Python has established itself as a powerful language for statistical analysis and data visualization. Its user-friendly syntax, coupled with a wide array of libraries, makes Python an essential tool for anyone working with data. In this article, we’ll explore how Python can be used to perform statistical analysis and create compelling visualizations.
We’ll cover foundational concepts, dive into descriptive statistics and data visualization with Python, understand random variables and probability, and discuss hypothesis testing.
Analysis Basics of Python for Statistical Analysis
Before diving into statistical analysis, it’s essential to understand Python’s basic structure and the libraries that facilitate these operations. Python offers several robust modules to handle data efficiently:
Core statistical libraries in Python
1. NumPy
NumPy is a fundamental library for numerical computations. It provides support for arrays, mathematical functions, and random number generation.
import numpy as np
# Create a NumPy array
data = np.array([1, 2, 3, 4, 5])
# Compute basic statistics
mean = np.mean(data)
std_dev = np.std(data)
2. SciPy
SciPy builds upon NumPy and includes modules for optimization, integration, and advanced statistical operations.
from scipy.stats import skew, kurtosis
# Calculate skewness and kurtosis
skewness = skew(data)
kurt = kurtosis(data)
3. pandas
pandas is indispensable for data manipulation and analysis. It allows users to load, clean, and transform datasets seamlessly.
import pandas as pd
# Load a dataset
df = pd.read_csv('dataset.csv')
# View basic statistics
summary = df.describe()
Understanding Descriptive Statistics
Descriptive statistics summarize the main characteristics of a dataset, providing a foundation for further analysis by focusing on its central tendency, variability, and distribution. These metrics allow us to make sense of raw data by distilling it into meaningful summaries. Below are the key components of descriptive statistics:
1. Measures of Central Tendency
Central tendency refers to the “center” of a dataset.
- Mean: The average of the values, calculated by summing all data points and dividing by their count.
- Median: The middle value when the data is sorted, robust against outliers.
- Mode: The most frequently occurring value, ideal for categorical data.
mean = df['column_name'].mean()
median = df['column_name'].median()
mode = df['column_name'].mode()[0]
2. Measures of Dispersion
Dispersion indicates how spread out the data points are.
- Range: The difference between the maximum and minimum values.
- Variance: Quantifies the average squared deviation from the mean.
- Standard Deviation: The square root of variance, giving a more interpretable measure of spread.
range_value = df['column_name'].max() - df['column_name'].min()
variance = df['column_name'].var()
std_dev = df['column_name'].std()
3. Shape of Distribution
These metrics describe the overall form of the dataset.
- Skewness: Indicates asymmetry; positive skew means a longer tail on the right.
- Kurtosis: Measures the “tailedness” of the distribution, highlighting the presence of outliers.
Understanding these descriptive measures enables analysts to grasp the nature of a dataset, identify trends, detect anomalies, and lay the groundwork for more complex statistical analyses.
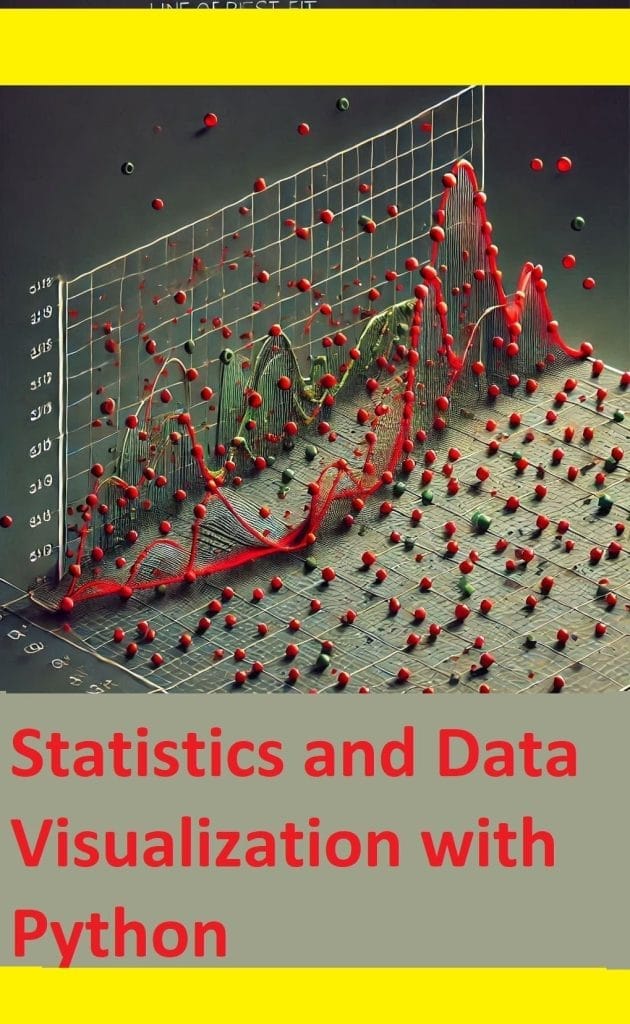
Download PDF: Statistics and Data Visualization with Python
Random Variables and Probability
1. Random Variables
A random variable is a numerical representation of outcomes from a random process. It forms the backbone of probability and statistics by assigning values to random phenomena.
- Discrete Random Variables: These take on specific, countable values. Examples include the number of heads in a series of coin tosses or the number of defective items in a batch. Their probabilities are described using functions like probability mass functions (PMFs).
- Continuous Random Variables: These can take any value within a specified range, such as the weight of individuals or the temperature in a city. Continuous variables use probability density functions (PDFs) to describe probabilities.
2. Probability Distributions
Probability distributions define how the probabilities of different outcomes are spread over the values of the random variable.
- Normal Distribution: Often called the Gaussian distribution, it is symmetric and bell-shaped, frequently used in natural and social sciences.
- Binomial Distribution: Describes the probability of a fixed number of successes in a fixed number of trials, given a constant probability of success.
- Poisson Distribution: Models the probability of a given number of events occurring in a fixed interval, useful for rare events.
from scipy.stats import norm, binom, poisson
# Normal distribution
normal_pdf = norm.pdf(x=0, loc=0, scale=1)
# Binomial distribution
binom_prob = binom.pmf(k=2, n=5, p=0.5)
# Poisson distribution
poisson_prob = poisson.pmf(k=3, mu=2)
Understanding these distributions allows us to perform advanced statistical analyses, such as hypothesis testing and predictive modeling.
Hypothesis Testing and Statistical Tests
Hypothesis testing is a statistical method used to make inferences or decisions about a population based on sample data. It involves formulating a null hypothesis (H₀) and an alternative hypothesis (H₁) and then using statistical tests to determine whether the observed data provides sufficient evidence to reject the null hypothesis. This process is critical in fields like science, business, and healthcare for validating assumptions and making data-driven decisions.
1. Parametric Tests
Parametric tests rely on the assumption that the data follows a specific distribution, typically a normal distribution. These tests are powerful and widely used when data meets their assumptions. Common parametric tests include:
- t-test: Used to compare the means of two groups.
- ANOVA (Analysis of Variance): Used to compare the means of three or more groups.
from scipy.stats import ttest_ind, f_oneway
# t-test
t_stat, p_value = ttest_ind(group1, group2)
# ANOVA
anova_result = f_oneway(group1, group2, group3)
2. Non-Parametric Tests
When data does not meet the assumptions required for parametric tests (e.g., non-normal distribution or unequal variances), non-parametric tests are preferred. These include:
- Mann-Whitney U Test: Compares the medians of two independent groups.
- Kruskal-Wallis Test: Extends Mann-Whitney to compare medians across multiple groups.
from scipy.stats import mannwhitneyu, kruskal
# Mann-Whitney U Test
u_stat, p_value = mannwhitneyu(group1, group2)
# Kruskal-Wallis Test
kw_stat, p_value = kruskal(group1, group2, group3)
Both approaches provide robust tools for determining whether observed differences are statistically significant, allowing analysts to draw meaningful conclusions from data.
Data Visualization with Python
Data visualization plays a pivotal role in presenting statistical findings effectively.
1. Basic Visualizations
Using libraries like Matplotlib and Seaborn, you can create:
- Histograms: Show data distribution.
- Boxplots: Display variability and outliers.
import matplotlib.pyplot as plt
import seaborn as sns
# Histogram
plt.hist(df['column_name'], bins=10, color='blue', edgecolor='black')
plt.title('Histogram')
plt.show()
# Boxplot
sns.boxplot(x='category', y='value', data=df)
plt.title('Boxplot')
plt.show()
2. Advanced Visualizations
For more interactive and detailed plots, libraries like Plotly and Bokeh can be used.
import plotly.express as px
# Interactive scatter plot
fig = px.scatter(df, x='x_column', y='y_column', color='group_column')
fig.show()
3. Time Series Visualization
Time series data can be visualized to uncover trends over time.
plt.plot(df['date'], df['value'])
plt.title('Time Series Plot')
plt.xlabel('Date')
plt.ylabel('Value')
plt.show()
Conclusion
Python’s extensive library ecosystem makes it an indispensable tool for statistical analysis and data visualization. Starting with the basics using NumPy, SciPy, and pandas, you can quickly progress to advanced techniques like hypothesis testing and creating sophisticated visualizations. By mastering descriptive statistics, probability distributions, and both parametric and non-parametric tests, you can extract meaningful insights from data. Leveraging visualization tools like Matplotlib, Seaborn, and Plotly, you can communicate your findings effectively to any audience.
Leave a Reply