Data Science is transforming industries worldwide, enabling organizations to analyze vast datasets and extract actionable insights. Python, a powerful and versatile programming language, is one of the most popular tools for data scientists due to its simplicity and robust ecosystem of libraries.
This article provides a comprehensive overview of Python for data science, covering topics such as Python’s object-oriented programming (OOP) concepts, modules, exceptions, arrays, and libraries.
Introduction to Data Science
Data Science is an interdisciplinary field that combines statistics, computer science, and domain expertise to analyze and interpret complex data. It involves collecting, cleaning, and analyzing data to uncover patterns and make data-driven decisions. With the rise of big data, businesses rely heavily on data science to enhance efficiency, optimize operations, and create predictive models.
Key Concepts of Data Science Using Python
1. Data Collection and Preprocessing
The first step in any data science project is collecting and preparing the data. Data can come from various sources, such as databases, APIs, or web scraping. Once collected, it often needs cleaning and preprocessing to handle missing values, duplicates, and inconsistencies.
Python Tools for Data Collection:
- BeautifulSoup: A library for web scraping to extract data from HTML and XML files.
- Scrapy: A robust framework for web scraping and crawling.
- Requests: Simplifies HTTP requests to interact with APIs.
Data Preprocessing with Python:
- Pandas: Used for data manipulation and cleaning. For instance:
import pandas as pd
# Load data
data = pd.read_csv('data.csv')
# Handle missing values
data.fillna(method='ffill', inplace=True)
# Remove duplicates
data.drop_duplicates(inplace=True)
2. Exploratory Data Analysis (EDA)
EDA involves summarizing the data to uncover patterns and insights. This step often uses visualizations to make data more understandable.
Popular Python Libraries for EDA:
- Matplotlib: For creating static, animated, and interactive plots.
- Seaborn: A library built on Matplotlib, offering more aesthetically pleasing visualizations.
- Pandas Profiling: Generates a complete report of the dataset, including statistics and visualizations.
Example of EDA using Python:
import pandas as pd
import seaborn as sns
import matplotlib.pyplot as plt
# Load dataset
data = pd.read_csv('data.csv')
# Summary statistics
print(data.describe())
# Visualization
sns.pairplot(data)
plt.show()
3. Feature Engineering
Feature engineering involves selecting and transforming variables to improve model performance. Key techniques include:
- Scaling and Normalization: Ensures all features contribute equally to the analysis.
- Encoding Categorical Variables: Converts categorical data into numerical form using one-hot encoding or label encoding.
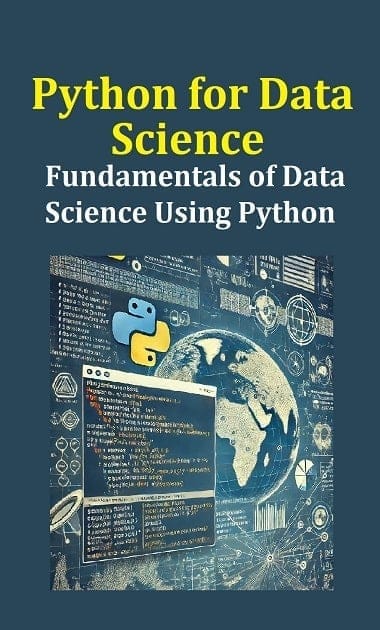
Python Example for Feature Engineering:
from sklearn.preprocessing import StandardScaler, OneHotEncoder
import pandas as pd
# Scaling numerical data
scaler = StandardScaler()
scaled_data = scaler.fit_transform(data[['feature1', 'feature2']])
# Encoding categorical data
encoder = OneHotEncoder()
encoded_data = encoder.fit_transform(data[['category']]).toarray()
4. Statistical Analysis Using Python
Statistical analysis helps validate hypotheses and derive meaningful conclusions from data. Python libraries like SciPy and Statsmodels are widely used for statistical testing.
Example of Statistical Testing:
from scipy.stats import ttest_ind
# Perform a t-test
t_stat, p_value = ttest_ind(data['group1'], data['group2'])
print(f"T-statistic: {t_stat}, P-value: {p_value}")
5. Machine Learning Using Python
Machine learning is a core component of data science. Python’s scikit-learn library is a powerful tool for implementing machine learning algorithms. It supports tasks such as classification, regression, clustering, and more.
Example of Machine Learning with Python:
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a Random Forest model
model = RandomForestClassifier()
model.fit(X_train, y_train)
# Evaluate the model
predictions = model.predict(X_test)
print(f"Accuracy: {accuracy_score(y_test, predictions)}")
6. Data Visualization in Python
Data visualization plays a vital role in communicating insights effectively. Libraries like Plotly and Bokeh offer interactive visualization tools, while Matplotlib and Seaborn are perfect for static plots.
Example of Data Visualization:
import matplotlib.pyplot as plt
import seaborn as sns
# Line plot
plt.plot(data['Date'], data['Sales'])
plt.title('Sales Over Time')
plt.xlabel('Date')
plt.ylabel('Sales')
plt.show()
# Heatmap
sns.heatmap(data.corr(), annot=True)
plt.show()
7. Big Data Processing Using Python
For handling massive datasets, Python integrates with big data technologies like Apache Spark via PySpark.
Example of Big Data Analysis with PySpark:
from pyspark.sql import SparkSession
# Initialize Spark session
spark = SparkSession.builder.appName("Big Data Analysis").getOrCreate()
# Load large dataset
data = spark.read.csv('bigdata.csv', header=True, inferSchema=True)
data.show()
Python OOP Concepts
Object-Oriented Programming (OOP) is a programming paradigm in Python that organizes code into reusable “objects.” Understanding OOP concepts is essential for creating scalable and maintainable data science applications.
Key OOP Concepts in Python:
1. Classes and Objects in Python:
- A class is a blueprint for objects, and an object is an instance of a class.
- Example:
class Data:
def __init__(self, dataset):
self.dataset = dataset
def display(self):
print(self.dataset)
data = Data("Sample Dataset")
data.display()
2. Inheritance:
- Enables a class to derive properties and behaviors from another class.
- Example:
class Analysis(Data):
def analyze(self):
print(f"Analyzing: {self.dataset}")
analysis = Analysis("Sales Data")
analysis.analyze()
3. Polymorphism in Python:
- Allows methods to be redefined in derived classes.
- Example:
class Data:
def process(self):
print("Processing Data")
class TextData(Data):
def process(self):
print("Processing Text Data")
data = TextData()
data.process()
4. Encapsulation in Python:
- Bundles data and methods within a class to restrict access to specific parts of an object.
OOP concepts are integral for building complex data science pipelines and reusable modules.
Python Modules, Exceptions, and Arrays
Python’s modular structure and error-handling mechanisms are essential for building robust data science applications.
Python Modules
Modules in Python are files containing reusable code. They simplify development by allowing you to organize your code and avoid redundancy. Python offers both standard modules (e.g., math, os, random) and third-party libraries for specialized tasks.
Example: Using Modules for Random Sampling in Python
import random
# Generate a random sample
data_sample = random.sample(range(1, 100), 10)
print(data_sample)
Handling Exceptions in Python
Exception handling is crucial for managing errors that arise during data processing. Python’s try-except blocks ensure that your program can recover gracefully from errors like missing files or incompatible data types.
Example: Exception Handling in Data Loading
try:
data = pd.read_csv('nonexistent_file.csv')
except FileNotFoundError as e:
print(f"Error: {e}")
Working with Arrays in Python
Arrays, provided by the array module or NumPy, are critical for numerical computations in data science. They allow for efficient storage and manipulation of data.
Example: Using NumPy Arrays
import numpy as np
# Create a NumPy array
array = np.array([1, 2, 3, 4, 5])
# Perform element-wise operations
print(array * 2)
Conclusion
Python has established itself as the premier language for data science, thanks to its versatility and powerful libraries. By understanding the core concepts outlined in this guide—data collection, preprocessing, EDA, machine learning, and visualization—you can confidently embark on your data science journey. As you progress, remember to continuously learn and adapt, as the field of data science evolves rapidly.
Download PDF: Python For Data Science – Fundamentals of Data Science Using Python