Machine learning has revolutionized numerous fields by enabling computers to learn from data and make predictions or decisions without explicit programming. Two of the foundational libraries in Python for machine learning and scientific computing are NumPy and SciPy. Machine learning with NumPy and SciPy offer essential tools and functions for data manipulation, mathematical computation, and algorithm development. In this comprehensive guide, we’ll explore how to leverage NumPy and SciPy for machine learning, providing practical examples and insights to enhance your data science toolkit.
Understanding NumPy and SciPy
What is NumPy?
NumPy (Numerical Python) is a fundamental library for scientific computing in Python. It provides support for arrays, matrices, and a wide array of mathematical functions. NumPy’s core functionality is its ndarray object, a multidimensional array that allows for efficient storage and manipulation of numerical data. Key features include:
- N-Dimensional Arrays: Efficient handling of large datasets.
- Mathematical Functions: Comprehensive set of operations for arithmetic, statistical, and linear algebra.
- Broadcasting: Ability to perform operations on arrays of different shapes and sizes.
What is SciPy?
SciPy builds on NumPy by adding additional functionality for scientific and technical computing. It includes modules for optimization, integration, interpolation, eigenvalue problems, and other advanced mathematical operations. Key features include:
- Optimization: Algorithms for minimizing or maximizing functions.
- Integration: Numerical integration methods for evaluating integrals.
- Interpolation: Techniques for estimating values between data points.
- Signal Processing: Tools for analyzing and manipulating signals.
Together, NumPy and SciPy provide a robust environment for developing machine learning algorithms and performing complex computations.
Applying NumPy and SciPy to Machine Learning
Machine learning tasks often involve handling large datasets, performing mathematical computations, and developing algorithms. Here’s how NumPy and SciPy can be used effectively in these areas:
Data Preparation and Manipulation
Before applying machine learning algorithms, it is crucial to preprocess and manipulate data. NumPy offers powerful tools for these tasks:
Array Operations: Use NumPy arrays to efficiently perform operations on large datasets. For example, you can use numpy.array() to create arrays and numpy.reshape() to change their shape.
import numpy as np
# Create an array
data = np.array([[1, 2, 3], [4, 5, 6]])
# Reshape the array
reshaped_data = data.reshape(3, 2)
Data Normalization: Scaling features to a uniform range is essential for many machine learning algorithms. NumPy provides functions like numpy.mean() and numpy.std() for normalization.
from sklearn.preprocessing import StandardScaler
# Data normalization
scaler = StandardScaler()
normalized_data = scaler.fit_transform(data)
Statistical Analysis
Statistical analysis is a critical aspect of understanding data distributions and relationships. NumPy and SciPy offer various statistical functions:
Descriptive Statistics: Calculate measures such as mean, median, variance, and standard deviation.
# Mean and standard deviation
mean = np.mean(data)
std_dev = np.std(data)
Probability Distributions: SciPy provides a wide range of probability distributions and statistical tests. For example, scipy.stats.norm allows you to work with the normal distribution.
from scipy import stats
# Probability density function
pdf = stats.norm.pdf(data)
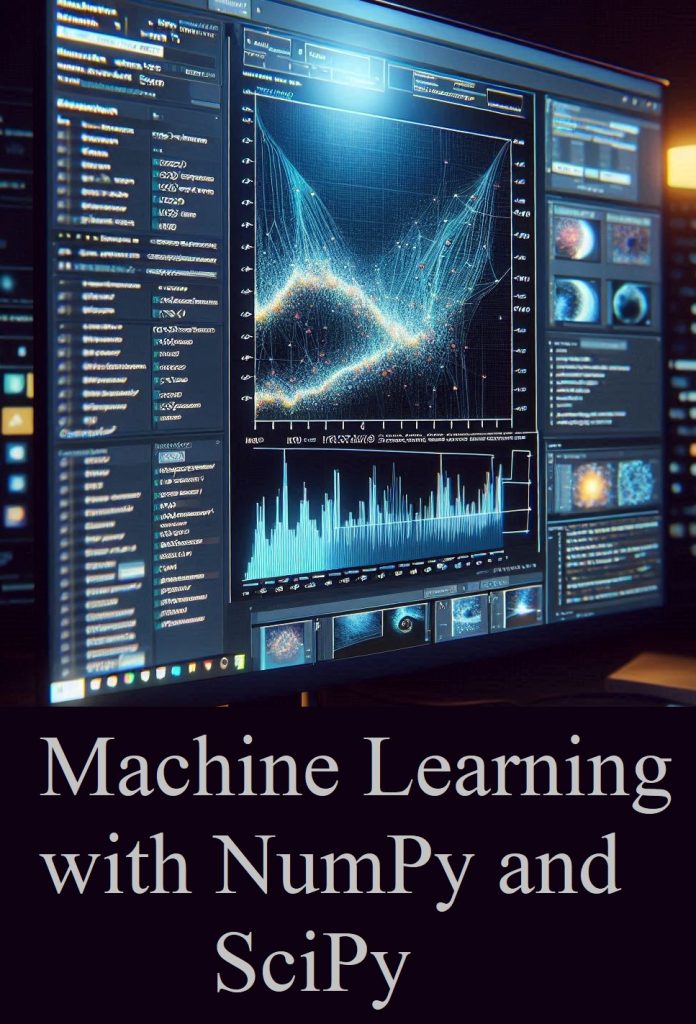
Linear Algebra in Python
Many machine learning algorithms rely on linear algebra operations such as matrix multiplication and eigenvalue decomposition:
Matrix Multiplication: NumPy’s numpy.dot() function performs matrix multiplication efficiently.
# Matrix multiplication
matrix_a = np.array([[1, 2], [3, 4]])
matrix_b = np.array([[5, 6], [7, 8]])
result = np.dot(matrix_a, matrix_b)
Eigenvalue Decomposition: SciPy’s scipy.linalg.eig() function computes eigenvalues and eigenvectors of a matrix.
from scipy.linalg import eig
# Eigenvalue decomposition
values, vectors = eig(matrix_a)
Optimization
Optimization techniques are used to minimize or maximize objective functions. SciPy offers a variety of optimization algorithms:
Minimizing Functions: Use scipy.optimize.minimize() to find the minimum of a function.
from scipy.optimize import minimize
# Define a simple quadratic function
def objective_function(x):
return x**2 + 5*x + 6
# Find the minimum
result = minimize(objective_function, x0=0)
Curve Fitting: SciPy’s scipy.optimize.curve_fit() can fit a curve to data, which is useful for regression tasks.
from scipy.optimize import curve_fit
# Define a model function
def model(x, a, b):
return a * x + b
# Fit the model to data
params, covariance = curve_fit(model, x_data, y_data)
SciPy for Machine Learning Algorithms
NumPy and SciPy provide foundational support for implementing various machine learning algorithms:
Gradient Descent in Machine Learning: Implement gradient descent for optimization in machine learning models.
# Gradient descent implementation
def gradient_descent(x, y, learning_rate, iterations):
for _ in range(iterations):
gradients = compute_gradients(x, y)
x -= learning_rate * gradients
return x
K Means Clustering Python: Perform clustering tasks such as k-means using NumPy and SciPy functions.
from scipy.cluster.vq import kmeans, vq
# K-means clustering
centroids, _ = kmeans(data, num_clusters)
clusters, _ = vq(data, centroids)
Data Visualization
Effective visualization of data and results is crucial in machine learning. While Matplotlib and Seaborn are commonly used libraries, NumPy and SciPy can assist in preparing data for visualization:
Preparing Data for Visualization: Use NumPy to manipulate and preprocess data before visualizing it with Matplotlib.
import matplotlib.pyplot as plt
# Prepare data
x = np.linspace(0, 10, 100)
y = np.sin(x)
# Plot the data
plt.plot(x, y)
plt.xlabel('X')
plt.ylabel('Y')
plt.title('Sine Wave')
plt.show()
Practical Examples and Use Cases
Example 1: Predicting Housing Prices
Consider a scenario where you want to predict housing prices based on features such as square footage, number of bedrooms, and location. You can use NumPy for data manipulation and SciPy for optimization:
Data Preparation: Load and preprocess the data using NumPy.
import numpy as np
# Load data
data = np.loadtxt('housing_data.csv', delimiter=',')
X = data[:, :-1]
y = data[:, -1]
Linear Regression: Use SciPy to perform linear regression and predict prices.
from scipy.linalg import lstsq
# Perform linear regression
coefficients, residuals, rank, s = lstsq(X, y)
Prediction: Use the model to predict new housing prices.
# Predict prices
predictions = np.dot(X_new, coefficients)
Example 2: Clustering Customer Data
Imagine you have customer data and want to cluster customers based on their purchasing behavior:
Load and Prepare Data: Use NumPy to handle the dataset.
import numpy as np
# Load customer data
data = np.loadtxt('customer_data.csv', delimiter=',')
Apply K-Means Clustering: Use SciPy’s k-means clustering algorithm to group customers.
from scipy.cluster.vq import kmeans, vq
# Cluster the data
centroids, _ = kmeans(data, num_clusters)
clusters, _ = vq(data, centroids)
Analyze Clusters: Examine the resulting clusters to understand customer segments.
# Analyze clusters
for cluster_id in range(num_clusters):
print(f'Cluster {cluster_id}: {np.mean(data[clusters == cluster_id], axis=0)}')
Conclusion
NumPy and SciPy are invaluable tools for machine learning and data analysis, providing the foundational support needed for efficient data manipulation, mathematical computation, and algorithm development. By leveraging these libraries, data scientists and machine learning practitioners can build robust models, perform complex analyses, and derive meaningful insights from data.
Understanding machine learning with numpy and SciPy can significantly enhance your ability to tackle diverse problems and develop effective solutions. As you continue to explore these libraries, keep experimenting with different algorithms, techniques, and applications to stay at the forefront of machine learning advancements.