Machine learning is reshaping industries by enabling systems to learn and improve from data without explicit programming. Python, with its simplicity and vast ecosystem of libraries, is the preferred language for implementing machine learning solutions. This article provides an introduction to machine learning with Python, covering essential topics such as supervised learning, unsupervised learning, and preprocessing, as well as techniques for model evaluation and improvement.
Supervised Learning: Understanding Core Concepts
Supervised learning is a machine learning approach where the algorithm learns from labeled data to make predictions. By analyzing the relationship between the inputs and outputs during training, supervised learning models can generalize to unseen data. It is widely used for tasks such as classification and regression.
Classification and Regression
- Classification: Classification assigns data points to predefined categories, such as distinguishing emails as spam or not spam. Algorithms like logistic regression, decision trees, and support vector machines are commonly used for classification tasks. Applications include sentiment analysis, fraud detection, and image recognition.
- Regression: Regression predicts continuous numeric values, such as housing prices or temperature trends. Techniques like linear regression and ridge regression model relationships between input features and target outputs. Regression finds applications in forecasting, resource allocation, and economic modeling..
Generalization, Overfitting, and Underfitting
- Generalization: Generalization refers to a model’s ability to perform well on unseen data, reflecting its robustness.
- Overfitting: Overfitting occurs when a model learns noise or irrelevant patterns in the training data, leading to poor performance on test data. This often results from excessively complex models.
- Underfitting: Underfitting happens when a model is too simplistic, failing to capture the underlying structure of the data. This leads to low accuracy on both training and test sets.
Achieving balance is crucial. Techniques like regularization (e.g., L1/L2 penalties) and cross-validation help mitigate these issues by optimizing model complexity and ensuring better generalization.
Uncertainty Estimates from Classifiers
In supervised learning, classifiers often provide probabilities alongside predictions, reflecting their confidence. For example, a logistic regression model might predict an email has an 85% likelihood of being spam and 15% of being legitimate. These probabilities are useful in decision-making processes, especially when the cost of incorrect predictions varies, such as in fraud detection or medical diagnosis.
The Decision Function
The decision function is the mathematical rule a model uses to separate different classes. For example, a support vector machine defines hyperplanes in feature space to divide classes. Visualizing decision boundaries is invaluable for understanding how the model operates and identifying areas of uncertainty or overlap between classes.
Predicting Probabilities and Uncertainty in Multiclass Classification
In multiclass classification tasks, models like random forests, logistic regression, or neural networks assign probabilities to each possible class. For instance, a model predicting customer preferences might assign 70% to “Product A,” 20% to “Product B,” and 10% to “Product C.” These probabilities provide insights into the model’s confidence and allow for informed decision-making. Tools like the softmax function are commonly used to normalize outputs in such tasks.
Unsupervised Learning and Preprocessing
Unsupervised learning identifies patterns in unlabeled data. It is often used for exploratory data analysis, clustering, and dimensionality reduction.
Types of Unsupervised Learning
1. Clustering
Clustering algorithms group similar data points based on shared characteristics. Techniques like k-means, hierarchical clustering, and DBSCAN are widely used to segment data into clusters, enabling tasks such as market segmentation, customer profiling, or anomaly detection. Clustering helps uncover inherent groupings in data that are not immediately apparent.
2. Dimensionality Reduction
Dimensionality reduction techniques, such as Principal Component Analysis (PCA) and t-SNE, reduce the number of features in a dataset while retaining its essential information. This simplifies datasets, enhances visualization, and improves the computational efficiency of machine learning algorithms, particularly when dealing with high-dimensional data.
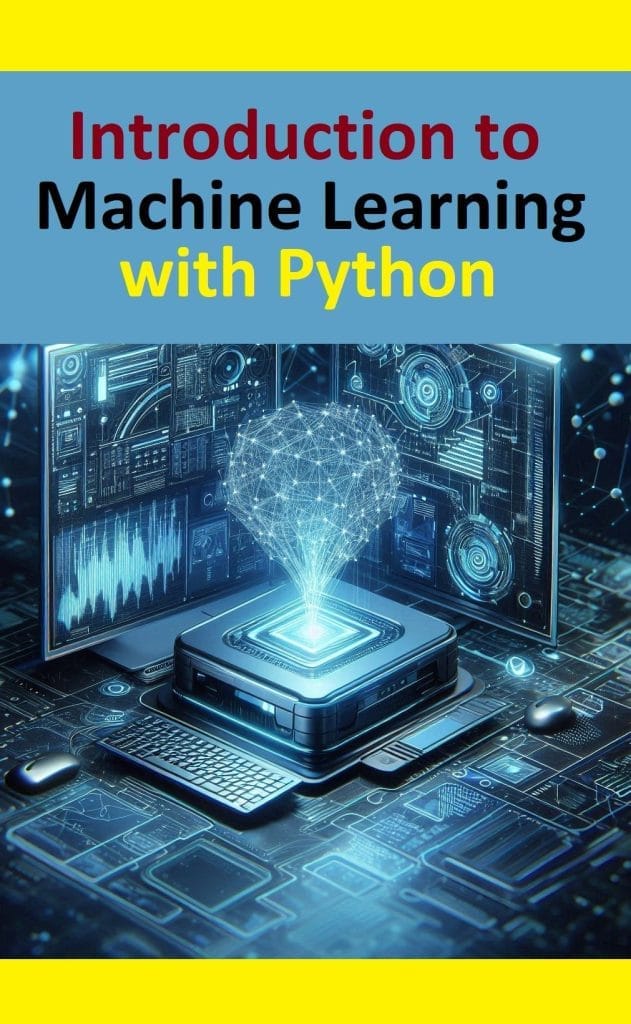
Challenges in Unsupervised Learning
One of the primary challenges in unsupervised learning is the absence of labeled data, which complicates the evaluation of model performance. Additionally, selecting the optimal number of clusters or components in techniques like k-means or PCA often requires domain expertise and iterative experimentation, adding to the complexity.
Preprocessing and Scaling
Preprocessing prepares raw data for machine learning algorithms, ensuring consistency and reliability in model outcomes.
- Scaling: Standardizing feature values ensures they are on a comparable scale, which is critical for algorithms sensitive to differences in feature magnitudes, such as k-means clustering or support vector machines.
- Encoding Categorical Data: Transforming categorical variables into numerical representations, such as through one-hot encoding or label encoding, ensures they are machine-readable without introducing biases.
Model Evaluation and Improvement
Evaluating and refining machine learning models is critical to achieving reliable results. Python provides powerful tools to assess and optimize model performance.
Cross-Validation
Cross-validation splits the dataset into multiple subsets to train and validate the model on different data portions. Popular strategies include:
- K-Fold Cross-Validation: Divides data into k subsets and trains the model k times, using a different subset for validation each time.
- Leave-One-Out Cross-Validation (LOOCV): Uses all but one data point for training, repeating this process for every data point.
Cross-validation helps detect overfitting and ensures the model generalizes well.
Grid Search
Grid search automates hyperparameter tuning by testing all possible combinations of specified parameter values. For example, to optimize a support vector machine, you might search through combinations of kernel types, regularization parameters, and gamma values.
Example:
from sklearn.model_selection import GridSearchCV
from sklearn.svm import SVC
param_grid = {
'C': [0.1, 1, 10],
'kernel': ['linear', 'rbf'],
'gamma': [1, 0.1, 0.01]
}
grid = GridSearchCV(SVC(), param_grid, cv=5)
grid.fit(X_train, y_train)
print(f"Best parameters: {grid.best_params_}")
Evaluation Metrics and Scoring
Choosing the right evaluation metric depends on the task:
- Classification:
- Accuracy: Proportion of correct predictions.
- Precision and Recall: Useful for imbalanced datasets.
- F1 Score: Harmonic mean of precision and recall.
- ROC-AUC: Measures model performance across different classification thresholds.
- Regression:
- Mean Squared Error (MSE): Measures average squared error between predicted and actual values.
- R² Score: Indicates the proportion of variance explained by the model.
Scikit-learn provides functions to calculate these metrics easily.
Practical Example: End-to-End Workflow
To illustrate the concepts discussed earlier, let’s implement a complete machine learning workflow in Python. In this example, we’ll use supervised learning to classify flowers in the famous Iris dataset, a classic dataset in machine learning. It contains features like petal and sepal dimensions to predict flower species.
Step 1: Import Libraries
Before working with the dataset, import the necessary libraries. These include tools for data loading, splitting, preprocessing, model training, and evaluation.
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split, GridSearchCV
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import classification_report
These libraries provide essential functions for handling datasets, applying machine learning models, optimizing hyperparameters, and evaluating model performance.
Step 2: Load and Split Data
Load the Iris dataset, which consists of 150 samples with four features each. Split it into training and testing subsets to evaluate model generalization.
data = load_iris()
X = data.data
y = data.target
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
This step ensures the model is trained on one portion of the data and tested on another for unbiased performance evaluation.
Step 3: Preprocess Data
Standardize the feature values to ensure all dimensions have comparable scales, improving the performance of algorithms sensitive to feature magnitudes.
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
Scaling helps machine learning algorithms converge faster and achieve better accuracy, especially for distance-based methods.
Step 4: Train and Tune the Model
Build a Random Forest Classifier, a robust ensemble method. Use GridSearchCV to find the optimal hyperparameters for the number of trees and maximum depth.
param_grid = {'n_estimators': [10, 50, 100], 'max_depth': [None, 10, 20]}
grid = GridSearchCV(RandomForestClassifier(), param_grid, cv=5)
grid.fit(X_train, y_train)
GridSearchCV automates hyperparameter tuning by evaluating different parameter combinations to find the best-performing model.
Step 5: Evaluating Machine Learning Models
Use the optimized model to predict test set labels and evaluate its performance using a detailed classification report, including metrics like precision, recall, and F1-score.
y_pred = grid.best_estimator_.predict(X_test)
print(classification_report(y_test, y_pred))
This evaluation provides insights into the model’s effectiveness across all classes, highlighting areas of strength and potential improvement.
Conclusion
Machine learning with Python opens doors to innovative solutions in various domains. By understanding supervised learning, unsupervised learning, preprocessing, and model evaluation, you can build robust machine learning models. Python’s extensive libraries and tools simplify these processes, making it accessible for both beginners and professionals.