Statistics plays a crucial role in data science, analytics, and decision-making. It helps uncover patterns in data, make predictions, and validate hypotheses. Python, with its extensive ecosystem of libraries, provides a powerful framework for statistical analysis. This guide explores fundamental statistical concepts, the tools available in Python for statistical analysis, and a step-by-step approach to learning statistics with Python.
Core Statistical Concepts for Data Analysis
Before diving into statistical analysis with Python, it’s essential to understand the fundamental statistical concepts. These concepts form the basis for analyzing and interpreting data effectively.
1. Descriptive Statistics
Descriptive statistics summarize and organize data, making it easier to interpret.
- Measures of Central Tendency:
- Mean (Average): The sum of all values divided by the number of observations.
- Median: The middle value in an ordered dataset, useful for skewed distributions.
- Mode: The most frequently occurring value in the dataset.
- Measures of Dispersion: These indicate the spread of data points.
- Range: The difference between the maximum and minimum values.
- Variance: Measures how far individual data points are from the mean.
- Standard Deviation: The square root of variance, indicating how much the data deviates from the mean.
- Distribution Shape: Understanding the shape of data distribution is important for statistical modeling.
- Skewness: Measures the asymmetry of data distribution. A positive skew indicates more values concentrated on the left, while a negative skew suggests more values on the right.
- Kurtosis: Determines whether a distribution has heavy or light tails compared to a normal distribution.
2. Probability Distributions
Probability distributions define how data values are likely to be distributed in a dataset. Common types include:
- Normal Distribution: Also known as a bell curve, this distribution is symmetrical, with most values clustering around the mean. It is widely used in statistical analysis and hypothesis testing.
- Binomial Distribution: Describes the probability of success or failure in a fixed number of trials. It is commonly used in scenarios like customer conversions or product defect rates.
- Poisson Distribution: Used to model the number of times an event occurs in a fixed interval, such as the number of website visits per hour or calls received at a call center.
3. Inferential Statistics
Inferential statistics allow analysts to make predictions and draw conclusions about a population based on sample data. Key techniques include:
- Hypothesis Testing: Determines whether an assumption about a dataset is statistically significant. Common tests include:
- T-tests: Compare means between two groups to see if differences are statistically significant.
- Chi-Square Test: Evaluates relationships between categorical variables.
- Analysis of Variance (ANOVA): Compares means across multiple groups.
- Confidence Intervals: Indicate the range within which a population parameter is likely to fall, typically with a 95% confidence level.
- Regression Analysis: Used to identify relationships between variables.
- Linear Regression: Determines the relationship between two numerical variables, often used in predictive modeling.
- Logistic Regression: Used when the outcome variable is categorical, such as predicting customer churn (yes/no) or disease presence (positive/negative).
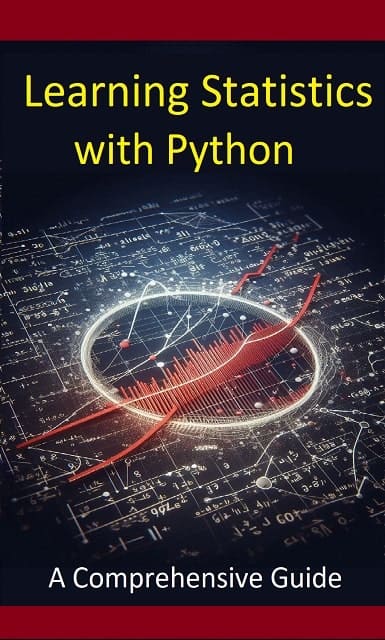
Python Libraries for Statistical Analysis
Python provides several powerful libraries that simplify statistical computations and data analysis.
1. NumPy (Numerical Python)
NumPy is a core library that provides efficient numerical operations, including statistical functions for mean, median, standard deviation, and variance.
2. Pandas (Data Analysis and Manipulation)
Pandas is widely used for handling structured data. It allows users to clean, transform, and analyze datasets efficiently using tabular structures like DataFrames.
3. SciPy (Scientific Computing)
SciPy extends NumPy by offering advanced statistical functions such as probability distributions, hypothesis testing, and optimization. It is commonly used in scientific and engineering applications.
4. Statsmodels (Statistical Modeling)
Statsmodels provides tools for statistical modeling, hypothesis testing, and regression analysis. It is particularly useful for econometrics and advanced statistical analysis.
5. Matplotlib & Seaborn (Data Visualization)
Data visualization is crucial for understanding statistical patterns. Matplotlib and Seaborn help create histograms, scatter plots, box plots, and heatmaps, making data interpretation easier.
Step-by-Step Guide to Learning Statistics with Python
Step 1: Install Required Libraries
To begin statistical analysis in Python, install the necessary libraries:
pip install numpy pandas scipy statsmodels seaborn matplotlib
Step 2: Import Libraries
Once installed, import these libraries into your Python script:
import numpy as np
import pandas as pd
import scipy.stats as stats
import statsmodels.api as sm
import seaborn as sns
import matplotlib.pyplot as plt
Step 3: Load and Explore Data
Using Pandas, you can load datasets and check the summary statistics:
df = pd.read_csv('data.csv')
print(df.describe())
Step 4: Perform Descriptive Statistics
Calculate mean, median, and standard deviation:
mean_value = np.mean(df['column_name'])
median_value = np.median(df['column_name'])
std_dev = np.std(df['column_name'])
Step 5: Hypothesis Testing
Conduct a t-test to compare means:
t_stat, p_value = stats.ttest_ind(df['group1'], df['group2'])
Step 6: Visualizing Data
Use Seaborn to create histograms and box plots:
sns.histplot(df['column_name'], bins=30, kde=True)
plt.show()
Challenges in Learning Statistics with Python
Despite Python’s simplicity, learning statistics can be challenging due to:
- Understanding Complex Statistical Terms: Concepts like p-values, confidence intervals, and effect sizes can be difficult for beginners.
- Choosing the Right Statistical Test: Selecting the appropriate test depends on the dataset and the hypothesis being tested.
- Handling Large and Noisy Datasets: Real-world data often contains missing values and inconsistencies, requiring careful preprocessing.
- Avoiding Overfitting in Predictive Models: Statistical models should be validated to ensure accuracy and reliability.
To overcome these challenges, consistent practice, working with real-world datasets, and referring to statistical textbooks and online courses are recommended.
Conclusion
Mastering statistics with Python is a valuable skill for anyone working in data analysis, research, or business intelligence. Python’s extensive libraries make statistical computations accessible, allowing analysts to draw meaningful insights from data.
By following a structured learning approach, applying statistical techniques to real-world scenarios, and continuously exploring new methodologies, professionals can enhance their data-driven decision-making capabilities and excel in their careers.