The modern world is powered by data, data science and machine learning (ML) are at the forefront of technological innovation. Python, with its extensive libraries and ease of use, has become the go-to programming language for data scientists, statisticians, and machine learning enthusiasts.
This guide provides a hands-on approach to mastering data science and Python machine learning, with in-depth discussions on statistics, predictive models, and machine learning techniques.
Statistics and Probability with Python
A strong foundation in statistics and probability is essential for working in data science and machine learning. These mathematical concepts enable you to understand data behavior, make informed decisions, and build predictive models. Python offers robust tools for statistical analysis and probability calculations, making it a preferred choice for data scientists.
Types of Data and Their Treatment
Data can be broadly categorized into:
- Nominal Data: Categorical data without a specific order (e.g., colors, names). This type of data is qualitative and is often represented using labels or codes.
- Ordinal Data: Categorical data with a meaningful order (e.g., ratings: good, better, best). Though it has a ranking, the intervals between values are not standardized.
- Interval Data: Numerical data without a true zero (e.g., temperature in Celsius). It allows for meaningful comparison of differences but not ratios.
- Ratio Data: Numerical data with a true zero (e.g., height, weight). This type supports operations like addition, subtraction, and ratio comparison.
Python libraries like Pandas make it easy to handle these data types by providing versatile structures like DataFrames. For example:
import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35], 'Score': [88, 92, 95]}
df = pd.DataFrame(data)
print(df.info())
Key Statistical Concepts
Statistics help summarize and understand data by providing key metrics. These include:
- Mean: The average value of a dataset, calculated by summing all data points and dividing by the number of points. It provides a central tendency but can be influenced by outliers.
- Median: The middle value of a sorted dataset, particularly useful when data is skewed, as it remains unaffected by extreme values.
- Mode: The most frequent value in the dataset, beneficial for analyzing categorical data to identify common categories.
- Standard Deviation: A measure of data spread, indicating how data points deviate from the mean. Smaller values signify less variability.
- Variance: The square of the standard deviation, illustrating the dispersion of data points around the mean, with higher values representing greater spread.
Python provides easy-to-use functions to compute these metrics using libraries like NumPy:
import numpy as np
data = [10, 20, 20, 40, 50]
print("Mean:", np.mean(data))
print("Median:", np.median(data))
print("Mode:", max(set(data), key=data.count))
print("Standard Deviation:", np.std(data))
print("Variance:", np.var(data))
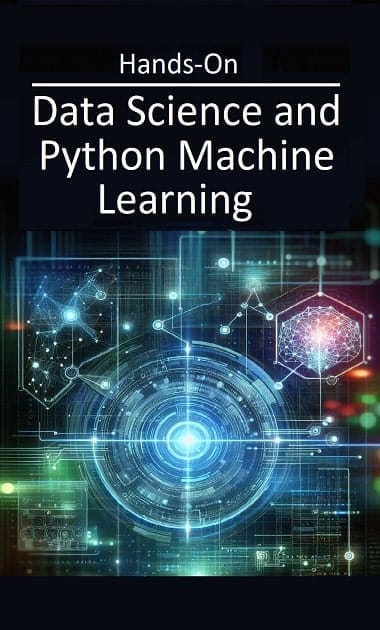
Probability Functions and Data Distributions
Probability plays a central role in predictive modeling, helping estimate the likelihood of events. Some key concepts include:
- Probability Density Functions (PDFs): Represent the likelihood of a continuous random variable taking on a specific value.
- Probability Mass Functions (PMFs): Represent the probabilities for discrete random variables.
Python libraries like Matplotlib and SciPy allow for easy visualization and analysis of data distributions, enabling insights into data behavior. Here’s how you can plot a normal distribution:
import matplotlib.pyplot as plt
import numpy as np
data = np.random.normal(0, 1, 1000)
plt.hist(data, bins=30, density=True, alpha=0.6, color='g')
plt.title('Normal Distribution')
plt.show()
By mastering these statistical and probability concepts, you gain the tools needed to process and analyze data effectively, laying the groundwork for building sophisticated machine learning models.
Exploring Machine Learning Techniques
Machine learning involves building algorithms that learn from and make predictions on data. It leverages mathematical and statistical techniques to enable systems to improve performance with experience, making it an essential tool for modern problem-solving.
Supervised and Unsupervised Learning
- Supervised Learning: Models are trained using labeled data, where the input-output relationships are explicitly known. Examples include classification tasks (e.g., spam detection) and regression tasks (e.g., predicting housing prices).
- Unsupervised Learning: Models discover patterns in unlabeled data. Common applications include clustering (e.g., customer segmentation) and dimensionality reduction (e.g., simplifying data for visualization).
Avoiding Overfitting
Overfitting occurs when a model performs well on training data but poorly on unseen data. Splitting the dataset into training and testing sets ensures better generalization of the model:
from sklearn.model_selection import train_test_split
X = np.array([[1], [2], [3], [4], [5]])
y = np.array([2, 4, 5, 4, 5])
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
Bayesian Methods
Bayesian inference involves updating the probability of a hypothesis as evidence accumulates. It provides a probabilistic approach to modeling uncertainty. Libraries like PyMC3 and SciPy make Bayesian analysis accessible for applications such as predicting trends or improving decision-making.
K-Means Clustering
K-means clustering groups data points into clusters based on similarity, measured by the distance between points. It’s widely used in image compression, customer segmentation, and anomaly detection.
from sklearn.cluster import KMeans
import numpy as np
data = np.array([[1, 2], [1, 4], [1, 0], [10, 2], [10, 4], [10, 0]])
kmeans = KMeans(n_clusters=2).fit(data)
print("Cluster Centers:", kmeans.cluster_centers_)
Decision Trees
Decision trees are interpretable models that split data based on feature conditions to make predictions. They are used for classification (e.g., identifying diseases) and regression (e.g., predicting stock prices).
from sklearn.tree import DecisionTreeClassifier
X = [[0, 0], [1, 1]]
y = [0, 1]
clf = DecisionTreeClassifier().fit(X, y)
Ensemble Learning
Ensemble methods combine predictions from multiple models to improve accuracy and robustness. Techniques like Random Forest and Gradient Boosting aggregate the strengths of individual models, reducing the risk of overfitting.
Support Vector Machines (SVM)
SVMs are effective for both linear and non-linear classification problems. By maximizing the margin between classes, they ensure robust predictions, making them ideal for tasks like handwriting recognition and bioinformatics.
from sklearn import svm
X = [[0, 0], [1, 1]]
y = [0, 1]
clf = svm.SVC().fit(X, y)
print(clf.predict([[2, 2]]))
Building Predictive Models with Python
Predictive modeling is at the heart of machine learning. It uses statistical and mathematical techniques to forecast outcomes based on historical data.
Linear Regression Using Python
Linear regression models the relationship between a dependent variable and one or more independent variables. It assumes a linear relationship and is widely used for its simplicity and interpretability. Scikit-learn simplifies implementation:
from sklearn.linear_model import LinearRegression
import numpy as np
X = np.array([1, 2, 3, 4, 5]).reshape(-1, 1)
y = np.array([2, 4, 5, 4, 5])
model = LinearRegression().fit(X, y)
print("Coefficient:", model.coef_)
print("Intercept:", model.intercept_)
Polynomial Regression in Python
Polynomial regression captures non-linear relationships by adding polynomial terms, making it a versatile tool for modeling curved data trends. It is especially useful in scenarios where linear regression fails to capture complexities. For example:
from sklearn.preprocessing import PolynomialFeatures
from sklearn.linear_model import LinearRegression
X = np.array([1, 2, 3, 4, 5]).reshape(-1, 1)
y = np.array([1, 4, 9, 16, 25])
poly = PolynomialFeatures(degree=2)
X_poly = poly.fit_transform(X)
model = LinearRegression().fit(X_poly, y)
Multivariate Regression Using Python
Multivariate regression involves multiple independent variables to predict a single dependent variable, making it effective for multidimensional problems. It is commonly applied in scenarios requiring a holistic understanding of how different factors influence outcomes. Here’s an example:
import numpy as np
from sklearn.linear_model import LinearRegression
X = np.array([[1, 2], [2, 3], [3, 4]])
y = np.array([2, 3, 4])
model = LinearRegression().fit(X, y)
print("Coefficients:", model.coef_)
Conclusion
Mastering data science and Python machine learning is a journey that requires a blend of theoretical knowledge and hands-on practice. By exploring statistics, predictive modeling, and advanced machine learning concepts, you can build robust analytical solutions for real-world challenges.
Download PDF: Hands-On Data Science and Python Machine Learning
Leave a Reply