In the rapidly evolving landscape of technology, machine learning and deep learning, stand out as pivotal domains that drive innovation across various industries. From healthcare to finance, the ability to develop advanced models using frameworks like PyTorch and Scikit-Learn has become an indispensable skill for modern data scientists and developers.
This article will cover the core concept of mastering machine learning and Deep learning with PyTorch, offering insights and strategies to develop robust and scalable models.
Understanding Machine Learning and Deep Learning
Machine learning is a subset of artificial intelligence (AI) that focuses on developing algorithms that allow computers to learn from and make predictions based on data. Deep learning, a further subset of machine learning, involves neural networks with many layers (deep neural networks) that can model complex patterns in data.
PyTorch and Scikit-Learn are two of the most popular libraries in the Python ecosystem for implementing machine learning and deep learning models.
Why Choose PyTorch and Scikit-Learn?
PyTorch is renowned for its flexibility and dynamic computation graph, making it a favorite among researchers and practitioners for developing and experimenting with deep learning models. It provides a seamless path from research to production deployment, thanks to its integration with major platforms and ease of use.
Scikit-Learn, on the other hand, is a robust library for traditional machine learning. It offers a wide range of simple and efficient tools for data mining and data analysis, making it accessible for beginners while powerful enough for advanced users.
Getting Started with Scikit-Learn
Scikit-Learn is a versatile machine-learning library in Python that provides simple and efficient tools for data mining and data analysis. It is built on top of NumPy, SciPy, and Matplotlib and is known for its easy-to-use API.
1. Installing Scikit-Learn
To start using Scikit-Learn, you need to install it along with the necessary dependencies. You can do this using pip:
pip install scikit-learn
2. Key Features of Scikit-Learn
- Classification: Identify the category to which an object belongs. Example: Email spam detection.
- Regression: Predict a continuous value. Example: House price prediction.
- Clustering: Group objects into clusters. Example: Customer segmentation.
- Dimensionality Reduction: Reduce the number of random variables. Example: Principal Component Analysis (PCA).
- Model Selection: Compare, validate, and select parameters and models.
- Preprocessing: Feature extraction and normalization.
3. Building a Simple Scikit Learn Machine Learning Model
Let’s walk through a basic example of building a machine-learning model using Scikit-Learn.
Step 1: Importing Libraries
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.datasets import load_iris
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
Step 2: Loading the Dataset
iris = load_iris()
X = iris.data
y = iris.target
Step 3: Splitting the Dataset
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
Step 4: Training the Model
clf = RandomForestClassifier(n_estimators=100)
clf.fit(X_train, y_train)
Step 5: Making Predictions and Evaluating the Model
y_pred = clf.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print(f"Model Accuracy: {accuracy * 100:.2f}%")
This simple example demonstrates how easy it is to build a machine-learning model using Scikit-Learn. The library’s extensive documentation and active community make it a great starting point for anyone interested in machine learning.
Scikit-Learn simplifies the process of building and evaluating machine learning models. Its consistent API and comprehensive documentation make it an excellent choice for beginners and experts.
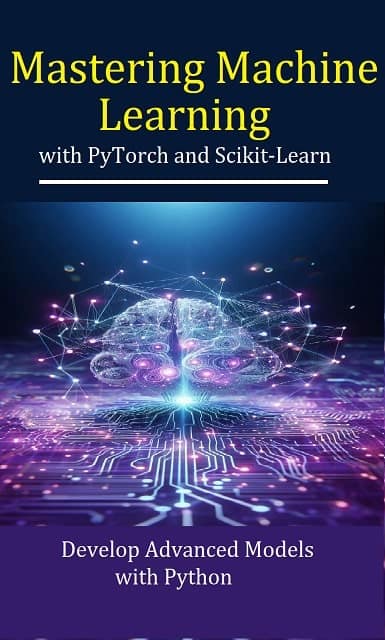
Example: Building a Regression Model
Here’s how to build a simple linear regression model using Scikit-Learn:
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
# Sample data
X = [[1], [2], [3], [4], [5]]
y = [1, 4, 9, 16, 25]
# Split the data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Instantiate and train the model
model = LinearRegression()
model.fit(X_train, y_train)
# Predict and evaluate
y_pred = model.predict(X_test)
print(f'Mean Squared Error: {mean_squared_error(y_test, y_pred)}')
Diving into Deep Learning with PyTorch
PyTorch is a deep learning library developed by Facebook’s AI Research lab. It has gained immense popularity due to its dynamic computational graph, ease of use, and flexibility. PyTorch is widely used in both academia and industry for developing deep learning models.
1. Installing PyTorch
To install PyTorch, use the following command:
pip install torch torchvision torchaudio
2. Key Features of PyTorch
- Dynamic Computational Graph: Unlike static computational graphs used by other deep learning frameworks, PyTorch allows you to modify the graph on the fly.
- Automatic Differentiation: PyTorch’s autograd module provides automatic differentiation, which is essential for training neural networks.
- Support for GPU: PyTorch seamlessly integrates with GPUs, enabling faster computation.
- Extensive Libraries: PyTorch has numerous libraries and tools like torchvision (for computer vision) and torchaudio (for audio processing).
3. Building a Simple Neural Network in PyTorch
Let’s build a basic neural network model using PyTorch.
Step 1: Importing Libraries
import torch
import torch.nn as nn
import torch.optim as optim
import torch.nn.functional as F
from torchvision import datasets, transforms
from torch.utils.data import DataLoader
Step 2: Defining the Neural Network
class SimpleNN(nn.Module):
def __init__(self):
super(SimpleNN, self).__init__()
self.fc1 = nn.Linear(784, 128)
self.fc2 = nn.Linear(128, 64)
self.fc3 = nn.Linear(64, 10)
def forward(self, x):
x = x.view(-1, 784)
x = F.relu(self.fc1(x))
x = F.relu(self.fc2(x))
x = self.fc3(x)
return F.log_softmax(x, dim=1)
Step 3: Loading the Dataset
transform = transforms.Compose([transforms.ToTensor(), transforms.Normalize((0.5,), (0.5,))])
trainset = datasets.MNIST(root='./data', train=True, download=True, transform=transform)
trainloader = DataLoader(trainset, batch_size=64, shuffle=True)
Step 4: Training the Model
model = SimpleNN()
criterion = nn.CrossEntropyLoss()
optimizer = optim.SGD(model.parameters(), lr=0.01)
for epoch in range(10):
running_loss = 0
for images, labels in trainloader:
optimizer.zero_grad()
output = model(images)
loss = criterion(output, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
print(f"Epoch {epoch + 1} - Training loss: {running_loss / len(trainloader)}")
Step 5: Evaluating the Model
correct = 0
total = 0
with torch.no_grad():
for images, labels in trainloader:
outputs = model(images)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
print(f'Accuracy of the model on the training images: {100 * correct / total:.2f}%')
This example illustrates how PyTorch can be used to create and train a simple neural network in pytorch. The flexibility of PyTorch allows developers to easily experiment with new ideas and models, making it an invaluable tool for deep learning.
Combining PyTorch and Scikit-Learn
One of the most powerful aspects of using PyTorch and Scikit-Learn together is leveraging the strengths of both libraries. For instance, you can use Scikit-Learn for preprocessing and feature extraction, and then pass the processed data to a PyTorch model for training.
from sklearn.preprocessing import StandardScaler
from sklearn.datasets import load_iris
import torch
# Load and preprocess the data
data = load_iris()
X, y = data.data, data.target
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
# Convert to PyTorch tensors
X_tensor = torch.tensor(X_scaled, dtype=torch.float32)
y_tensor = torch.tensor(y, dtype=torch.int64)
Advanced Machine Learning with Python
For more advanced use cases, PyTorch and Scikit-Learn offer tools for hyperparameter tuning, model validation, and deployment. Libraries like Optuna can be used for hyperparameter optimization, while ONNX provides a pathway for exporting PyTorch models to other frameworks or hardware. Once you have a solid understanding of the basics, you can explore more advanced topics such as:
- Transfer Learning: Reusing a pre-trained model on a new problem.
- Hyperparameter Tuning: Optimizing model parameters to improve performance.
- Ensemble Methods: Combining multiple models to improve accuracy.
- Reinforcement Learning: Training models based on rewards and penalties.
- Generative Adversarial Networks (GANs): Generating new data similar to existing data.
Practical Applications of Machine Learning and Deep Learning
The applications of machine learning and deep learning are vast and varied. Here are some examples:
- Natural Language Processing (NLP): Developing models that can understand and generate human language.
- Computer Vision: Building models that can interpret and make decisions based on visual data.
- Predictive Analytics: Using historical data to make predictions about future outcomes.
- Autonomous Systems: Creating systems that can perform tasks without human intervention, such as self-driving cars.
Conclusion
Mastering machine learning and deep learning with PyTorch and Scikit-Learn opens up a world of opportunities for developing advanced models that can tackle complex problems. By understanding the strengths of each library and how to leverage them effectively, you can build robust, scalable applications that meet the demands of various industries.
Download PDF: Mastering Machine Learning with PyTorch and Scikit-Learn