Biostatistics with Python plays a critical role in modern biological research, clinical trials, and biotechnology by providing a framework for interpreting data. Python, with its versatile libraries and tools, is becoming the preferred programming language for performing biostatistical analysis, hypothesis testing, and predictive modeling.
This article introduces key Python libraries, techniques for hypothesis testing, effect size interpretation, and predictive biostatistics, with a focus on real-world applications in life sciences.
Introduction to Python for Biostatistics
Python is a powerful tool for biostatistics due to its extensive collection of libraries for statistical analysis, hypothesis testing, and predictive modeling. Let’s explore these key components:
Libraries for Biostatistics Hypothesis Tests in Python
Python provides a range of robust libraries specifically designed for biostatistical hypothesis testing:
- SciPy: This core scientific library includes modules such as scipy.stats that support t-tests, chi-squared tests, ANOVA, and non-parametric tests. It is a fundamental tool for conducting hypothesis testing in Python.
- Statsmodels: Statsmodels provides advanced tools for hypothesis testing and regression analysis, such as linear models, ANOVA, and time-series analysis. It also produces detailed statistical summaries for in-depth interpretation.
- Pingouin: A relatively new library, Pingouin simplifies complex hypothesis testing with user-friendly syntax while supporting advanced tests like effect sizes, pairwise comparisons, and more.
- NumPy and Pandas: NumPy provides efficient numerical computations, while Pandas is used for data preprocessing and manipulation, ensuring data is clean and ready for analysis.
The Underlying Principles of P-values
P-values are at the heart of hypothesis testing. They quantify the evidence against the null hypothesis by calculating the probability of obtaining results as extreme as those observed, assuming the null hypothesis is true. In biostatistics, a p-value threshold of 0.05 is often used, where a smaller value (p < 0.05) suggests strong evidence to reject the null hypothesis in favor of the alternative hypothesis.
Performing Tests in Python
Python’s scipy.stats module simplifies hypothesis testing for various statistical needs. For instance:
from scipy import stats
# Example: Performing a t-test
sample1 = [12, 15, 14, 10, 13]
sample2 = [22, 25, 20, 18, 19]
t_stat, p_value = stats.ttest_ind(sample1, sample2)
print(f"T-statistic: {t_stat}, P-value: {p_value}")
Libraries for Predictive Biostatistics in Python
Predictive biostatistics relies on machine learning and statistical models to forecast outcomes. Python supports this through:
- Scikit-learn: A powerful machine learning library that offers tools for linear regression, logistic regression, and classification models.
- Statsmodels: Ideal for statistical modeling, hypothesis testing, and generating interpretable regression outputs.
- Seaborn and Matplotlib: These libraries create detailed visualizations of predictive models, such as regression plots, ROC curves, and feature distributions, enhancing model interpretation.
With Python’s tools, researchers can efficiently conduct hypothesis tests and implement predictive biostatistical models, driving meaningful insights in biomedical and biotechnology fields.
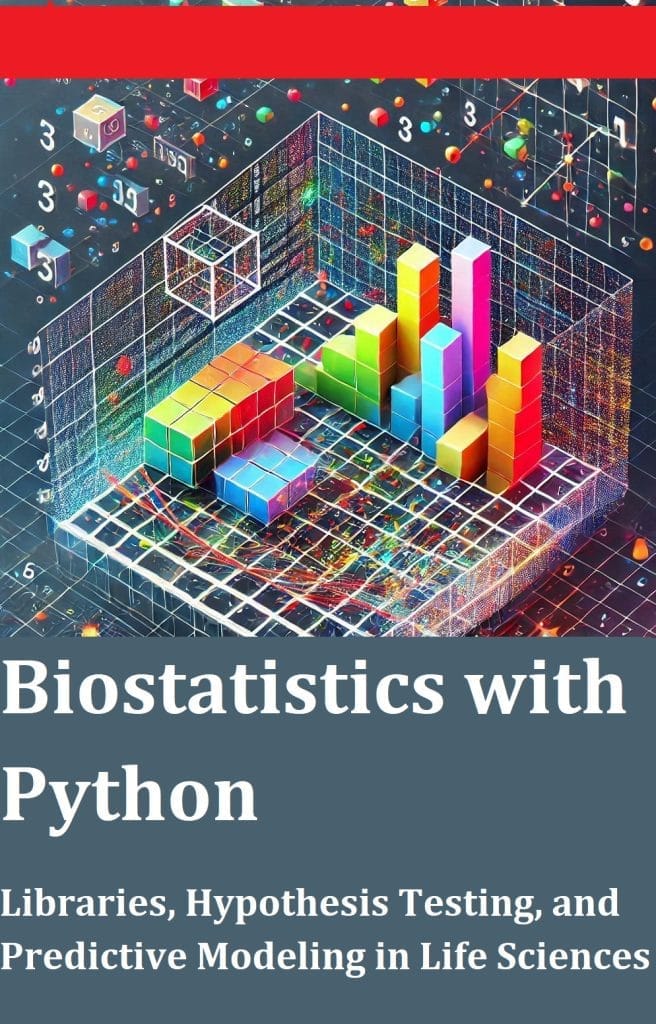
Download PDF: Biostatics with Python
Biostatistical Inference Using Hypothesis Tests and Effect Sizes
Performing Student’s t-test in Python and Interpreting Effect Sizes
A Student’s t-test compares the means of two samples to determine if they are statistically different. In addition to p-values, effect size (Cohen’s d) is used to quantify the magnitude of the difference.
Example: Two-Sample t-test with Effect Size
import numpy as np
from scipy import stats
# Data
sample1 = np.array([12, 14, 15, 11, 13])
sample2 = np.array([22, 24, 21, 23, 25])
# t-test
t_stat, p_val = stats.ttest_ind(sample1, sample2)
# Effect size (Cohen's d)
mean_diff = np.mean(sample2) - np.mean(sample1)
pooled_std = np.sqrt((np.std(sample1)**2 + np.std(sample2)**2) / 2)
effect_size = mean_diff / pooled_std
print(f"T-statistic: {t_stat}, P-value: {p_val}, Effect Size (Cohen's d): {effect_size}")
In this example:
- The t-statistic indicates how far apart the group means are relative to the pooled standard deviation.
- The p-value evaluates whether the observed difference is statistically significant. If p<0.05p < 0.05p<0.05, we reject the null hypothesis and conclude that the means are significantly different.
How Does the t-test Work?
The t-test works by calculating the ratio of the difference between the group means to the variability (standard error). It assumes the data is sampled independently from normally distributed populations. For small sample sizes, the t-test is highly sensitive to violations of normality, making tests like the Wilcoxon signed-rank test a robust alternative when this assumption is not met.
The result of the t-test is a t-statistic and a p-value, both of which help determine if the observed difference is due to chance or represents a true effect. To strengthen the analysis, effect size (Cohen’s d) bridges the gap between statistical significance and practical significance, offering a more complete interpretation of results.
By combining p-values with effect size, researchers gain deeper insights into their biostatistical findings, ensuring both statistical rigor and meaningful conclusions.
Performing Wilcoxon Signed-Rank Test in Python
The Wilcoxon signed-rank test is a non-parametric test used when the assumptions of the paired t-test, such as normality, are not met. It evaluates whether the median difference between paired observations is zero, making it ideal for analyzing matched-pair samples or before-and-after studies. Unlike the paired t-test, it works with ordinal data or non-normally distributed continuous data, ranking the absolute differences between paired values while considering their signs (+/-).
Example: Analyzing Improvement After Treatment
from scipy.stats import wilcoxon
# Example Data
before = [10, 12, 14, 11, 13] # Before treatment
after = [15, 17, 18, 14, 16] # After treatment
# Wilcoxon Signed-Rank Test
stat, p = wilcoxon(before, after)
print(f"Wilcoxon statistic: {stat}, P-value: {p}")
The test returns a Wilcoxon statistic and p-value. A p-value below 0.05 indicates a significant difference between the paired samples, supporting the alternative hypothesis.
Performing Chi-Squared Tests in Python
The chi-squared test evaluates the association between categorical variables by comparing observed and expected frequencies in a contingency table. It determines whether the differences between groups are statistically significant or due to chance. This is particularly useful in biostatistics for analyzing survey results, clinical studies, or genetic data. The output includes the chi-squared statistic, p-value, degrees of freedom (dof), and expected frequencies, which help assess the relationship between variables.
import pandas as pd
from scipy.stats import chi2_contingency
# Contingency Table
data = [[50, 30], [20, 40]]
chi2, p, dof, ex = chi2_contingency(data)
print(f"Chi-squared: {chi2}, P-value: {p}")
Analyzing Associations Among Multiple Variables – Correlations in Python
Correlation analysis helps identify the strength and direction of associations between variables. Pearson’s r measures linear relationships, while Spearman’s rho assesses monotonic relationships, making it suitable for non-linear or ranked data. Python’s Pandas calculates correlation matrices, and libraries like Seaborn visualize these associations using heatmaps, which highlight variable relationships intuitively. Correlation analysis is crucial for identifying predictors in biostatistics and forming hypotheses for further testing.
import seaborn as sns
import matplotlib.pyplot as plt
import pandas as pd
# Correlation Heatmap
df = pd.DataFrame({"A": [1, 2, 3], "B": [2, 4, 6], "C": [3, 6, 9]})
sns.heatmap(df.corr(), annot=True, cmap="coolwarm")
plt.show()
Analyzing Multiple Groups in Python – ANOVA and Kruskal–Wallis Test
When analyzing data with more than two groups, statistical tests such as ANOVA (Analysis of Variance) and the Kruskal–Wallis test become essential. These tests help determine whether significant differences exist between group means, allowing researchers to make data-driven decisions.
ANOVA (Analysis of Variance)
ANOVA is a parametric test used to compare the means of three or more groups. It assumes that the data within each group follows a normal distribution and that the group variances are approximately equal (homoscedasticity). The test calculates the F-statistic, which compares the variance between the group means to the variance within the groups. If the p-value associated with the F-statistic is less than the significance level (e.g., 0.05), the null hypothesis—stating that all group means are equal—is rejected.
For example, in biomedical research, ANOVA can determine if blood pressure varies significantly across patients receiving three different treatments. Post-hoc tests like Tukey’s HSD can identify which specific groups differ.
Kruskal–Wallis Test
The Kruskal–Wallis test is the non-parametric alternative to ANOVA. It is used when the assumptions of ANOVA (normality and equal variances) are violated. Instead of comparing means, it ranks the data and tests for significant differences in the median values across groups. This test is particularly useful for skewed or ordinal data.
For example, if patient satisfaction scores are not normally distributed, the Kruskal–Wallis test can determine if there are differences among hospitals. Both tests are critical in biostatistics for analyzing multiple groups and drawing robust conclusions from biological or clinical data.
Predictive Biostatistics Using Python
Learning Predictive Biostatistics and Their Uses
Predictive biostatistics applies regression models to predict outcomes based on independent variables. Examples include predicting disease risk, drug response, or health outcomes.
Dependent and Independent Variables
- Dependent Variable: In predictive modeling, dependent variables are the outcomes researchers seek to predict or understand. These could include health metrics such as cholesterol levels, disease presence, or patient survival rates
- Independent Variable: On the other hand, independent variables are the factors believed to influence the dependent variable. For example, in a study predicting heart disease risk, independent variables could include age, blood pressure, cholesterol levels, and smoking habits. These predictors help build models that assess how changes in these variables influence health outcomes.
Linear Regression for Biostatistics in Python
Linear regression models the relationship between a dependent variable and one or more independent variables. The statsmodels library makes it easy to perform linear regression, assess model fit, and interpret the coefficients, helping researchers make accurate predictions from complex datasets.
import statsmodels.api as sm
# Data
x = [1, 2, 3, 4, 5]
y = [2.2, 2.8, 4.5, 4.0, 5.5]
x = sm.add_constant(x)
# Linear Regression
model = sm.OLS(y, x).fit()
print(model.summary())
Logistic Regression in Python
Logistic regression, unlike linear regression, is used when the dependent variable is binary. For instance, predicting whether a patient will develop a particular disease (yes/no) based on various health indicators can be done using logistic regression.
Python’s sklearn library offers a simple interface for performing logistic regression and interpreting the odds ratios, which help understand the probability of certain outcomes based on predictor variables.
from sklearn.linear_model import LogisticRegression
# Example Data
X = [[1], [2], [3], [4], [5]]
y = [0, 0, 1, 1, 1]
# Logistic Regression
model = LogisticRegression().fit(X, y)
print(model.predict_proba([[3]]))
Multiple Linear and Logistic Regressions Using Python
Multiple regression models include multiple independent variables to predict a dependent variable. This approach enhances predictive power, especially for complex datasets where a single predictor is not sufficient to explain the outcome.
Multiple linear regression is used when the outcome is continuous, while multiple logistic regression is suitable for binary outcomes. These models can help researchers understand how combinations of predictors influence outcomes, providing a more nuanced view of the factors affecting health.
T-Test, ANOVA, and Linear and Logistic Regression
Implementing Different Versions of Student’s t-test
Python can handle different versions of the Student’s t-test to compare means between groups:
- Independent t-test: This test compares the means of two independent groups to determine if there is a statistically significant difference between them. It’s commonly used when analyzing data from two separate, unrelated samples.
- Paired t-test: Used when comparing two related samples or matched data points, such as before-and-after treatment measurements from the same subjects. This test helps assess whether the mean difference between the pairs is zero.
Applying Post-Hoc Tests Using ANOVA
After conducting an ANOVA, if significant differences are found among groups, post-hoc tests like Tukey’s HSD can be used to identify exactly which groups differ. These tests perform pairwise comparisons to pinpoint the specific group differences that contribute to the overall significant result.
Performing and Visualizing Linear Regression in Python
To visualize linear regression results, Python’s Seaborn library can create scatter plots with a regression line. This helps to visually assess the relationship between the independent and dependent variables.
import seaborn as sns
sns.lmplot(x="A", y="B", data=df)
plt.show()
Conclusion
Python simplifies biostatistics by offering powerful libraries and tools for hypothesis testing, predictive modeling, and data analysis. By mastering techniques like t-tests, ANOVA, linear and logistic regression, and their interpretations, researchers can solve complex problems in biomedical and life sciences. Biostatistics with Python enables data-driven discoveries, enhancing healthcare, biotechnology, and research outcomes.
Leave a Reply