Geographic Data Science (GDS) is an essential discipline for analyzing spatial data to uncover patterns, relationships, and trends in various fields, from urban planning to environmental monitoring. Python, with its vast ecosystem of libraries, has become the popular programming language for Geographic Data Science due to its flexibility and power.
This article explores key aspects of Geographic Data Science with Python, focusing on core data structures in Geographic Data Science, spatial data concepts, core analysis techniques, and advanced topics. These insights will provide a comprehensive understanding of how Python empowers professionals in the geospatial domain.
Core Data Structures in Geographic Data Science
In Geographic Data Science, spatial data is commonly organized into three primary data structures: geographic tables, surfaces, and spatial graphs.
1. Geographic Tables
Geographic tables are structured datasets where each row represents a geographic entity (e.g., a country, city, or postal code), and columns hold attributes such as population, area, or GDP. These datasets are often stored as geospatial files like shapefiles or GeoJSON, providing an efficient way to represent both spatial and non-spatial data attributes. Python libraries like Geopandas simplify data manipulation, visualization, and spatial analysis tasks.
Example in Python:
import geopandas as gpd
# Load a shapefile as a GeoDataFrame
data = gpd.read_file("path_to_shapefile.shp")
print(data.head())
2. Surfaces datasets
Surfaces represent continuous phenomena, such as elevation, temperature, or rainfall, across geographic spaces. These datasets, typically stored in raster formats, capture grid-based data where each cell has a specific value corresponding to the phenomenon. Surfaces are integral to environmental modeling, terrain analysis, and resource distribution studies, and Python’s Rasterio library provides tools to process and analyze such data.
Example:
import rasterio
# Open a raster dataset
with rasterio.open("path_to_raster.tif") as dataset:
print(dataset.read(1)) # Read the first band
3. Spatial Graphs
Spatial graphs model relationships between geographic entities, such as road networks, social connections, or utilities. Nodes represent entities like intersections or cities, while edges denote connections like roads or pipelines. These graphs are essential for network analysis, route optimization, and connectivity studies. Python’s NetworkX library enables efficient creation, visualization, and analysis of spatial graphs, making it a vital tool for Geographic Data Science.
Example:
import networkx as nx
# Create a spatial graph
G = nx.Graph()
G.add_edge("Location A", "Location B", weight=5)
print(G.edges(data=True))
Spatial Data and Spatial Weights
Understanding Spatial Data
Spatial data includes any data that has a geographic or locational component. It can be vector-based (points, lines, polygons) or raster-based (grids, surfaces). Handling spatial data in Python involves loading, manipulating, and analyzing geographic datasets using libraries like Geopandas, Fiona, and Shapely.
Spatial Weights
Spatial weights quantify the relationship between spatial entities. They are essential for spatial statistics, where the proximity or interaction between locations is a critical factor. Spatial weights can be contiguity-based (e.g., shared borders) or distance-based. Python’s PySAL library is a powerful tool for constructing spatial weights matrices.
Example:
from libpysal.weights import Queen
# Create a spatial weights matrix
weights = Queen.from_dataframe(data)
print(weights.neighbors)
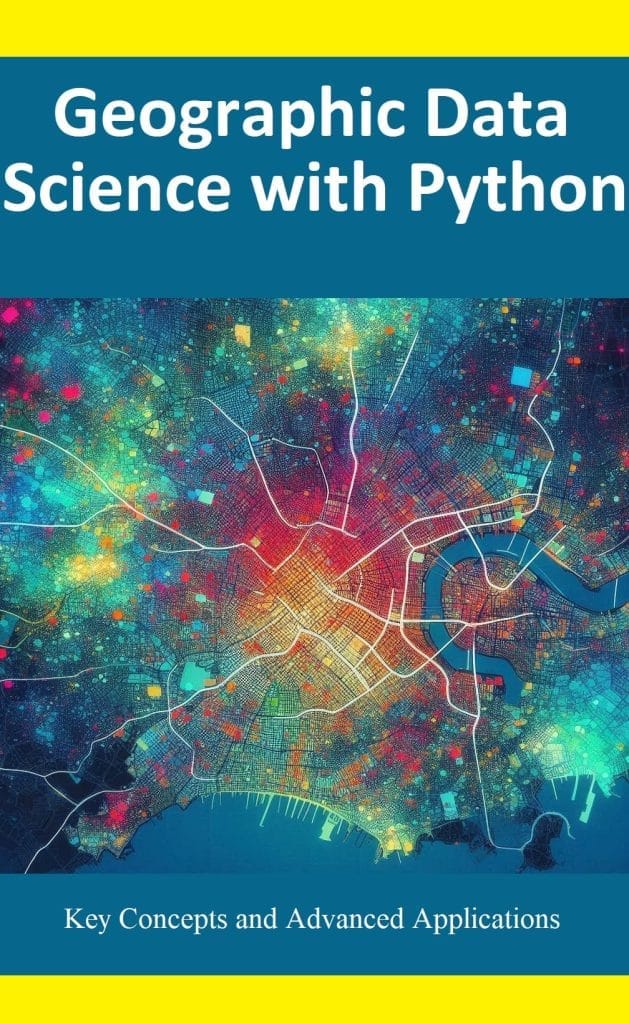
Spatial Data Analysis
Spatial data analysis involves applying techniques to uncover patterns and relationships in spatial data. Key methods include choropleth mapping, global and local spatial autocorrelation, and point pattern analysis.
1. Choropleth Mapping
By shading geographic regions based on attribute values, they help highlight patterns such as population density, income distribution, or disease prevalence. These maps are highly effective for identifying spatial disparities and trends, making them a staple in Geographic Data Science. Python’s Geopandas library simplifies the creation of choropleth maps with customizable color schemes and legends.
Example:
data.plot(column="population_density", cmap="OrRd", legend=True)
2. Global Spatial Autocorrelation
Global spatial autocorrelation assesses whether spatial data exhibits a clustered, dispersed, or random pattern across a geographic space. Moran’s I is a widely used statistic that ranges from -1 (perfect dispersion) to +1 (perfect clustering). A value near zero suggests a random spatial distribution. Calculating Moran’s I with PySAL provides insights into overall spatial relationships and dependencies.
Example:
from esda.moran import Moran
moran = Moran(data["population_density"], weights)
print(moran.I) # Moran's I value
3. Local Spatial Autocorrelation
While global measures summarize overall spatial relationships, local spatial autocorrelation reveals specific clusters or outliers within the dataset. Tools like Local Moran’s I identify hot spots (regions with high attribute values surrounded by similar values) and cold spots (low-value regions in similar contexts). These insights are crucial for targeting interventions in public health, urban development, or market expansion.
Example:
from esda.moran import Moran_Local
local_moran = Moran_Local(data["income"], weights)
data["local_moran"] = local_moran.Is
4. Point Pattern Analysis
Point pattern analysis focuses on the distribution of individual events or features, such as crime incidents, retail locations, or wildlife sightings. This method examines whether points are randomly distributed, clustered, or evenly spaced. Tools like Poisson Point Process in PySAL model the spatial intensity of events, helping researchers detect patterns and predict future occurrences.
Example:
from pointpats import PoissonPointProcess
# Perform point pattern analysis
process = PoissonPointProcess(data.geometry, data.crs, intensity=0.01)
print(process.complete)
Advanced Topics in Geographic Data Science
1. Spatial Inequality Dynamics
Spatial inequality dynamics analyze disparities in resource distribution, infrastructure, or economic opportunities across regions. These analyses help policymakers address socio-economic imbalances.
Example:
Using spatial Gini coefficients to measure inequality across regions.
2. Clustering and Regionalization
Spatial clustering groups regions with similar characteristics, while regionalization identifies contiguous areas with shared attributes. Python libraries like PySAL and Scikit-learn are used for clustering.
Example:
from sklearn.cluster import KMeans
# Apply clustering
kmeans = KMeans(n_clusters=5)
data["clusters"] = kmeans.fit_predict(data[["income", "population_density"]])
3. Spatial Regression
Spatial regression models account for spatial dependencies in data. These models are crucial for accurate predictions and policy impact assessments. PySAL provides tools for spatial econometrics.
Example:
from spreg import OLS
# Fit a spatial regression model
model = OLS(data[["income"]], data[["education", "employment"]])
print(model.summary)
4. Spatial Feature Engineering
Spatial feature engineering involves creating new features from raw spatial data to improve machine learning model performance. Examples include proximity measures, spatial interactions, and landscape metrics.
Example:
data["distance_to_city"] = data.geometry.distance(city_center.geometry)
Conclusion
Geographic Data Science with Python is a transformative field for analyzing spatial data to solve real-world challenges. From fundamental concepts like geographic tables and spatial weights to advanced techniques such as clustering, spatial regression, and feature engineering, Python equips professionals with the tools needed to extract meaningful insights.
By mastering these methods and leveraging Python’s extensive libraries, you can harness the power of Geographic Data Science to drive innovation and make informed decisions across diverse applications.
Download PDF: Geographic Data Science with Python – Key Concepts and Advanced Application