The rise of artificial intelligence (AI) and machine learning (ML) has transformed the way businesses and individuals interact with technology. One of the most significant developments in this space is the chatbot. In this article, we will explore the key steps to building chatbots with Python, focusing on Natural Language Processing (NLP) and Machine Learning (ML). We will cover essential tools, methodologies, and best practices, ensuring that you can create a powerful chatbot for your business or personal project.
Fundamentals of Building a Chatbot
Building an intelligent chatbot requires a combination of Natural Language Processing (NLP), Machine Learning (ML), and Python programming. These elements work together to enable a chatbot to understand, process, and respond to user queries effectively.
- Natural Language Processing (NLP) – NLP allows chatbots to interpret and analyze human language by performing text preprocessing, entity recognition, and sentiment analysis.
- Machine Learning (ML) Algorithms – ML enables chatbots to learn from interactions, improving their responses over time through supervised or unsupervised learning techniques.
- Database and API Integration – Chatbots access databases or external APIs to fetch real-time information, such as product details or weather updates.
- User Interface (UI) – Chatbots can be deployed on messaging platforms, websites, or mobile apps, enhancing user engagement.
Python’s robust ecosystem, including NLTK, spaCy, TensorFlow, and ChatterBot, simplifies chatbot development and ensures efficient performance.
Step-by-Step Guide to Building a Chatbot with Python
Building an intelligent chatbot requires a structured approach, starting from setting up the environment to choosing the right model. Below is a detailed step-by-step guide to chatbot development using Python.
1. Setting Up the Development Environment
To build a chatbot, the first step is installing Python (preferably version 3.7+) and essential libraries. Python offers a robust ecosystem for NLP and machine learning, making it the preferred language for chatbot development. Key libraries include:
- NLTK (Natural Language Toolkit) – For NLP tasks like tokenization and stemming.
- spaCy – An advanced NLP library for faster and more efficient text processing.
- TensorFlow & Keras – To build deep learning-based AI chatbots.
- ChatterBot – For creating simple rule-based chatbots.
To install these, run:
pip install nltk spacy tensorflow keras chatterbot chatterbot_corpus
This ensures all required dependencies are available before proceeding with chatbot development.
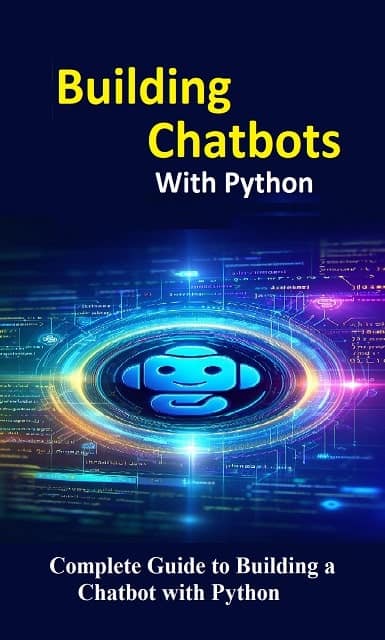
2. Understanding Natural Language Processing (NLP)
Natural Language Processing (NLP) is the key to enabling a chatbot to understand and respond to human language. It includes several techniques:
- Tokenization – Splitting text into individual words or sentences for processing.
- Lemmatization & Stemming – Converting words to their root forms (e.g., “running” into “run”).
- Named Entity Recognition (NER) – Extracting meaningful entities like names, locations, and dates.
- Sentiment Analysis – Determining if a sentence expresses positive, negative, or neutral sentiment.
Libraries like NLTK and spaCy simplify NLP implementation, making it easier to preprocess and analyze textual input for chatbots.
3. Choosing the Right Chatbot Type
Before building a chatbot, decide on its type:
- Rule-based Chatbots – These use predefined patterns and keyword-based responses. They are simple but limited in handling unpredictable conversations.
- AI-powered Chatbots – These utilize Machine Learning and NLP to generate intelligent, context-aware responses by learning from past conversations.
For more advanced AI chatbots, deep learning models like Long Short-Term Memory (LSTM) networks and Transformers (e.g., BERT, GPT) provide state-of-the-art performance.
Building a Rule-Based Chatbot
A rule-based chatbot operates on pattern recognition and predefined rules. It responds based on a structured conversation flow, making it useful for answering FAQs and guiding users through simple queries. The ChatterBot library in Python provides an easy way to implement such chatbots.
Implementing a Basic Chatbot Using ChatterBot
The following example demonstrates how to create a simple chatbot using ChatterBot and train it with an English-language dataset:
from chatterbot import ChatBot
from chatterbot.trainers import ChatterBotCorpusTrainer
chatbot = ChatBot("SimpleBot")
trainer = ChatterBotCorpusTrainer(chatbot)
trainer.train("chatterbot.corpus.english")
response = chatbot.get_response("Hello!")
print(response)
This chatbot can respond to basic greetings and common conversational inputs. However, since it follows predefined patterns, it struggles with dynamic, open-ended conversations.
While rule-based chatbots are useful for simple tasks, AI-powered chatbots offer greater flexibility and improved user interaction by continuously learning from conversations.
Building an AI-Powered Chatbot Using NLP & Machine Learning
To build a chatbot with NLP and ML, we follow these steps:
1. Data Collection and Preprocessing
For an ML-based chatbot, we need training data. This could be:
- Custom datasets – A dataset of conversations related to a specific domain.
- Public datasets – Kaggle and other sources provide chatbot datasets.
2. Text Preprocessing
Before training an ML model, we preprocess the text:
import nltk
from nltk.tokenize import word_tokenize
from nltk.corpus import stopwords
import string
nltk.download('punkt')
nltk.download('stopwords')
def preprocess_text(text):
text = text.lower()
tokens = word_tokenize(text)
tokens = [word for word in tokens if word not in stopwords.words('english') and word not in string.punctuation]
return tokens
sample_text = "Hello! How can I assist you today?"
print(preprocess_text(sample_text))
This removes stopwords, and punctuation, and tokenizes the text for further processing.
3. Training a Chatbot Using Deep Learning
Deep learning models like Recurrent Neural Networks (RNNs), Long Short-Term Memory (LSTMs), and Transformers are commonly used for chatbots.
Implementing an AI Chatbot Using TensorFlow & Keras
We use TensorFlow and Keras to build and train a neural network for our chatbot.
Model Architecture:
- Convert text data into numerical form using word embeddings (Word2Vec, TF-IDF).
- Train an LSTM-based neural network for context-aware responses.
- Fine-tune using real-world conversations.
A chatbot trained with Deep Learning improves over time and can generate more intelligent responses than rule-based systems.
Deploying the Chatbot
Once trained, a chatbot must be deployed to make it accessible to users. The deployment method depends on the intended use case and target audience.
- Web Applications – Chatbots can be embedded in websites using Flask or Django, allowing businesses to offer automated assistance through a web interface.
- Messaging Platforms – Many businesses integrate chatbots with WhatsApp, Telegram, and Facebook Messenger to engage customers through popular messaging apps.
- Voice Assistants – AI chatbots can be deployed with Google Assistant or Alexa, enabling voice interactions for a hands-free experience.
Proper deployment ensures seamless communication, enhancing customer satisfaction and business efficiency.
Future of Chatbots
With advancements in AI, chatbots are becoming more sophisticated and human-like, enhancing user experience and business efficiency.
- GPT-powered chatbots – Leveraging advanced AI models like GPT-4, these chatbots can generate context-aware, near-human responses, making interactions more fluid and engaging.
- Voice-enabled chatbots – Integrating speech recognition, these chatbots enable hands-free interactions, improving accessibility and convenience.
- Emotionally intelligent chatbots – Using sentiment analysis and emotional AI, these bots detect user emotions and respond empathetically, fostering deeper engagement and personalized support.
Conclusion
Building a chatbot using Python, NLP, and Machine Learning is a rewarding experience. Whether you develop a rule-based chatbot or an AI-driven conversational agent, Python’s rich ecosystem of libraries and frameworks makes chatbot development more efficient. As AI continues to evolve, chatbots will play an even bigger role in customer service, business automation, and human-computer interaction.
Wonderful book