Logistic regression is a fundamental statistical technique widely used in predictive modeling and machine learning. It helps determine the probability of a binary outcome (such as success/failure, yes/no, or 0/1) based on one or more predictor variables.
Whether you’re new to data science or a professional looking to build predictive models, mastering logistic regression with R is an essential step toward understanding classification algorithms and binary outcome analysis. In this guide, we will explore logistic regression using R programming, one of the most powerful and accessible tools for statistical computing.
When to Use Logistic Regression?
You should use logistic regression when:
- Your target variable is binary (e.g., “pass” or “fail”)
- You want to understand the relationship between the dependent variable and one or more independent variables
- You need to predict the probability of a specific event occurring
Examples:
- Predicting whether a customer will buy a product (yes/no)
- Determining if an email is spam or not
- Assessing patient disease risk (present/absent)
Logistic Regression Syntax in R
In R, logistic regression is implemented using the glm() function with the family argument set to binomial.
Syntax:
model <- glm(formula = target ~ predictors, family = "binomial", data = dataset)
For example:
model <- glm(purchased ~ age + income, family = "binomial", data = marketing_data)
Step-by-Step Guide to Performing Logistic Regression in R
R provides a highly flexible and intuitive environment for building logistic regression models. Here’s a step-by-step overview of how to use logistic regression in R for binary classification.
Step 1: Loading the Data
You can either use in-built datasets like mtcars, Titanic, or load your own CSV data.
data <- read.csv("your_dataset.csv")
str(data)
Step 2: Exploratory Data Analysis (EDA)
Before modeling, perform data cleaning, visualizations, and summary statistics.
summary(data)
plot(data)
Step 3: Fitting a Logistic Regression Model
Use the glm() function with family = binomial to fit a logistic regression model.
model <- glm(target ~ predictor1 + predictor2, data = data, family = binomial)
summary(model)
Step 4: Interpreting the Model Output
- Coefficients: Indicate the log-odds change in the outcome for a one-unit increase in the predictor.
- Significance (p-values): Tell you whether the predictor is statistically significant.
- Odds Ratios: Can be derived by taking the exponent of the coefficient using exp(coef(model)).
Step 5: Model Diagnostics
Assess model performance using:
- Confusion Matrix
- ROC Curve and AUC
- Accuracy, Sensitivity, Specificity
library(caret)
pred <- predict(model, type = "response")
confusionMatrix(as.factor(pred > 0.5), as.factor(data$target))
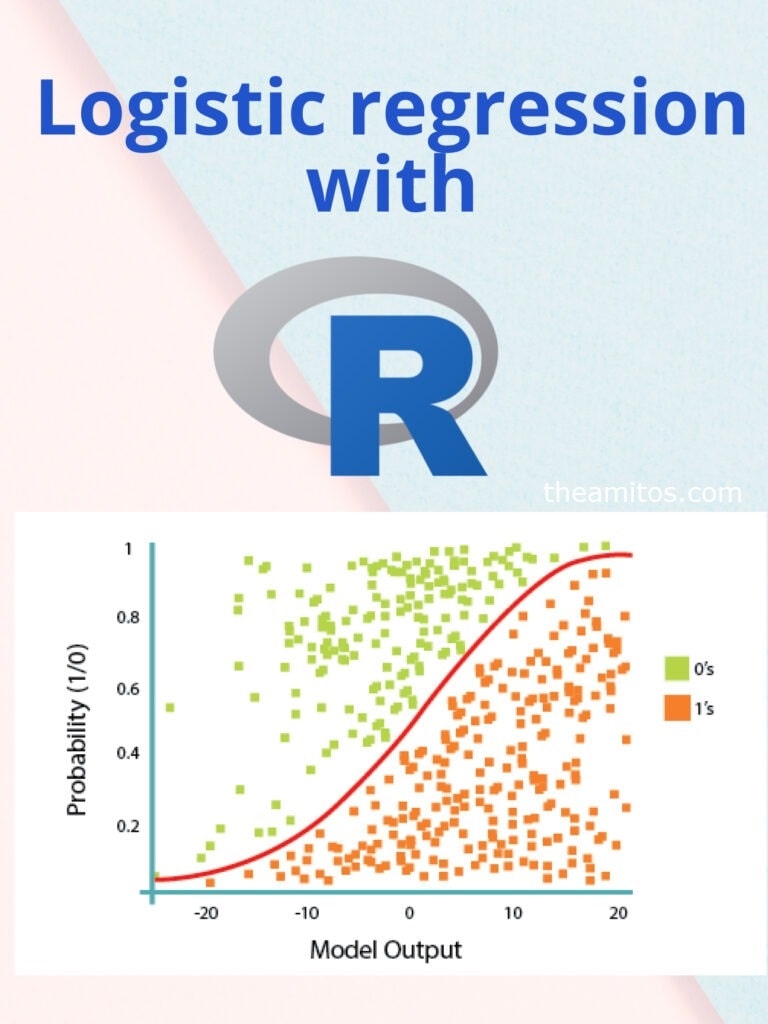
Advanced Topics in Logistic Regression with R
1. Multicollinearity
Check for multicollinearity using the Variance Inflation Factor (VIF).
library(car)
vif(model)
High VIF values indicate multicollinearity, which can be mitigated by removing or combining correlated variables.
2. Feature Selection
Use stepwise selection methods (forward, backward, or both) to choose the most predictive variables.
step(model, direction = "both")
3. Interaction Terms
Model interactions between predictors to capture more complex relationships.
model_interaction <- glm(target ~ predictor1 * predictor2, data = data, family = binomial)
4. Regularization Techniques
Use packages like glmnet for LASSO and Ridge regression, which help in variable selection and prevent overfitting.
library(glmnet)
x <- model.matrix(target ~ ., data)[,-1]
y <- data$target
fit <- glmnet(x, y, family = "binomial", alpha = 1)
Model Evaluation Metrics
To understand the efficiency of your logistic regression model in R, evaluate it using various metrics:
- Accuracy: Proportion of correct predictions.
- Precision and Recall: Relevant in imbalanced datasets.
- F1 Score: Harmonic mean of precision and recall.
- AUC-ROC: Represents classifier performance across thresholds.
library(pROC)
roc_obj <- roc(data$target, pred)
auc(roc_obj)
Visualizing Logistic Regression Results
Visualization improves the interpretability of the logistic regression model.
library(ggplot2)
ggplot(data, aes(x = predictor1, y = target)) +
geom_point() +
stat_smooth(method = "glm", method.args = list(family = "binomial"), se = FALSE)
Dealing with Imbalanced Datasets
In many real-world scenarios, one class may heavily outweigh the other. Strategies include:
- Oversampling the minority class (e.g., SMOTE)
- Undersampling the majority class
- Using class weights in modeling
library(DMwR)
balanced_data <- SMOTE(target ~ ., data = data, perc.over = 100, perc.under = 200)
Case Study: Predicting Heart Disease with Logistic Regression in R
Let’s say you are tasked with predicting heart disease presence using clinical parameters like age, cholesterol, and blood pressure. Using logistic regression, you can:
- Fit the model: Identify significant predictors.
- Generate predicted probabilities.
- Assess model accuracy using ROC and a confusion matrix.
- Communicate actionable insights to healthcare providers.
This process showcases how logistic regression with R can translate data into meaningful decisions.
Conclusion
Logistic regression with R is a powerful and intuitive method for binary classification problems. It forms the foundation for more advanced machine learning techniques and is widely used across industries. By learning how to implement, interpret, and evaluate logistic regression models in R, students and professionals can enhance their analytical capabilities and drive data-informed decisions.
Download PDF: Logistic Regression with R