Python is the most widely used programming language in the world. It is also known for its simplicity, readability, and extensive ecosystem. A well-organized Python cheat sheet can serve as a quick reference guide for a beginner or an experienced developer. This guide provides an in-depth overview of Python’s core features. It helps you to understand how to use the language effectively in different scenarios.
1. Getting Started with Python
1.1 Installing Python
To begin coding in Python, you need to downloaded it from its official website and install it on your system. Ensure that you get the latest stable version. You can check the version number in your terminal or command prompt to verify.
1.2 Running Python Code
There are multiple ways to execute Python Code:
- Interactive Mode: This mode allows you to run Python commands one at a time in the terminal. It is useful for testing small code snippets.
- Script Mode: Python scripts are saved with a .py extension and can be executed as standalone files.
- Jupyter Notebooks: These provide an interactive interface, particularly useful for data science and machine learning applications.
1.3 Understanding Indentation in Python
Python relies on indentation to define the blocks of code. This means the number of spaces at the beginning of a line is significant. To avoid syntax errors it is crucial to maintain consistent indentation.
1.4 Variables and Data Types
Python can automatically determine variable types based on the assigned value. This flexibility makes it easier to declare and use variables without explicitly mentioning data types. Some common data types include:
- Strings – Used for text data
- Integers – Used for whole numbers
- Floats – Used for decimal values
- Booleans – Used for logical operations
Python also provides functions to check and convert data types, ensuring smooth data handling in various applications.
2. Operators in Python
To perform calculations and logical operations in Python operators are essential. There are mainly three types of operators:
2.1 Arithmetic Operators
These types of operators are used to handle mathematical operations such as addition (+), subtraction (-), multiplication (*), and division (/). Rather than this, there are also some other operators like floor division (used to discard decimal values), modulus (used to find remainders), and exponentiation (for powers).
2.2 Comparison Operators
It is used to evaluate conditions and it returns Boolean values (True or False) which are used in further decision-making structures. For example, it can be used to compare values if they are equal, greater than, or less than to each other.
2.3 Logical Operators
Python has three logical operators, and, or, and not operators. These are particularly useful for combining multiple conditions to build complex decision-making expressions.
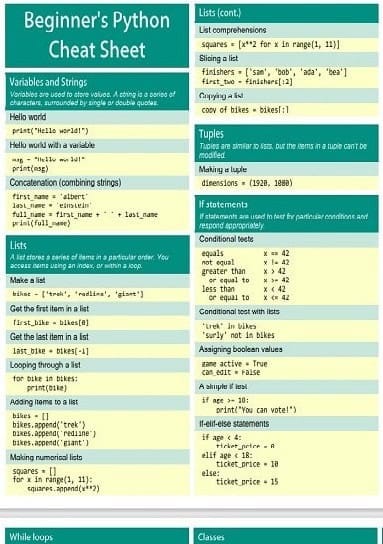
3. Python Data Structures
Python provides powerful data structures that enable efficient storage, retrieval, and manipulation of data. These built-in structures are extensively used across various programming applications to enhance code efficiency and organization.
3.1 Lists
Lists can store multiple items and considering as ordered, mutable collections. It is one of the most versatile elements in Python and supports various operations like adding, removing, and sorting elements.
3.2 Tuples
Tuples are the same as lists and are mostly used when data integrity needs to be preserved but the only difference is they are immutable, which means once the value is created cannot change.
3.3 Dictionaries
It stores data in key-value pairs, allowing quick lookups and efficient data retrieval. They are widely used for organizing structured data, mapping relationships, and enhancing data accessibility within Python programs.
3.4 Sets
Sets are unordered collections of unique elements and are useful when handling large datasets where duplicate values need to be removed or checked efficiently.
4. Control Flow in Python
Control flow determines how different parts of a program execute based on conditions and loops.
4.1 Conditional Statements
Python provides if, elif, and else statements to execute code based on specific conditions. These structures allow dynamic decision-making in applications.
4.2 Loops
Loops help in automating repetitive tasks:
- For loops iterate over sequences such as lists, tuples, or strings.
- While loops execute code as long as a specified condition remains true.
Using loops effectively helps streamline complex operations and improves code efficiency.
5. Functions in Python
Functions in Python enable code reuse and modular programming.
5.1 Defining and Calling Functions
Functions are created using the def keyword followed by the function name and parentheses. They can accept parameters and return values, making them useful for structuring programs into manageable components.
5.2 Lambda Functions
Python allows the creation of anonymous functions using the lambda keyword. These one-liner functions are commonly used in situations requiring quick computations without defining a full function.
6. File Handling in Python
To interact with files, Python has built-in functions.
6.1 Reading and Writing Files
Files can be opened in different modes:
- r for reading
- w for writing (overwrites existing content)
- a for appending new data
Using context managers (the with statement) ensures files are properly handled, reducing the risk of memory leaks.
6.2 Working with CSV Files
Python’s built-in CSV module simplifies reading and writing CSV files, making it ideal for data analysis applications.
7. Exception Handling in Python
Errors in Python can be managed using exception handling.
7.1 Handling Errors Gracefully
Python provides try, except, and finally blocks to catch and manage errors. This prevents abrupt program termination and allows smooth execution of alternative code.
7.2 Common Python Errors
Some frequently encountered errors include:
- SyntaxError due to incorrect syntax
- TypeError when operations are applied to incompatible data types
- ZeroDivisionError occurs when attempts to divide by zero.
It is important to understand these errors for effective coding practice.
8. Python Libraries and Modules
Python’s ecosystem is enriched by a vast collection of libraries that enhance its functionality.
8.1 Importing Modules
Modules contain pre-written functions that can be imported into a script. Python’s standard library includes modules for math, file handling, and more.
8.2 Popular Python Libraries
- NumPy: Efficient numerical computations
- Pandas: Data analysis and manipulation
- Matplotlib: Data visualization
- Scikit-learn: Machine learning algorithms
- TensorFlow/PyTorch: Deep learning frameworks
Using these libraries significantly reduces development time and improves code efficiency.
9. Object-Oriented Programming in Python
Python supports object-oriented programming (OOP), which allows the creation of reusable and modular code.
9.1 Classes and Objects
Classes define blueprints for objects, while objects are instances of these classes. Python’s OOP principles include encapsulation, inheritance, and polymorphism, enabling efficient software design.
9.2 Benefits of OOP in Python
- Improved code organization
- Reusability of components
- Easier maintenance and scalability
Conclusion
This Python cheat sheet serves as a valuable reference guide for developers at all levels. Understanding Python’s core concepts, data structures, and best practices ensures efficient programming and problem-solving. Whether you are a beginner or an experienced coder, keeping this guide handy will enhance your coding skills and workflow.