Computer vision has rapidly evolved into one of the most influential domains in the world of artificial intelligence, driving advancements in autonomous driving, medical imaging, face recognition, and more. At the heart of this innovation is OpenCV (Open Source Computer Vision Library), a powerful, open-source computer vision and machine learning software library. When combined with Python, it becomes an indispensable tool for building image processing algorithms and computer vision techniques.
In this article, we will explore how you can learn OpenCV with Python through hands-on exercises that are designed to build your understanding of image processing using OpenCV. Whether you’re new to computer vision or an experienced developer looking to sharpen your skills, this guide will provide you with the practical exercises you need to master computer vision with python.
Why Computer Vision with Python Using OpenCV?
OpenCV, combined with Python, offers a versatile and easy-to-learn approach to computer vision:
- Comprehensive Library: OpenCV provides a wide array of tools, functions, and methods to process images, recognize patterns, and develop object-detection algorithms.
- Python’s Simplicity: Python’s syntax is beginner-friendly and extremely intuitive, making it easier to implement complex computer vision tasks with fewer lines of code.
- Speed and Efficiency: While Python’s flexibility is great, OpenCV’s backend is highly optimized in C++, allowing it to process images efficiently even in real-time applications.
Setting Up Your Environment
Before diving into exercises, ensure that you have installed OpenCV and other necessary libraries. This can be easily done using pip, the Python package manager:
pip install opencv-python
pip install numpy
NumPy is crucial for handling multidimensional arrays, which are heavily used in image processing.
Exercise 1: Reading and Displaying Images with OpenCV
The first step in any image processing task is loading the image. With OpenCV, this task is a breeze.
import cv2
# Load an image
image = cv2.imread('image_path.jpg')
# Display the image in a window
cv2.imshow('Loaded Image', image)
# Wait for any key press to close the window
cv2.waitKey(0)
cv2.destroyAllWindows()
In this exercise, you will:
- Load an image using cv2.imread()
- Display the image using cv2.imshow()
This simple exercise helps you get familiar with OpenCV’s basic functionality for reading and displaying images. Experiment by loading various image formats (JPEG, PNG, etc.) to see how OpenCV handles them.
Exercise 2: Image Processing Using OpenCV  – Resizing, Rotating, and Cropping
Image manipulation is a core part of building algorithms in computer vision. Let’s try resizing, rotating, and cropping images using OpenCV.
Resizing:
# Resize the image to half its size
resized_image = cv2.resize(image, (int(image.shape[1] / 2), int(image.shape[0] / 2)))
cv2.imshow('Resized Image', resized_image)
cv2.waitKey(0)
Rotating:
# Rotate the image by 90 degrees
rotated_image = cv2.rotate(image, cv2.ROTATE_90_CLOCKWISE)
cv2.imshow('Rotated Image', rotated_image)
cv2.waitKey(0)
Cropping:
# Crop the image (top-left corner to a 200x200 size)
cropped_image = image[0:200, 0:200]
cv2.imshow('Cropped Image', cropped_image)
cv2.waitKey(0)
In this exercise, you will practice resizing images for different resolutions, rotating them for tasks like object recognition, and cropping areas of interest. These operations are frequently used in preprocessing images before feeding them into machine learning models.
Exercise 3: Color Spaces and Conversion
Color space conversion is critical in image processing for extracting relevant features. OpenCV allows you to switch between various color spaces such as RGB, HSV, and Grayscale.
# Convert to Grayscale
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
cv2.imshow('Grayscale Image', gray_image)
cv2.waitKey(0)
# Convert to HSV
hsv_image = cv2.cvtColor(image, cv2.COLOR_BGR2HSV)
cv2.imshow('HSV Image', hsv_image)
cv2.waitKey(0)
In this exercise, you will convert an image from one color space to another and visualize the results. Grayscale conversion is particularly useful for reducing computation in algorithms, while HSV is often used in color segmentation tasks.
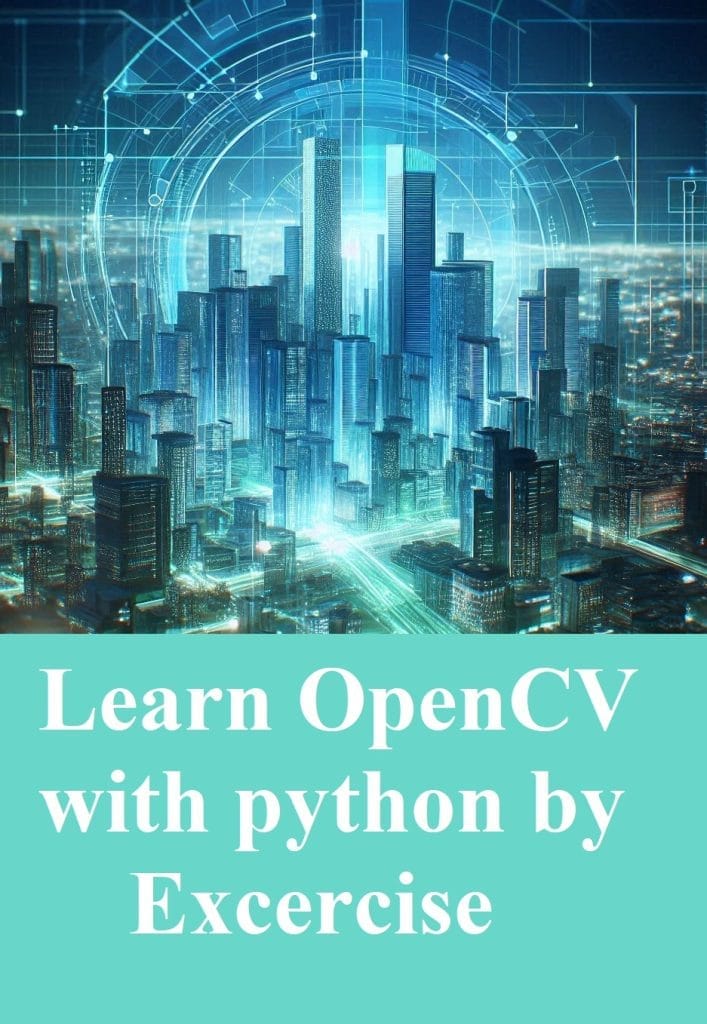
Exercise 4: Image Thresholding and Edge Detection
Thresholding and edge detection are key techniques in computer vision. Thresholding helps in image segmentation, and edge detection helps in detecting object boundaries.
Binary Thresholding:
# Apply binary thresholding
_, binary_image = cv2.threshold(gray_image, 127, 255, cv2.THRESH_BINARY)
cv2.imshow('Binary Threshold Image', binary_image)
cv2.waitKey(0)
Edge Detection with Canny:
# Perform Canny edge detection
edges = cv2.Canny(gray_image, 100, 200)
cv2.imshow('Edge Detection', edges)
cv2.waitKey(0)
This exercise introduces image segmentation and edge detection. By applying thresholding and using Canny edge detection, you can isolate significant features of an image that could later be used in object detection or image classification algorithms.
Exercise 5: Face Detection Using OpenCV
Haar cascades are one of the most popular methods for detecting faces and other objects. OpenCV comes with pre-trained classifiers for different objects such as faces, eyes, and smiles. In this exercise, we will use a Haar cascade to detect faces in an image.
# Load the cascade for face detection
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
# Convert the image to grayscale as the cascade works on grayscale images
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Detect faces
faces = face_cascade.detectMultiScale(gray, scaleFactor=1.1, minNeighbors=5)
# Draw rectangles around the faces
for (x, y, w, h) in faces:
cv2.rectangle(image, (x, y), (x+w, y+h), (255, 0, 0), 2)
cv2.imshow('Face Detection', image)
cv2.waitKey(0)
In this exercise, you will detect human faces in an image. This technique can be extended to detect other objects, and it is widely used in security systems and facial recognition applications.
Exercise 6: Real-Time Object Detection with a Webcam
One of the most practical applications of OpenCV is real-time video analysis. In this exercise, we will detect objects from the webcam feed in real time.
# Start the webcam feed
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
gray_frame = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# Detect edges in real-time
edges = cv2.Canny(gray_frame, 100, 200)
cv2.imshow('Real-Time Edge Detection', edges)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
This exercise focuses on capturing live video feed and processing each frame in real-time. We used edge detection as an example, but this concept can be extended to implement more complex algorithms such as object tracking, face detection, or gesture recognition.
Advanced Projects to Challenge Your Skills
Once you’ve completed the exercises above, it’s time to move on to more advanced projects. Here are a few suggestions:
- Building a Handwritten Digit Recognition System: Use OpenCV with a pre-trained neural network to recognize handwritten digits from the MNIST dataset.
- Autonomous Driving Simulations: Implement lane detection and traffic sign recognition algorithms to simulate a self-driving car.
- Augmented Reality: Build an AR application that overlays virtual objects on real-world surfaces by using marker-based tracking techniques in OpenCV.
- Medical Image Analysis: Use OpenCV to analyze medical images, such as MRI scans, for detecting abnormalities in tissues or organs.
Conclusion
Learning OpenCV with Python through exercises is an excellent way to master the fundamentals of computer vision and image processing. By working through these exercises, you’ll develop practical skills in image processing, object detection, and video analysis. These projects not only build technical expertise but also open doors to advanced computer vision applications such as autonomous vehicles, AI-based surveillance, and medical imaging.
Powerful image processing using OpenCV and computer vision with python, make them an unbeatable combination for building robust computer vision algorithms. As you progress, you can dive into more advanced topics, such as integrating deep learning models, building object tracking systems, and performing real-time video analysis.