly contributes to the outcome. By adding an L1 penalty, Lasso forces some coefficients to become exactly zero, effectively removing irrelevant features. This makes it a powerful tool for feature selection while simultaneously building the predictive model.
For instance, in a dataset with hundreds of variables, Lasso can automatically identify and retain only the most relevant predictors, eliminating noise and improving interpretability. This makes it particularly valuable for high-dimensional data with potential overfitting risks.
Example Code:
from sklearn.linear_model import Lasso
lasso = Lasso(alpha=0.1) # L1 penalty
lasso.fit(X_train, y_train)
print("Selected Features:", lasso.coef_)
Both ridge and Lasso regression methods enhance the reliability and efficiency of predictive models, especially in data-rich environments with numerous features. Their ability to handle complexity while maintaining simplicity makes them indispensable tools in predictive analytics.
Conclusion
Mastering predictive analysis requires a deep understanding of the data, algorithm selection, and model optimization. Penalized linear methods like Ridge and Lasso regression are indispensable tools for creating scalable and accurate models. By balancing performance, complexity, and data size, Python developers can unlock predictive insights to drive impactful decisions.
Download PDF: Machine Learning in Python
Leave a Reply