In today’s digital age, machine learning (ML) has become one of the most sought-after technologies for businesses, data scientists, and technologists alike. At the core of this revolution is Python, an incredibly versatile programming language that has gained prominence as the go-to tool for machine learning development. Whether you’re a novice looking to understand the basics of Python for ML or a seasoned professional aiming to apply machine learning to real-world scenarios, Python offers the necessary tools and libraries to streamline the entire process.
This article delves into the fundamentals of learning Python for machine learning, explores popular libraries, and presents practical real-world applications that demonstrate how Python can be effectively used to implement machine learning models. By the end, you’ll have a clearer understanding of how learning Python serves as a bridge between theory and practice in the realm of machine learning.
Key Python Libraries for Machine Learning
1. NumPy
NumPy (Numerical Python) is the foundational library for numerical computing in Python. It is primarily used for working with arrays and matrices of data. In machine learning, NumPy helps with tasks such as handling large datasets and performing mathematical computations like matrix multiplication, which are key components in many ML algorithms.
Example Use: Initializing large datasets and performing matrix operations when training neural networks.
2. Pandas
Pandas is a data manipulation and analysis library built on top of NumPy. In machine learning, Pandas is essential for data preprocessing, cleaning, and transformation. It allows for handling structured data like CSV files and Excel spreadsheets easily.
Example Use: Loading datasets, handling missing data, and transforming data into a format suitable for machine learning algorithms.
3. Scikit-learn
Scikit-learn is one of the most popular Python libraries for machine learning. It provides simple and efficient tools for data mining, classification, regression, clustering, and dimensionality reduction. Scikit-learn also offers model evaluation and selection tools, making it essential for most machine learning tasks.
Example Use: Training models for tasks like classification, regression, and clustering and evaluating model performance.
4. TensorFlow and Keras
TensorFlow, developed by Google, is one of the most powerful Python libraries for machine learning, particularly for deep learning. TensorFlow allows developers to build and train complex neural networks for tasks such as image recognition, natural language processing (NLP), and more. Keras, a higher-level API built on TensorFlow, simplifies the construction of neural networks by providing an easier-to-use interface.
Example Use: Building and training neural networks for deep learning tasks like image classification and language translation.
5. Matplotlib and Seaborn
While not strictly machine learning libraries, Matplotlib and Seaborn are essential for visualizing data and understanding trends. They help with data exploration, allowing data scientists to gain insights into the data before training machine learning models.
Example Use: Plotting data distributions, feature relationships, and model performance metrics.
Learning Python for Machine Learning Workflow: From Data to Prediction
The process of applying machine learning using Python typically follows these key steps:
1. Data Collection and Preparation
Before applying any machine learning model, the first step is data collection. You can gather data from multiple sources, such as CSV files, databases, or even web APIs. Once collected, data often needs cleaning and preprocessing, which involves handling missing values, converting categorical variables, and scaling numerical features.
Python Tools Used: Pandas for data manipulation, NumPy for numerical computations.
import pandas as pd
# Loading a dataset
data = pd.read_csv('dataset.csv')
# Handling missing values
data.fillna(method='ffill', inplace=True)
# Data scaling
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
data_scaled = scaler.fit_transform(data)
2. Model Selection and Training
Once the data is ready, you need to select a machine learning model. Depending on the type of problem—classification, regression, or clustering—you can use different algorithms. Scikit-learn provides a variety of pre-built models like logistic regression, decision trees, support vector machines, and random forests.
Python Tools Used: Scikit-learn for model selection and training.
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
# Splitting data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(data_scaled, labels, test_size=0.2)
# Training a RandomForest classifier
model = RandomForestClassifier()
model.fit(X_train, y_train)
3. Model Evaluation in Machine Learning
After training the model, it’s crucial to evaluate its performance using metrics like accuracy, precision, recall, and F1 score. Scikit-learn provides several evaluation tools to measure the effectiveness of your model on test data.
Python Tools Used: Scikit-learn for model evaluation.
from sklearn.metrics import accuracy_score, confusion_matrix
# Making predictions
y_pred = model.predict(X_test)
# Evaluating the model
accuracy = accuracy_score(y_test, y_pred)
print(f"Accuracy: {accuracy}")
# Displaying a confusion matrix
print(confusion_matrix(y_test, y_pred))
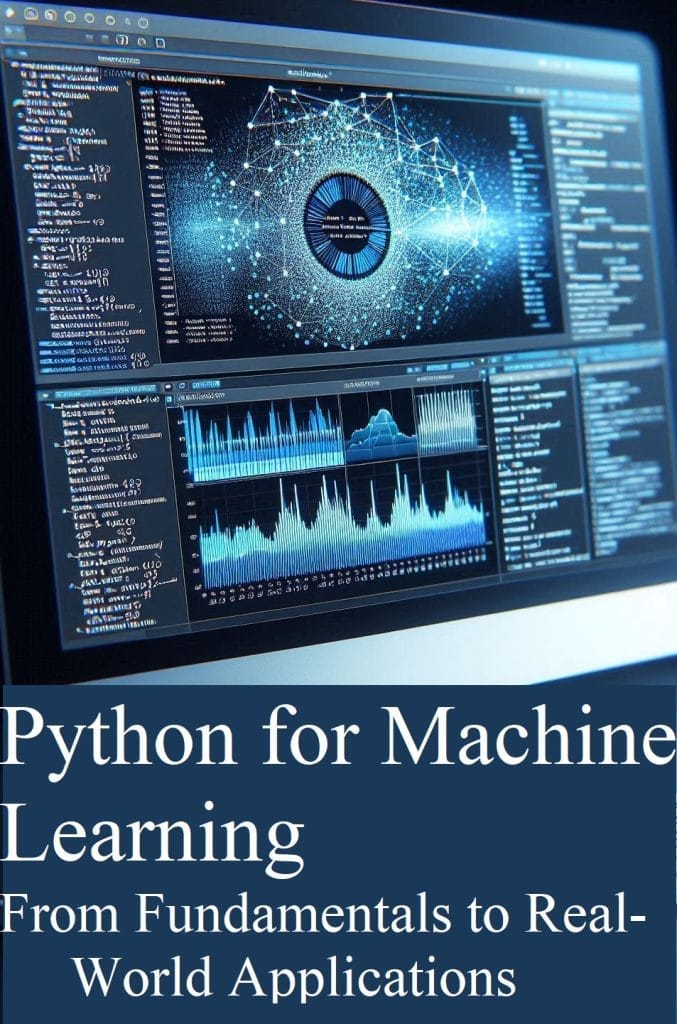
4. Model Tuning and Optimization
Once a model is trained and evaluated, you may want to fine-tune it by adjusting hyperparameters. Techniques like cross-validation and grid search can help in selecting the optimal parameters for your model.
Hyperparameter Tuning in Machine Learning: Scikit-learn for hyperparameter tuning, GridSearchCV for optimization.
from sklearn.model_selection import GridSearchCV
# Defining parameter grid for optimization
param_grid = {
'n_estimators': [100, 200, 300],
'max_depth': [10, 20, 30],
}
# Optimizing with GridSearchCV
grid_search = GridSearchCV(estimator=model, param_grid=param_grid, cv=5)
grid_search.fit(X_train, y_train)
# Best parameters
print(f"Best parameters: {grid_search.best_params_}")
5. Machine Learning Real World Examples
Python’s machine learning capabilities go beyond academia and research; they are widely applied in real-world scenarios across various industries.
- Healthcare: Python-based machine learning models are used in predicting disease outbreaks, diagnosing medical conditions, and personalizing treatment plans.
- Finance: In the finance industry, Python is used to develop algorithms for predicting stock prices, managing risks, and detecting fraudulent activities.
- Retail: Machine learning models are used to analyze customer behavior, optimize inventory management, and personalize product recommendations.
- Autonomous Vehicles: Python is integral in building models for self-driving cars, where deep learning algorithms are trained to make real-time decisions based on visual data.
- Natural Language Processing (NLP): Python’s libraries like NLTK and SpaCy are used for processing and analyzing large amounts of textual data, leading to the development of chatbots, sentiment analysis tools, and more.
Advanced Techniques in Python for Machine Learning
While the fundamentals are crucial, advanced techniques like deep learning, reinforcement learning, and ensemble methods can take your machine learning models to the next level.
- Deep Learning: Neural networks, particularly deep neural networks, are used for complex tasks like image recognition and speech processing. Libraries like TensorFlow and PyTorch are essential for building and training deep learning models.
- Reinforcement Learning: This involves training agents to make a sequence of decisions by rewarding or penalizing them based on their actions. Python’s OpenAI Gym provides a platform for experimenting with reinforcement learning algorithms.
- Ensemble Methods: Techniques like bagging and boosting combine multiple models to improve predictive performance. Python’s XGBoost library is popular for implementing boosting algorithms in competitions and real-world problems.
Conclusion
Python has established itself as a premier language for machine learning, offering a wide array of tools and libraries that cater to every aspect of the machine learning process, from data collection to model deployment. By mastering Python and its associated libraries like NumPy, Pandas, Scikit-learn, and TensorFlow, you can build sophisticated machine learning models and apply them to solve real-world problems across various industries.
As you delve deeper into Python for machine learning, you will find that the language’s flexibility and ease of use make it an indispensable tool for both beginners and seasoned professionals. The integration of Python with machine learning not only enhances efficiency but also drives innovation in data-driven industries.