In the ever-evolving field of software development, mastering data structures and algorithms is essential for anyone looking to excel in programming, particularly in Python. In this comprehensive guide, we will dive deep into the core concepts of data structures and algorithms in Python, showcasing practical implementations, while also discussing advanced topics for those aiming to improve their understanding and maximize performance in real-world applications.
Why Data Structures and Algorithms in Python Matter
When you write code, it’s not just about getting it to work but about how efficiently it can handle the tasks. For example, if you’re working on big data, the difference between a good and bad data structure or algorithm can result in minutes versus milliseconds of execution time. Data structures define the way data is organized in memory, and algorithms determine how operations such as searching, sorting, and modification are performed. Both are critical in designing high-performance systems, especially as the size of the data grows.
Key Data Structures in Python
1. Arrays (Lists)
In Python, the most commonly used data structure is the list, which is similar to an array in other languages. Lists are dynamic and can grow or shrink in size, making them ideal for many use cases. They allow for fast indexing but have limitations when it comes to insertion and deletion of elements in the middle of the list, which can be costly in terms of performance.
# Example of List in Python
my_list = [1, 2, 3, 4, 5]
my_list.append(6) # O(1) operation
Use Case:
Lists are excellent for storing and accessing a small number of items but may not be optimal for operations involving large datasets where insertion and deletion are frequent.
2. Stacks
A stack is a linear data structure that follows the LIFO (Last In First Out) principle. You can think of it like a stack of plates where you add and remove plates from the top. In Python, stacks are usually implemented using lists with the append() and pop() methods.
stack = []
stack.append(1)
stack.append(2)
stack.pop() # Removes 2 (the last inserted element)
Use Case:
Stacks are ideal for recursive algorithms, undo operations, or parsing expressions.
3. Queues
A queue follows the FIFO (First In First Out) principle. Python provides a deque class in the collections module for an efficient queue implementation, as using lists could lead to inefficiencies for large datasets.
from collections import deque
queue = deque()
queue.append(1)
queue.append(2)
queue.popleft() # Removes 1 (the first inserted element)
Use Case:
Queues are widely used in scenarios like breadth-first search (BFS) algorithms, task scheduling, and order management systems.
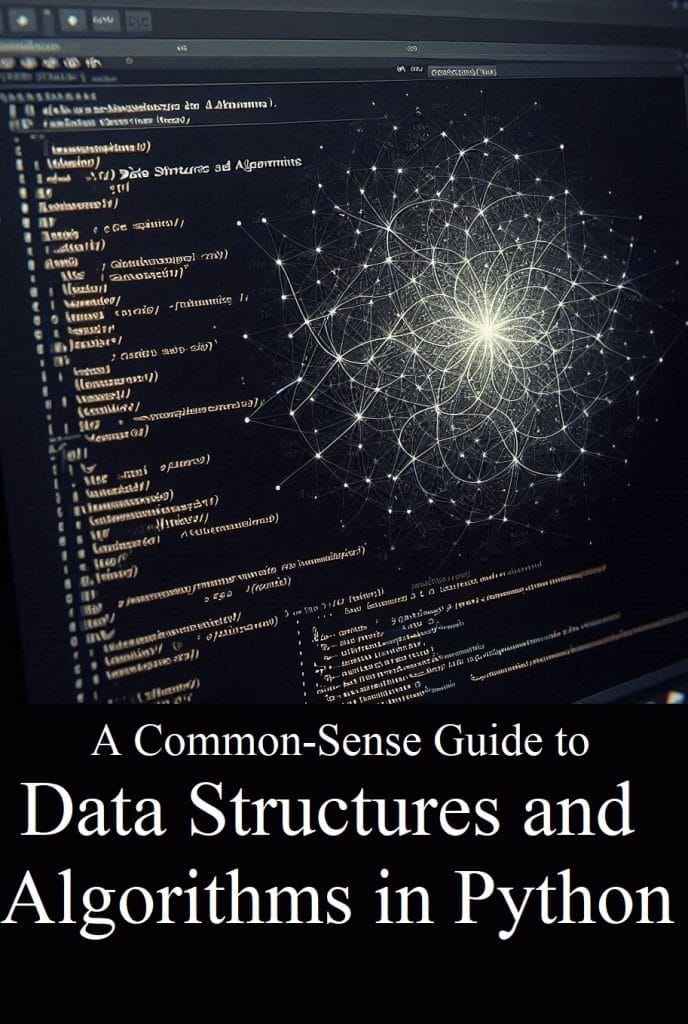
4. Linked Lists
A linked list is a linear data structure where each element is a node containing a reference (pointer) to the next node. It allows for efficient insertion and deletion compared to arrays but requires extra memory for storing pointers.
class Node:
def __init__(self, value=None):
self.value = value
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def insert(self, value):
new_node = Node(value)
new_node.next = self.head
self.head = new_node
Use Case:
Linked lists are excellent when frequent insertion and deletion operations are needed, such as in dynamic memory allocation, task scheduling, or undo features in software applications.
5. Hash Tables (Dictionaries)
A hash table is a collection of key-value pairs where each key is mapped to a value. In Python, this is implemented using the dict data type. Hash tables offer average O(1) time complexity for both insertion and search operations, making them one of the most efficient data structures.
hash_map = {}
hash_map["apple"] = 1
print(hash_map["apple"]) # O(1) access time
Use Case:
Hash tables are ideal for situations requiring fast lookup times, such as database indexing or implementing caches.
6. Trees (Binary Trees and Binary Search Trees)
A binary tree is a hierarchical data structure where each node has at most two children. A binary search tree (BST) is a binary tree where the left child is smaller than the parent, and the right child is greater, ensuring efficient searching, insertion, and deletion.
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
# Insert and search methods are omitted for brevity
Use Case:
Binary search trees are widely used in searching and sorting algorithms. They are also used in databases and file systems to maintain order and allow efficient access.
Essential Algorithms in Python
1. Python Sorting Algorithms
Sorting algorithms arrange data in a specific order, typically ascending or descending. Some of the most common sorting algorithms are:
- Bubble Sort: A simple comparison-based algorithm but inefficient for large datasets (O(n^2) time complexity).
- QuickSort: A divide-and-conquer algorithm with average O(n log n) time complexity.
- Merge Sort: Another divide-and-conquer algorithm with O(n log n) time complexity but requires extra space for merging.
# Example: QuickSort
def quicksort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quicksort(left) + middle + quicksort(right)
2. Search Algorithms Python
Search algorithms are used to find elements within data structures. The most commonly used search algorithms include:
- Python Linear Search algorithm: Scans the entire list to find the desired element (O(n) time complexity).
- Binary Search algorithm Python: Requires a sorted list and divides the list in half during each step (O(log n) time complexity).
# Example: Binary Search
def binary_search(arr, target):
low, high = 0, len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
3. Dynamic Programming Python
Dynamic programming (DP) is an optimization technique used to solve complex problems by breaking them down into simpler subproblems. The Fibonacci sequence is a classic example, where the nth Fibonacci number is the sum of the two preceding ones.
# Example: Dynamic Programming for Fibonacci
def fib(n):
dp = [0] * (n+1)
dp[1] = 1
for i in range(2, n+1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
4. Graph Algorithms Python
Graphs are used to represent relationships between elements. Depth-First Search (DFS) and Breadth-First Search (BFS) are popular graph traversal algorithms.
# Example: DFS
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
for neighbor in graph[start]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
return visited
Conclusion
Mastering data structures and algorithms in Python is crucial for optimizing code performance, especially when dealing with large datasets and complex problems. From basic structures like lists and stacks to advanced structures like trees and graphs, understanding how to implement and apply them efficiently will significantly improve your coding skills.