In today’s data-driven world, businesses and organizations heavily rely on data analytics to make informed decisions. Python has emerged as the leading programming language for data analysis due to its simplicity, versatility, and powerful libraries. Among the most popular libraries used for data analytics are Pandas, Matplotlib, and NumPy. These tools enable data scientists and analysts to manipulate, visualize, and analyze large datasets efficiently.
This comprehensive guide explores how Python data analytics empowers users to extract meaningful insights, visualize trends, and make data-driven decisions. Whether you’re a beginner or an experienced analyst, this article will help you understand the fundamentals of data science using Python and how to use its essential libraries effectively.
Understanding Pandas: The Backbone of Python Data Analysis
What is Pandas?
Pandas is a powerful data manipulation and analysis library in Python. It is built on NumPy and provides data structures like Series and DataFrames, which make handling structured data easy and intuitive.
Key Features of Pandas:
- Data Cleaning and Preprocessing: Handle missing values, duplicates, and data formatting.
- Data Transformation: Perform filtering, grouping, and aggregation operations.
- Handling Large Datasets: Efficiently manage big data with optimized performance.
- Integration with Other Libraries: Works seamlessly with Matplotlib, NumPy, and Scikit-Learn.
Essential Pandas Functions for Data Analysis
- Reading Data
- Load data from CSV, Excel, SQL databases, and JSON files.
- Data Cleaning and Preprocessing
- Handling missing values using fillna() and dropna().
- Removing duplicates with drop_duplicates().
- Formatting data with astype().
- Data Manipulation
- Filtering data using conditional selection.
- Aggregating data with groupby().
- Sorting and ranking data with sort_values().
- Merging and Concatenation
- Combine datasets using merge() and concat().
By mastering Pandas for data science, analysts can manipulate complex datasets and derive valuable insights.
Visualizing Data with Matplotlib: The Power of Data Visualization
What is Matplotlib?
Matplotlib is a widely used data visualization library in Python. It enables users to create static, animated, and interactive visualizations to understand trends, distributions, and correlations in data.
Why is Data Visualization Important?
- Helps in identifying trends and patterns in datasets.
- Simplifies complex data, making it easier to interpret.
- Enhances communication of analytical results through graphical representation.
Types of Plots in Matplotlib
- Line Charts – Useful for tracking changes over time.
- Bar Charts – Ideal for comparing categories.
- Histograms – Display frequency distributions.
- Scatter Plots – Show relationships between two variables.
- Pie Charts – Represent proportions and percentages.
With Matplotlib for data visualization, analysts can create compelling charts and graphs that make their findings easier to understand.
The Role of NumPy in Data Analytics
While Pandas is excellent for handling structured data, NumPy (Numerical Python) provides support for numerical computing and high-performance mathematical operations.
Key Features of NumPy:
- Efficient array handling with ndarray.
- Mathematical operations like mean, median, and standard deviation.
- Support for linear algebra and statistical analysis.
- Fast computations with vectorized operations.
Using NumPy for data analysis, Python users can handle large numerical datasets efficiently.
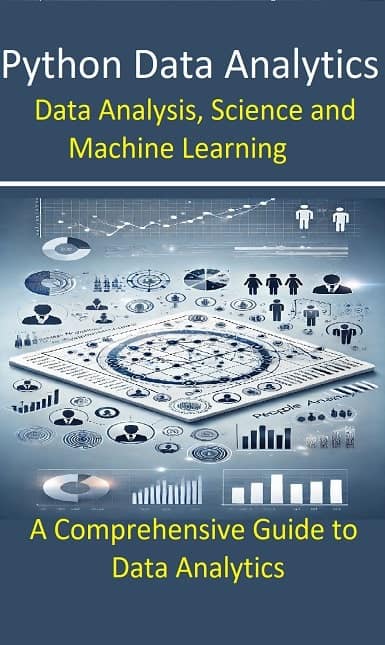
Python Data Analytics Workflow: From Raw Data to Insights
To perform data analysis using Python, follow this step-by-step workflow:
Step 1: Import Necessary Libraries
Start by importing essential Python libraries:
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
Step 2: Load the Dataset
Read the dataset into a Pandas DataFrame:
df = pd.read_csv('data.csv')
Step 3: Explore and Clean the Data
Check for missing values and remove unnecessary columns:
df.info()
df.dropna(inplace=True)
Step 4: Perform Data Analysis
Compute descriptive statistics and analyze trends:
df.describe()
df.groupby('Category')['Sales'].sum()
Step 5: Visualize Data
Create a bar chart for sales distribution:
df['Sales'].hist(bins=10)
plt.show()
Following this Python data analytics workflow, analysts can efficiently transform raw data into meaningful insights.
Machine Learning with Scikit-Learn
What is Scikit-Learn?
Scikit-Learn is the most popular machine learning library in Python. It provides tools for supervised and unsupervised learning, data preprocessing, and model evaluation.
Key Features of Scikit-Learn
Scikit-Learn is packed with features that make it a preferred choice for machine learning and data analysis. Some of its most significant functionalities include:
1. Built-in Machine Learning Algorithms
Scikit-Learn offers a wide range of machine learning models for different types of tasks:
- Supervised Learning: Supports classification (e.g., Logistic Regression, Decision Trees, Random Forests) and regression (e.g., Linear Regression, Ridge Regression).
- Unsupervised Learning: Includes clustering algorithms like K-Means, DBSCAN, and hierarchical clustering.
- Dimensionality Reduction: Implements techniques like Principal Component Analysis (PCA) and t-SNE to reduce feature space.
2. Data Preprocessing and Feature Engineering
Data preprocessing is an essential step in machine learning, and Scikit-Learn provides several tools to prepare data before training models:
- Handling Missing Data: Functions like SimpleImputer help fill in missing values.
- Feature Scaling: Standardization and normalization using StandardScaler and MinMaxScaler.
- Encoding Categorical Variables: Converting categorical data into numerical format using OneHotEncoder and LabelEncoder.
- Feature Selection: Identifies the most important variables using techniques like Recursive Feature Elimination (RFE).
3. Model Selection and Evaluation
Scikit-Learn includes comprehensive tools to evaluate machine learning models and select the best-performing ones:
- Model Validation: Provides train-test splitting, cross-validation, and bootstrapping.
- Performance Metrics: Includes accuracy, precision, recall, F1-score, and ROC-AUC for classification models.
- Hyperparameter Tuning: Automates parameter optimization using GridSearchCV and RandomizedSearchCV.
With these features, Scikit-Learn allows developers to build high-performing models efficiently, making it a cornerstone of Python-based machine learning workflows.
Expanding to Other Machine Learning Models
Scikit-Learn is not limited to just linear regression; it supports a wide range of machine learning algorithms for different types of tasks:
1. Classification Algorithms
Used for tasks where the output is categorical (e.g., spam detection, disease prediction):
- Logistic Regression – Suitable for binary classification problems.
- Decision Trees – Creates decision rules to classify data.
- Random Forest – An ensemble method that improves accuracy by combining multiple decision trees.
2. Regression Algorithms
Used for predicting continuous values (e.g., stock prices, sales forecasting):
- Linear Regression – Models relationships between variables using a straight-line equation.
- Ridge Regression – A regularized version of linear regression to prevent overfitting.
3. Clustering Algorithms
Used for grouping similar data points together:
- K-Means Clustering – Partitions data into clusters based on feature similarity.
- DBSCAN (Density-Based Spatial Clustering) – Groups data points based on density.
4. Dimensionality Reduction Techniques
Used to reduce dataset complexity while preserving essential features:
- Principal Component Analysis (PCA) – Reduces high-dimensional data into fewer components.
- t-SNE (t-Distributed Stochastic Neighbor Embedding) – A visualization-friendly dimensionality reduction technique.
Conclusion
Python has become the industry standard for data analytics due to its flexibility, powerful libraries, and ease of use. By leveraging Pandas for data manipulation, Matplotlib for data visualization, and NumPy for numerical computing, professionals can extract insights, visualize patterns, and make data-driven decisions effectively.